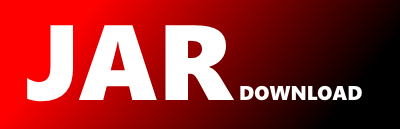
io.ultreia.gc.lfn.actions.BoxHeadingAction Maven / Gradle / Ivy
package io.ultreia.gc.lfn.actions;
/*-
* #%L
* GC toolkit :: LFN
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.Point;
import java.util.Arrays;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.swing.JTextArea;
import javax.swing.KeyStroke;
import javax.swing.text.BadLocationException;
import javax.swing.text.Document;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
/**
* Created by tchemit on 18/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class BoxHeadingAction extends BoxTextAction {
/** Logger. */
private static final Log log = LogFactory.getLog(BoxHeadingAction.class);
private static final Pattern HEADING_PATTERN = Pattern.compile("(#+\\s+)?([^#]+)(\\s+#+)");
private final int level;
private static String leftBox(int level) {
char[] tmp = new char[level + 1];
Arrays.fill(tmp, '#');
tmp[level] = ' ';
return new String(tmp);
}
private static String rightBox(int level) {
char[] tmp = new char[level + 1];
Arrays.fill(tmp, '#');
tmp[0] = ' ';
return new String(tmp);
}
public BoxHeadingAction(int level, JTextArea logText, KeyStroke keyStroke) {
super(leftBox(level), rightBox(level), "Heading", "/icons/text_heading_" + level + ".png", logText, keyStroke);
this.level = level;
}
@Override
void insertAtCaretPosition(int caretPosition) throws BadLocationException {
String text = logText.getText();
Point point = getCurrentLine(text, caretPosition);
apply(text, point);
}
@Override
void updateFromSelection(int start, String selectedText) throws BadLocationException {
String text = logText.getText();
Point point = getCurrentLine(text, start);
apply(text, point);
}
private void apply(String text, Point point) throws BadLocationException {
boolean emptyLine = point.x > point.y;
String currentLine = emptyLine ? "" : text.substring(point.x, point.y).trim();
if (emptyLine) {
point.x--;
}
log.info("Current Line: " + currentLine);
String newSelectedText;
boolean doBox = true;
Matcher matcher = HEADING_PATTERN.matcher(currentLine);
if (matcher.matches()) {
// already boxed
int oldLevel = matcher.group(1).length() - 1;
newSelectedText = matcher.group(2);
if (level == oldLevel) {
doBox = false;
}
} else {
// need to box
newSelectedText = currentLine;
if (newSelectedText.isEmpty()) {
newSelectedText = this.str;
}
}
log.info("new Selected Text: " + newSelectedText);
Document document = logText.getDocument();
document.remove(point.x, point.y - point.x);
document.insertString(point.x, doBox ? box(newSelectedText) : newSelectedText, null);
int start = point.x;
if (doBox) {
start += boxLeftSize;
}
logText.setSelectionStart(start);
logText.setSelectionEnd(start + newSelectedText.length());
}
private Point getCurrentLine(String text, int current) {
int start = current;
int end = current;
if (text.charAt(start) == '\n') {
start--;
}
while (start > 0 && text.charAt(start) != '\n') {
start--;
}
int textLength = text.length();
while (end < textLength && text.charAt(end) != '\n') {
end++;
}
if (text.charAt(start) == '\n' || text.charAt(start) == '\r') {
start++;
}
if (text.charAt(end) == '\n' || text.charAt(end) == '\r') {
end--;
}
return new Point(start, end + 1);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy