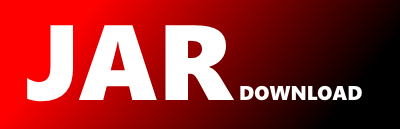
cache.KSerializerCache.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of avro-kotlin-serialization Show documentation
Show all versions of avro-kotlin-serialization Show documentation
Using kotlinx-serialization to encode/decode kotlin to avro.
The newest version!
package io.toolisticon.kotlin.avro.serialization.cache
import io.toolisticon.kotlin.avro.serialization.isSerializable
import kotlinx.serialization.KSerializer
import kotlinx.serialization.modules.SerializersModule
import kotlinx.serialization.serializer
import mu.KLogging
import org.apache.avro.util.WeakIdentityHashMap
import kotlin.reflect.KClass
import kotlin.reflect.KType
import kotlin.reflect.full.createType
/**
* Provides [KSerializer]s for given [KClass] using kotlin-reflect and avro4k.
* Values are cached for fast access of recurrent requests.
*
* Cache is in-mem only and not limited/evicted, as we will have one class/serializer pair for
* each avro-message type, and it seems reasonable to expect that there won't be more than a few dozens.
*
* TODO: avro4k internally keeps st similar, it would be nice if their cache would be accessible, then we could use it here (or omit this class completely).
*/
class KSerializerCache (
private val serializersModule: SerializersModule
) : AvroCache.SerializerByClassCache {
companion object : KLogging()
private val store: WeakIdentityHashMap, KSerializer<*>> = WeakIdentityHashMap()
@Suppress("UNCHECKED_CAST")
override operator fun get(klass: KClass): KSerializer = store.getOrPut(klass) {
require(klass.isSerializable()) { "$klass is not serializable with kotlinx-serialization." }
// TODO: if we use SpecificRecords, we could derive the schema from the class directly
logger.trace { "add kserializer for $klass." }
// TODO: createType takes a lot of optional args. We probably won't need them but at least we should check them.
val type: KType = klass.createType()
serializersModule.serializer(type)
} as KSerializer
fun keys(): Set> = store.keys.sortedBy { it.simpleName }.toSet()
override fun toString() = "KSerializerCache(keys=${keys()})"
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy