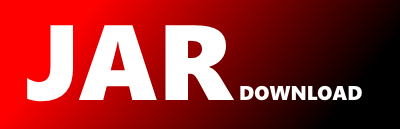
io.ultreia.gc.service.FieldNotesService Maven / Gradle / Ivy
package io.ultreia.gc.service;
/*-
* #%L
* GC toolkit :: API
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.github.mustachejava.DefaultMustacheFactory;
import com.github.mustachejava.Mustache;
import com.github.mustachejava.MustacheFactory;
import io.ultreia.gc.http.GcRequest;
import io.ultreia.gc.http.GcRequestBuilder;
import io.ultreia.gc.http.GcResponse;
import io.ultreia.gc.model.GcFieldNote;
import io.ultreia.gc.session.GcSession;
import java.io.IOException;
import java.io.StringReader;
import java.io.StringWriter;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
/**
* Created by tchemit on 16/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class FieldNotesService extends GcServiceSupport {
/** Logger. */
private static final Log log = LogFactory.getLog(FieldNotesService.class);
private MustacheFactory mustacheFactory;
/**
* @return the names of caches you got in your Geocaching.com field notes
*/
public List loadFieldNotes() {
GcSession gcSession = getGcSession();
GcRequest request = gcSession.forGet()
.build("https://www.geocaching.com/my/fieldnotes.aspx");
GcResponse build = gcSession.executeRequest(request);
Document doc = build.getResponseAsHtml();
Elements trs = doc.select("table[class=Table] > tbody > tr");
List fieldNotes = new LinkedList<>();
for (Element tr : trs) {
Elements select = tr.select("td > a[href^=https://www]");
String name = select.text();
String url = select.attr("href");
String date = tr.child(2).text();
String logType = tr.child(3).text();
String composeUrl = tr.child(4).select(" a[href^=fieldnotes.aspx?composeLog=]").first().attr("href");
fieldNotes.add(new GcFieldNote(name, url, composeUrl, date, logType));
}
log.info("Found " + fieldNotes.size() + " field note(s).");
fieldNotes.forEach(c -> log.debug("Cache " + c + " - " + c.getComposeUrl()));
return fieldNotes;
}
public void logFieldNote(GcFieldNote fieldNote, String logText, boolean autoVisitTrackables, ProgressMonitor progressBarModel) {
progressBarModel.increment("Prepare log for cache: " + fieldNote.getName());
GcSession gcSession = getGcSession();
GcRequest request = gcSession.forGet()
.build("https://www.geocaching.com/my/" + fieldNote.getComposeUrl());
GcResponse build = gcSession.executeRequest(request);
Document doc = build.getResponseAsHtml();
String logType = doc.select("select[id='ctl00_ContentBody_LogBookPanel1_ddLogType'] > option[selected='selected']").attr("value");
String date = doc.select("input[id='uxDateVisited']").attr("value");
String theOwner = doc.select("div[id='ctl00_ContentBody_LogBookPanel1_EditLogPanel'] > div > dl > dd > a:nth-child(4)").text();
int cacheCount = Integer.valueOf(doc.select("span[class='cache-count']").text().split(" ")[0].replace(",", ""));
log.info("cache count: " + cacheCount);
String action = doc.select("form[id='aspnetForm']").attr("action").replace("./", "");
log.info("action:" + action);
log.info("the owner: " + theOwner);
Mustache mustache = getMustacheFactory().compile(new StringReader(logText), "archeo");
Map mustacheModel = new HashMap<>();
mustacheModel.put("found", cacheCount + 1);
mustacheModel.put("owner", theOwner);
try (StringWriter logTextWriter = new StringWriter()) {
mustache.execute(logTextWriter, mustacheModel);
logTextWriter.flush();
logText = logTextWriter.toString();
} catch (IOException e) {
throw new RuntimeException(e);
}
GcRequestBuilder requestBuilder = gcSession.forPost()
.addParameter("ctl00$ContentBody$LogBookPanel1$uxLogCreationSource", "Old")
.addParameter("ctl00$ContentBody$LogBookPanel1$IsEditLog", "False")
.addParameter("ctl00$ContentBody$LogBookPanel1$ddLogType", logType)
.addParameter("ctl00$ContentBody$LogBookPanel1$uxDateVisited", date)
.addParameter("ctl00$ContentBody$LogBookPanel1$uxLogInfo", logText)
.addParameter("ctl00$ContentBody$LogBookPanel1$btnSubmitLog", "Submit Log Entry");
requestBuilder.addHiddenInputs(doc);
if (autoVisitTrackables) {
requestBuilder.addParameter("ctl00$ContentBody$LogBookPanel1$chkAddToFavorites", "on");
StringBuilder tbIds = new StringBuilder();
for (Element tbSelect : doc.select("table[id='tblTravelBugs']>tbody > tr> td > select")) {
String tbName = tbSelect.attr("name");
String tbId = doc.select("select[name='" + tbName + "'] > option:nth-child(3)").attr("value");
requestBuilder.addParameter(tbName, tbId);
tbIds.append(tbId).append(",");
}
requestBuilder.addParameter("ctl00$ContentBody$LogBookPanel1$uxTrackables$hdnSelectedActions", tbIds.toString());
}
GcRequest request1 = requestBuilder.build("https://www.geocaching.com/seek/" + action);
progressBarModel.increment("Send log for cache: " + fieldNote.getName());
GcResponse response = gcSession.executeRequest(request1);
Integer statusCode = response.getStatusCode();
log.info("Status code: " + statusCode);
}
private MustacheFactory getMustacheFactory() {
if (mustacheFactory == null) {
mustacheFactory = new DefaultMustacheFactory();
}
return mustacheFactory;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy