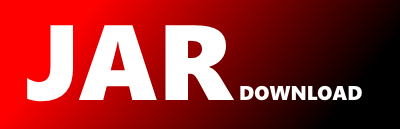
io.trino.filesystem.azure.AzureFileSystemFactory Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.trino.filesystem.azure;
import com.azure.core.http.HttpClient;
import com.azure.core.http.okhttp.OkHttpAsyncClientProvider;
import com.azure.core.tracing.opentelemetry.OpenTelemetryTracingOptions;
import com.azure.core.util.HttpClientOptions;
import com.azure.core.util.TracingOptions;
import com.google.inject.Inject;
import io.airlift.units.DataSize;
import io.opentelemetry.api.OpenTelemetry;
import io.trino.filesystem.TrinoFileSystem;
import io.trino.filesystem.TrinoFileSystemFactory;
import io.trino.spi.security.ConnectorIdentity;
import static com.google.common.base.Preconditions.checkArgument;
import static java.util.Objects.requireNonNull;
public class AzureFileSystemFactory
implements TrinoFileSystemFactory
{
private final AzureAuth auth;
private final DataSize readBlockSize;
private final DataSize writeBlockSize;
private final int maxWriteConcurrency;
private final DataSize maxSingleUploadSize;
private final TracingOptions tracingOptions;
private final HttpClient httpClient;
@Inject
public AzureFileSystemFactory(OpenTelemetry openTelemetry, AzureAuth azureAuth, AzureFileSystemConfig config)
{
this(openTelemetry,
azureAuth,
config.getReadBlockSize(),
config.getWriteBlockSize(),
config.getMaxWriteConcurrency(),
config.getMaxSingleUploadSize());
}
public AzureFileSystemFactory(
OpenTelemetry openTelemetry,
AzureAuth azureAuth,
DataSize readBlockSize,
DataSize writeBlockSize,
int maxWriteConcurrency,
DataSize maxSingleUploadSize)
{
this.auth = requireNonNull(azureAuth, "azureAuth is null");
this.readBlockSize = requireNonNull(readBlockSize, "readBlockSize is null");
this.writeBlockSize = requireNonNull(writeBlockSize, "writeBlockSize is null");
checkArgument(maxWriteConcurrency >= 0, "maxWriteConcurrency is negative");
this.maxWriteConcurrency = maxWriteConcurrency;
this.maxSingleUploadSize = requireNonNull(maxSingleUploadSize, "maxSingleUploadSize is null");
this.tracingOptions = new OpenTelemetryTracingOptions().setOpenTelemetry(openTelemetry);
this.httpClient = HttpClient.createDefault((HttpClientOptions) new HttpClientOptions()
.setHttpClientProvider(OkHttpAsyncClientProvider.class)
.setTracingOptions(tracingOptions));
}
@Override
public TrinoFileSystem create(ConnectorIdentity identity)
{
return new AzureFileSystem(httpClient, tracingOptions, auth, readBlockSize, writeBlockSize, maxWriteConcurrency, maxSingleUploadSize);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy