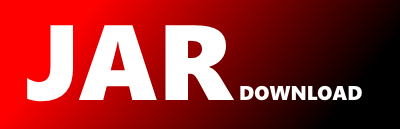
io.ultreia.gc.session.GcSession Maven / Gradle / Ivy
package io.ultreia.gc.session;
/*-
* #%L
* GC toolkit :: API
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.gc.http.GcExecuteRequestException;
import io.ultreia.gc.http.GcLoginException;
import io.ultreia.gc.http.GcRequest;
import io.ultreia.gc.http.GcRequestBuilder;
import io.ultreia.gc.http.GcResponse;
import io.ultreia.gc.http.GcResponseBuilder;
import java.io.Closeable;
import java.io.IOException;
import java.util.Arrays;
import java.util.LinkedHashMap;
import java.util.Map;
import java.util.Objects;
import java.util.UUID;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.jsoup.nodes.Document;
/**
* Created by tchemit on 13/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class GcSession implements Closeable {
private static final Log log = LogFactory.getLog(GcSession.class);
private static final Map HEADERS;
static {
HEADERS = new LinkedHashMap<>();
HEADERS.put("User-Agent", "Mozilla/5.0 (Windows NT 6.1; rv:48.0) Gecko/20100101 Firefox/48.0");
HEADERS.put("Accept", "text/html,application/xhtml+xml,application/xml;q=0.9,*/*;q=0.8");
HEADERS.put("Accept-Language", "fr-fr,en-us;q=0.7,en;q=0.3");
HEADERS.put("DNT", "1");
HEADERS.put("Connection", "keep-alive");
}
private final GcResponseBuilder responseBuilder = GcResponseBuilder.create();
private final String username;
private final String id;
public GcRequestBuilder forGet() {
return GcRequestBuilder.forGet().addHeaders(HEADERS);
}
public GcRequestBuilder forPost() {
return GcRequestBuilder.forPost().addHeaders(HEADERS);
}
GcSession(String username, String password) {
this.username = username;
this.id = UUID.randomUUID().toString();
GcRequest request = forGet().build("https://www.geocaching.com/account/login");
GcResponse build;
try {
build = responseBuilder.executeRequest(request);
} catch (IOException e) {
throw new GcLoginException("can't get login page", e);
}
Document doc = build.getResponseAsHtml();
String requestVerificationToken = doc.select("input[name='__RequestVerificationToken'][type='hidden']").attr("value");
log.debug("User requestVerificationToken: " + requestVerificationToken);
GcRequest request1 = GcRequestBuilder.forPost()
.addHeaders(HEADERS)
.addParameter("UserName", username)
.addParameter("Password", password)
.addParameter("__RequestVerificationToken", requestVerificationToken)
.build("https://www.geocaching.com/account/login");
GcResponse build1;
try {
build1 = responseBuilder.executeRequest(request1);
} catch (IOException e) {
throw new GcLoginException("can't submit login page", e);
}
log.debug("status code: " + build1.getStatusCode());
log.debug("headers: " + Arrays.toString(build1.getResponseHeaders()));
if (build1.getStatusCode() == 302) {
log.info("Log in.");
} else {
throw new GcLoginException(build1.getResponseAsString());
}
}
public GcResponse executeRequest(GcRequest request) {
try {
return responseBuilder.executeRequest(request);
} catch (IOException e) {
throw new GcExecuteRequestException(e);
}
}
@Override
public void close() {
try {
responseBuilder.close();
} catch (IOException e) {
throw new RuntimeException(e);
}
}
public String getUsername() {
return username;
}
public String getId() {
return id;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GcSession gcSession = (GcSession) o;
return Objects.equals(id, gcSession.id);
}
@Override
public int hashCode() {
return Objects.hash(id);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy