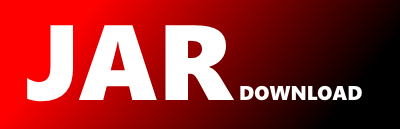
io.trino.spi.block.Block Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.trino.spi.block;
import java.util.OptionalInt;
import java.util.function.ObjLongConsumer;
import static io.trino.spi.block.BlockUtil.checkArrayRange;
import static io.trino.spi.block.DictionaryId.randomDictionaryId;
public sealed interface Block
permits DictionaryBlock, RunLengthEncodedBlock, LazyBlock, ValueBlock
{
/**
* Gets the value at the specified position as a single element block. The method
* must copy the data into a new block.
*
* This method is useful for operators that hold on to a single value without
* holding on to the entire block.
*
* @throws IllegalArgumentException if this position is not valid
*/
ValueBlock getSingleValueBlock(int position);
/**
* Returns the number of positions in this block.
*/
int getPositionCount();
/**
* Returns the size of this block as if it was compacted, ignoring any over-allocations
* and any unloaded nested blocks.
* For example, in dictionary blocks, this only counts each dictionary entry once,
* rather than each time a value is referenced.
*/
long getSizeInBytes();
/**
* Returns the size of {@code block.getRegion(position, length)}.
* The method can be expensive. Do not use it outside an implementation of Block.
*/
long getRegionSizeInBytes(int position, int length);
/**
* Returns the number of bytes (in terms of {@link Block#getSizeInBytes()}) required per position
* that this block contains, assuming that the number of bytes required is a known static quantity
* and not dependent on any particular specific position. This allows for some complex block wrappings
* to potentially avoid having to call {@link Block#getPositionsSizeInBytes(boolean[], int)} which
* would require computing the specific positions selected
*
* @return The size in bytes, per position, if this block type does not require specific position information to compute its size
*/
OptionalInt fixedSizeInBytesPerPosition();
/**
* Returns the size of all positions marked true in the positions array.
* This is equivalent to multiple calls of {@code block.getRegionSizeInBytes(position, length)}
* where you mark all positions for the regions first.
* The 'selectedPositionsCount' variable may be used to skip iterating through
* the positions array in case this is a fixed-width block
*/
long getPositionsSizeInBytes(boolean[] positions, int selectedPositionsCount);
/**
* Returns the retained size of this block in memory, including over-allocations.
* This method is called from the inner most execution loop and must be fast.
*/
long getRetainedSizeInBytes();
/**
* Returns the estimated in memory data size for stats of position.
* Do not use it for other purpose.
*/
long getEstimatedDataSizeForStats(int position);
/**
* {@code consumer} visits each of the internal data container and accepts the size for it.
* This method can be helpful in cases such as memory counting for internal data structure.
* Also, the method should be non-recursive, only visit the elements at the top level,
* and specifically should not call retainedBytesForEachPart on nested blocks
* {@code consumer} should be called at least once with the current block and
* must include the instance size of the current block
*/
void retainedBytesForEachPart(ObjLongConsumer