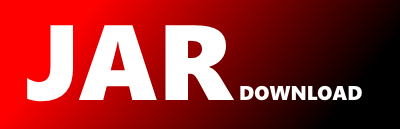
io.ultreia.gc.model.GcLog Maven / Gradle / Ivy
package io.ultreia.gc.model;
/*-
* #%L
* GC toolkit :: API
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.text.DateFormat;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Map;
/**
* Created by tchemit on 18/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class GcLog {
public static GcLog fromMap(Map map) {
GcLog result = new GcLog();
result.setLogID(((Double) map.get("LogID")).longValue());
result.setCacheID(((Double) map.get("CacheID")).longValue());
result.setLogGuid((String) map.get("LogGuid"));
result.setLogType((String) map.get("LogType"));
result.setLogText((String) map.get("LogText"));
result.setCreated((String) map.get("Created"));
result.setUserName((String) map.get("UserName"));
return result;
}
private long LogID;
private long CacheID;
private String LogGuid;
private String LogType;
private String LogText;
private String Created;
private String UserName;
public boolean isFoundByUser(String userName) {
return isFoundIt() && getUserName().equals(userName);
}
public boolean isFoundIt() {
return LogType.equals("Found it");
}
public long getLogID() {
return LogID;
}
public void setLogID(long logID) {
LogID = logID;
}
public long getCacheID() {
return CacheID;
}
public void setCacheID(long cacheId) {
CacheID = cacheId;
}
public String getLogGuid() {
return LogGuid;
}
public void setLogGuid(String logGuid) {
LogGuid = logGuid;
}
public String getLogType() {
return LogType;
}
public void setLogType(String logType) {
LogType = logType;
}
public String getLogText() {
return LogText;
}
public void setLogText(String logText) {
LogText = logText;
}
public String getCreated() {
return Created;
}
public void setCreated(String created) {
Created = created;
}
public String getUserName() {
return UserName;
}
public void setUserName(String userName) {
UserName = userName;
}
public final transient DateFormat df = new SimpleDateFormat("dd/MM/yyyy");
public synchronized Date getDate() {
try {
return df.parse(Created);
} catch (Exception e) {
throw new RuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy