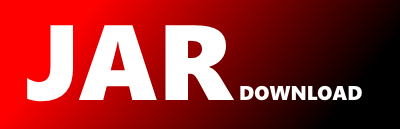
io.tiledb.cloud.rest_api.v2.api.FilesApi Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of tiledb-cloud-java Show documentation
Show all versions of tiledb-cloud-java Show documentation
The Java client for the TileDB Cloud Service
/*
* Tiledb Storage Platform API
* TileDB Storage Platform REST API
*
* The version of the OpenAPI document: 1.4.0
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package io.tiledb.cloud.rest_api.v2.api;
import io.tiledb.cloud.rest_api.v2.model.FileUploaded;
import io.tiledb.cloud.rest_api.v2.ApiCallback;
import io.tiledb.cloud.rest_api.v2.ApiClient;
import io.tiledb.cloud.rest_api.v2.ApiException;
import io.tiledb.cloud.rest_api.v2.ApiResponse;
import io.tiledb.cloud.rest_api.v2.Configuration;
import io.tiledb.cloud.rest_api.v2.Pair;
import com.google.gson.reflect.TypeToken;
import java.io.File;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class FilesApi {
private ApiClient localVarApiClient;
private int localHostIndex;
private String localCustomBaseUrl;
public FilesApi() {
this(Configuration.getDefaultApiClient());
}
public FilesApi(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public ApiClient getApiClient() {
return localVarApiClient;
}
public void setApiClient(ApiClient apiClient) {
this.localVarApiClient = apiClient;
}
public int getHostIndex() {
return localHostIndex;
}
public void setHostIndex(int hostIndex) {
this.localHostIndex = hostIndex;
}
public String getCustomBaseUrl() {
return localCustomBaseUrl;
}
public void setCustomBaseUrl(String customBaseUrl) {
this.localCustomBaseUrl = customBaseUrl;
}
/**
* Build call for handleUploadFile
* @param namespace The namespace of the file (required)
* @param array name/uri of array that is url-encoded (required)
* @param contentType Content Type of input (required)
* @param filesize size of the file to upload in bytes (required)
* @param _file file to upload (required)
* @param X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME Optional registered access credentials to use for creation (optional)
* @param name name of the TileDB array to create, if missing {array} is used (optional)
* @param filename original file name (optional)
* @param mimetype Mime type of the uploaded file. Autogenerated clients do not always support changing the content type param. Server will always use mimetype query param to set mimetype for file, if it is not set Content-Type will be used (optional)
* @param _callback Callback for upload/download progress
* @return Call to execute
* @throws ApiException If fail to serialize the request body object
* @http.response.details
Status Code Description Response Headers
201 File uploaded -
502 Bad Gateway -
0 error response -
*/
public okhttp3.Call handleUploadFileCall(String namespace, String array, String contentType, Integer filesize, File _file, String X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, String name, String filename, String mimetype, final ApiCallback _callback) throws ApiException {
String basePath = null;
// Operation Servers
String[] localBasePaths = new String[] { };
// Determine Base Path to Use
if (localCustomBaseUrl != null){
basePath = localCustomBaseUrl;
} else if ( localBasePaths.length > 0 ) {
basePath = localBasePaths[localHostIndex];
} else {
basePath = null;
}
Object localVarPostBody = _file;
// create path and map variables
String localVarPath = "/files/{namespace}/{array}/upload"
.replaceAll("\\{" + "namespace" + "\\}", localVarApiClient.escapeString(namespace.toString()))
.replaceAll("\\{" + "array" + "\\}", localVarApiClient.escapeString(array.toString()));
List localVarQueryParams = new ArrayList();
List localVarCollectionQueryParams = new ArrayList();
Map localVarHeaderParams = new HashMap();
Map localVarCookieParams = new HashMap();
Map localVarFormParams = new HashMap();
if (name != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("name", name));
}
if (filename != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("filename", filename));
}
if (filesize != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("filesize", filesize));
}
if (mimetype != null) {
localVarQueryParams.addAll(localVarApiClient.parameterToPair("mimetype", mimetype));
}
if (X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME != null) {
localVarHeaderParams.put("X-TILEDB-CLOUD-ACCESS-CREDENTIALS-NAME", localVarApiClient.parameterToString(X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME));
}
if (contentType != null) {
localVarHeaderParams.put("Content-Type", localVarApiClient.parameterToString(contentType));
}
final String[] localVarAccepts = {
"application/json"
};
final String localVarAccept = localVarApiClient.selectHeaderAccept(localVarAccepts);
if (localVarAccept != null) {
localVarHeaderParams.put("Accept", localVarAccept);
}
final String[] localVarContentTypes = {
"application/octet-stream"
};
final String localVarContentType = localVarApiClient.selectHeaderContentType(localVarContentTypes);
if (localVarContentType != null) {
localVarHeaderParams.put("Content-Type", localVarContentType);
}
String[] localVarAuthNames = new String[] { "ApiKeyAuth", "BasicAuth" };
return localVarApiClient.buildCall(basePath, localVarPath, "POST", localVarQueryParams, localVarCollectionQueryParams, localVarPostBody, localVarHeaderParams, localVarCookieParams, localVarFormParams, localVarAuthNames, _callback);
}
@SuppressWarnings("rawtypes")
private okhttp3.Call handleUploadFileValidateBeforeCall(String namespace, String array, String contentType, Integer filesize, File _file, String X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, String name, String filename, String mimetype, final ApiCallback _callback) throws ApiException {
// verify the required parameter 'namespace' is set
if (namespace == null) {
throw new ApiException("Missing the required parameter 'namespace' when calling handleUploadFile(Async)");
}
// verify the required parameter 'array' is set
if (array == null) {
throw new ApiException("Missing the required parameter 'array' when calling handleUploadFile(Async)");
}
// verify the required parameter 'contentType' is set
if (contentType == null) {
throw new ApiException("Missing the required parameter 'contentType' when calling handleUploadFile(Async)");
}
// verify the required parameter 'filesize' is set
if (filesize == null) {
throw new ApiException("Missing the required parameter 'filesize' when calling handleUploadFile(Async)");
}
// verify the required parameter '_file' is set
if (_file == null) {
throw new ApiException("Missing the required parameter '_file' when calling handleUploadFile(Async)");
}
okhttp3.Call localVarCall = handleUploadFileCall(namespace, array, contentType, filesize, _file, X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, name, filename, mimetype, _callback);
return localVarCall;
}
/**
*
* Upload a file at the specified location and wrap it in TileDB Array
* @param namespace The namespace of the file (required)
* @param array name/uri of array that is url-encoded (required)
* @param contentType Content Type of input (required)
* @param filesize size of the file to upload in bytes (required)
* @param _file file to upload (required)
* @param X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME Optional registered access credentials to use for creation (optional)
* @param name name of the TileDB array to create, if missing {array} is used (optional)
* @param filename original file name (optional)
* @param mimetype Mime type of the uploaded file. Autogenerated clients do not always support changing the content type param. Server will always use mimetype query param to set mimetype for file, if it is not set Content-Type will be used (optional)
* @return FileUploaded
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @http.response.details
Status Code Description Response Headers
201 File uploaded -
502 Bad Gateway -
0 error response -
*/
public FileUploaded handleUploadFile(String namespace, String array, String contentType, Integer filesize, File _file, String X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, String name, String filename, String mimetype) throws ApiException {
ApiResponse localVarResp = handleUploadFileWithHttpInfo(namespace, array, contentType, filesize, _file, X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, name, filename, mimetype);
return localVarResp.getData();
}
/**
*
* Upload a file at the specified location and wrap it in TileDB Array
* @param namespace The namespace of the file (required)
* @param array name/uri of array that is url-encoded (required)
* @param contentType Content Type of input (required)
* @param filesize size of the file to upload in bytes (required)
* @param _file file to upload (required)
* @param X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME Optional registered access credentials to use for creation (optional)
* @param name name of the TileDB array to create, if missing {array} is used (optional)
* @param filename original file name (optional)
* @param mimetype Mime type of the uploaded file. Autogenerated clients do not always support changing the content type param. Server will always use mimetype query param to set mimetype for file, if it is not set Content-Type will be used (optional)
* @return ApiResponse<FileUploaded>
* @throws ApiException If fail to call the API, e.g. server error or cannot deserialize the response body
* @http.response.details
Status Code Description Response Headers
201 File uploaded -
502 Bad Gateway -
0 error response -
*/
public ApiResponse handleUploadFileWithHttpInfo(String namespace, String array, String contentType, Integer filesize, File _file, String X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, String name, String filename, String mimetype) throws ApiException {
okhttp3.Call localVarCall = handleUploadFileValidateBeforeCall(namespace, array, contentType, filesize, _file, X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, name, filename, mimetype, null);
Type localVarReturnType = new TypeToken(){}.getType();
return localVarApiClient.execute(localVarCall, localVarReturnType);
}
/**
* (asynchronously)
* Upload a file at the specified location and wrap it in TileDB Array
* @param namespace The namespace of the file (required)
* @param array name/uri of array that is url-encoded (required)
* @param contentType Content Type of input (required)
* @param filesize size of the file to upload in bytes (required)
* @param _file file to upload (required)
* @param X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME Optional registered access credentials to use for creation (optional)
* @param name name of the TileDB array to create, if missing {array} is used (optional)
* @param filename original file name (optional)
* @param mimetype Mime type of the uploaded file. Autogenerated clients do not always support changing the content type param. Server will always use mimetype query param to set mimetype for file, if it is not set Content-Type will be used (optional)
* @param _callback The callback to be executed when the API call finishes
* @return The request call
* @throws ApiException If fail to process the API call, e.g. serializing the request body object
* @http.response.details
Status Code Description Response Headers
201 File uploaded -
502 Bad Gateway -
0 error response -
*/
public okhttp3.Call handleUploadFileAsync(String namespace, String array, String contentType, Integer filesize, File _file, String X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, String name, String filename, String mimetype, final ApiCallback _callback) throws ApiException {
okhttp3.Call localVarCall = handleUploadFileValidateBeforeCall(namespace, array, contentType, filesize, _file, X_TILEDB_CLOUD_ACCESS_CREDENTIALS_NAME, name, filename, mimetype, _callback);
Type localVarReturnType = new TypeToken(){}.getType();
localVarApiClient.executeAsync(localVarCall, localVarReturnType, _callback);
return localVarCall;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy