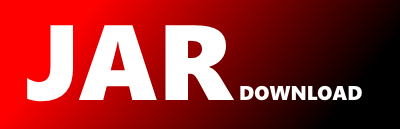
io.ultreia.gc.model.GcArcheoLog Maven / Gradle / Ivy
package io.ultreia.gc.model;
/*-
* #%L
* GC toolkit :: API
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Date;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Optional;
import java.util.concurrent.TimeUnit;
/**
* Created by tchemit on 18/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class GcArcheoLog {
private final String gcName;
private final String cacheUid;
private final GcLog myLog;
private final GcLog previousLog;
private int nbDays;
private int score;
private static final Map SCORES_TABLES = new LinkedHashMap<>();
static {
SCORES_TABLES.put(500, 30);
SCORES_TABLES.put(365, 20);
SCORES_TABLES.put(270, 15);
SCORES_TABLES.put(180, 10);
SCORES_TABLES.put(90, 5);
}
public GcArcheoLog(String gcName, String cacheUid, GcLog myLog, GcLog previousLog) {
this.gcName = gcName;
this.cacheUid = cacheUid;
this.myLog = myLog;
this.previousLog = previousLog;
computeType();
}
public void computeType() {
getPreviousLog().ifPresent(pl -> {
Date myDate = myLog.getDate();
Date previousDate = previousLog.getDate();
nbDays = (int) (TimeUnit.DAYS.convert(myDate.getTime(), TimeUnit.MILLISECONDS)
- TimeUnit.DAYS.convert(previousDate.getTime(), TimeUnit.MILLISECONDS));
for (Map.Entry entry : SCORES_TABLES.entrySet()) {
if (nbDays > entry.getKey()) {
score = entry.getValue();
break;
}
}
});
}
public String getGcName() {
return gcName;
}
public String getCacheID() {
return cacheUid;
}
public GcLog getMyLog() {
return myLog;
}
public Optional getPreviousLog() {
return Optional.ofNullable(previousLog);
}
public long getNbDays() {
return nbDays;
}
public int getScore() {
return score;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy