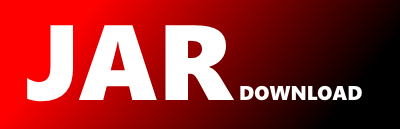
io.trino.plugin.hive.metastore.glue.converter.GlueToTrinoConverter Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.trino.plugin.hive.metastore.glue.converter;
import com.amazonaws.services.glue.model.SerDeInfo;
import com.amazonaws.services.glue.model.StorageDescriptor;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import io.trino.plugin.hive.HiveBucketProperty;
import io.trino.plugin.hive.HiveStorageFormat;
import io.trino.plugin.hive.HiveType;
import io.trino.plugin.hive.metastore.Column;
import io.trino.plugin.hive.metastore.Database;
import io.trino.plugin.hive.metastore.Partition;
import io.trino.plugin.hive.metastore.SortingColumn;
import io.trino.plugin.hive.metastore.SortingColumn.Order;
import io.trino.plugin.hive.metastore.Storage;
import io.trino.plugin.hive.metastore.StorageFormat;
import io.trino.plugin.hive.metastore.Table;
import io.trino.plugin.hive.util.HiveBucketing;
import io.trino.plugin.hive.util.HiveBucketing.BucketingVersion;
import io.trino.spi.TrinoException;
import io.trino.spi.security.PrincipalType;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Optional;
import java.util.function.Function;
import java.util.function.UnaryOperator;
import static com.google.common.base.MoreObjects.firstNonNull;
import static com.google.common.base.Strings.nullToEmpty;
import static io.trino.plugin.hive.HiveErrorCode.HIVE_INVALID_METADATA;
import static io.trino.plugin.hive.metastore.util.Memoizers.memoizeLast;
import static java.lang.String.format;
import static java.util.Objects.requireNonNull;
import static org.apache.hadoop.hive.metastore.TableType.EXTERNAL_TABLE;
public final class GlueToTrinoConverter
{
private static final String PUBLIC_OWNER = "PUBLIC";
private GlueToTrinoConverter() {}
public static Database convertDatabase(com.amazonaws.services.glue.model.Database glueDb)
{
return Database.builder()
.setDatabaseName(glueDb.getName())
.setLocation(Optional.ofNullable(glueDb.getLocationUri()))
.setComment(Optional.ofNullable(glueDb.getDescription()))
.setParameters(firstNonNull(glueDb.getParameters(), ImmutableMap.of()))
.setOwnerName(PUBLIC_OWNER)
.setOwnerType(PrincipalType.ROLE)
.build();
}
public static Table convertTable(com.amazonaws.services.glue.model.Table glueTable, String dbName)
{
requireNonNull(glueTable.getStorageDescriptor(), "Table StorageDescriptor is null");
Map tableParameters = convertParameters(glueTable.getParameters());
StorageDescriptor sd = glueTable.getStorageDescriptor();
Table.Builder tableBuilder = Table.builder()
.setDatabaseName(dbName)
.setTableName(glueTable.getName())
.setOwner(nullToEmpty(glueTable.getOwner()))
// Athena treats missing table type as EXTERNAL_TABLE.
.setTableType(firstNonNull(glueTable.getTableType(), EXTERNAL_TABLE.name()))
.setDataColumns(convertColumns(sd.getColumns(), sd.getSerdeInfo().getSerializationLibrary()))
.setParameters(tableParameters)
.setViewOriginalText(Optional.ofNullable(glueTable.getViewOriginalText()))
.setViewExpandedText(Optional.ofNullable(glueTable.getViewExpandedText()));
if (glueTable.getPartitionKeys() != null) {
tableBuilder.setPartitionColumns(convertColumns(glueTable.getPartitionKeys(), sd.getSerdeInfo().getSerializationLibrary()));
}
else {
tableBuilder.setPartitionColumns(ImmutableList.of());
}
// No benefit to memoizing here, just reusing the implementation
new StorageConverter().setStorageBuilder(sd, tableBuilder.getStorageBuilder(), tableParameters);
return tableBuilder.build();
}
private static Column convertColumn(com.amazonaws.services.glue.model.Column glueColumn, String serDe)
{
// OpenCSVSerde deserializes columns from csv file into strings, so we set the column type from the metastore
// to string to avoid cast exceptions.
if (HiveStorageFormat.CSV.getSerDe().equals(serDe)) {
//TODO(https://github.com/trinodb/trino/issues/7240) Add tests
return new Column(glueColumn.getName(), HiveType.HIVE_STRING, Optional.ofNullable(glueColumn.getComment()));
}
else {
return new Column(glueColumn.getName(), HiveType.valueOf(glueColumn.getType().toLowerCase(Locale.ENGLISH)), Optional.ofNullable(glueColumn.getComment()));
}
}
private static List convertColumns(List glueColumns, String serDe)
{
return mappedCopy(glueColumns, glueColumn -> convertColumn(glueColumn, serDe));
}
private static Map convertParameters(Map parameters)
{
if (parameters == null || parameters.isEmpty()) {
return ImmutableMap.of();
}
return ImmutableMap.copyOf(parameters);
}
private static Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy