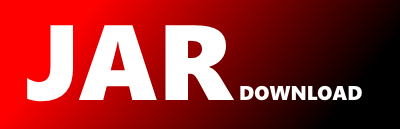
io.ultreia.gc.service.GcCacheService Maven / Gradle / Ivy
package io.ultreia.gc.service;
/*-
* #%L
* GC toolkit :: API
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.gson.GsonBuilder;
import io.ultreia.gc.http.GcRequest;
import io.ultreia.gc.http.GcResponse;
import io.ultreia.gc.model.GcLog;
import io.ultreia.gc.session.GcSession;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
/**
* Created by tchemit on 18/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class GcCacheService extends GcServiceSupport {
/** Logger. */
private static final Log log = LogFactory.getLog(GcCacheService.class);
public void getCacheFromGuid(String guid) {
GcSession gcSession = getGcSession();
GcRequest request = gcSession.forGet()
.addParameter("guid", guid)
.build("https://www.geocaching.com/seek/cache_details.aspx");
GcResponse build = gcSession.executeRequest(request);
Document doc = build.getResponseAsHtml();
String userToken = null;
String logs = null;
List allLogs = new LinkedList<>();
for (Element element : doc.select("script[type='text/javascript']")) {
String text = element.data();
if (text.contains("userToken = '")) {
int i = text.indexOf("userToken =");
int start = text.indexOf("'", i) + 1;
int end = text.indexOf("'", start);
userToken = text.substring(start, end);
log.info("User token: " + userToken);
i = text.indexOf("initalLogs = ");
start = text.indexOf("{", i);
end = text.indexOf("};", start) + 1;
logs = text.substring(start, end);
HashMap hashMap = new GsonBuilder().setPrettyPrinting().create().fromJson(logs, HashMap.class);
List data = (List) hashMap.get("data");
for (Object datum : data) {
GcLog gcLog = GcLog.fromMap((Map) datum);
allLogs.add(gcLog);
}
log.info("logs: " + hashMap);
}
}
String uploadUrl = doc.select("a[title='Upload Image']").attr("href");
log.info("Upload image url: " + uploadUrl);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy