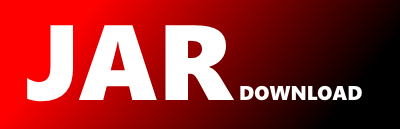
io.ultreia.gc.lfn.ui.LogFieldNotesContentPanelUI Maven / Gradle / Ivy
package io.ultreia.gc.lfn.ui;
/*-
* #%L
* GC toolkit :: LFN
* %%
* Copyright (C) 2017 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.gc.lfn.GcLfnConfig;
import io.ultreia.gc.lfn.LogFieldNotesContext;
import io.ultreia.gc.lfn.actions.ReloadFieldNotesAction;
import io.ultreia.gc.lfn.actions.ResetFavoriteAction;
import io.ultreia.gc.lfn.actions.ResetFieldNoteAction;
import io.ultreia.gc.lfn.actions.SelectAllFavoritesAction;
import io.ultreia.gc.lfn.actions.SelectAllFieldNotesAction;
import io.ultreia.gc.lfn.actions.SelectFavoriteAction;
import io.ultreia.gc.lfn.actions.SelectFieldNoteAction;
import io.ultreia.gc.lfn.actions.SendLogsAction;
import io.ultreia.gc.model.GcFieldNote;
import io.ultreia.gc.ui.ContentPanelUI;
import io.ultreia.gc.ui.actions.LogOutAction;
import io.ultreia.gc.ui.actions.QuitAction;
import java.awt.BorderLayout;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.GridLayout;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import javax.swing.ImageIcon;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JLabel;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTable;
import javax.swing.JTextArea;
import javax.swing.JToolBar;
import javax.swing.table.DefaultTableCellRenderer;
import javax.swing.table.TableColumnModel;
import org.nuiton.jaxx.runtime.swing.SwingUtil;
/**
* Created by tchemit on 16/04/17.
*
* @author Tony Chemit - [email protected]
*/
public class LogFieldNotesContentPanelUI extends ContentPanelUI {
private final LogFieldNotesUI ui;
private final LogFieldNotesUIModel model;
private final JTable fieldNotesTable;
private final JTextArea logText;
private final JButton sendLogs;
private final JButton reloadFieldNotes;
private final JButton logOut;
private final JToolBar fieldNotesTableHeader;
private final JLabel fieldNotesTableHeaderStatusLabel;
LogFieldNotesContentPanelUI(LogFieldNotesUI ui, LogFieldNotesUIModel model) {
this.ui = ui;
this.model = model;
setLayout(new BorderLayout());
JPanel splitPane = new JPanel();
splitPane.setLayout(new GridLayout(1, 2));
add(splitPane, BorderLayout.CENTER);
JPanel leftPanel = new JPanel();
leftPanel.setLayout(new BorderLayout());
splitPane.add(leftPanel);
JPanel configuration = new JPanel();
configuration.setLayout(new GridLayout(0, 1));
leftPanel.add(configuration, BorderLayout.NORTH);
JPanel autoVisitTrackablesPanel = new JPanel();
autoVisitTrackablesPanel.setLayout(new BorderLayout());
configuration.add(autoVisitTrackablesPanel);
autoVisitTrackablesPanel.add(new JLabel("Automatic visit of trackables?"), BorderLayout.WEST);
JCheckBox autoVisitTrackablesCheckBox = new JCheckBox();
autoVisitTrackablesCheckBox.setSelected(this.model.isAutoVisitTrackables());
autoVisitTrackablesPanel.add(autoVisitTrackablesCheckBox, BorderLayout.EAST);
autoVisitTrackablesCheckBox.addChangeListener(e -> {
this.model.setAutoVisitTrackables(autoVisitTrackablesCheckBox.isSelected());
updateApply();
});
JPanel leftPane = new JPanel();
leftPane.setLayout(new BorderLayout());
leftPanel.add(leftPane, BorderLayout.CENTER);
LogFieldNotesTableModel logFieldNotesTableModel = new LogFieldNotesTableModel(this.model);
fieldNotesTable = new JTable(logFieldNotesTableModel);
DefaultTableCellRenderer renderer = new DefaultTableCellRenderer() {
private Font defaultFont;
private Font selectedFont;
private final ImageIcon favoriteIcon = SwingUtil.createIcon("/icons/favorite.png");
@Override
public Component getTableCellRendererComponent(JTable table, Object value, boolean isSelected, boolean hasFocus, int row, int column) {
GcFieldNote fieldNote = logFieldNotesTableModel.getFieldNote(row);
super.getTableCellRendererComponent(table, value, isSelected, hasFocus, row, column);
if (column == 0) {
setText(null);
setIcon(fieldNote.isFavorite() ? favoriteIcon : null);
} else {
setIcon(null);
Font font = getFont(table, fieldNote.isSelected());
setFont(font);
}
return this;
}
private Font getFont(JTable table, boolean selected) {
if (selectedFont == null) {
defaultFont = table.getFont();
selectedFont = defaultFont.deriveFont(Font.BOLD);
}
return selected ? selectedFont : defaultFont;
}
};
fieldNotesTable.setDefaultRenderer(String.class, renderer);
fieldNotesTable.setDefaultRenderer(boolean.class, renderer);
fieldNotesTable.setAutoResizeMode(JTable.AUTO_RESIZE_LAST_COLUMN);
TableColumnModel columnModel = fieldNotesTable.getColumnModel();
columnModel.getColumn(0).setMinWidth(20);
columnModel.getColumn(0).setMaxWidth(20);
columnModel.getColumn(1).setMinWidth(80);
columnModel.getColumn(1).setMaxWidth(80);
columnModel.getColumn(2).setMinWidth(100);
columnModel.getColumn(2).setMaxWidth(100);
fieldNotesTable.setMinimumSize(new Dimension(600, 200));
fieldNotesTableHeader = new JToolBar();
fieldNotesTableHeader.setBorderPainted(false);
fieldNotesTableHeader.setFloatable(false);
fieldNotesTableHeader.setOpaque(true);
fieldNotesTableHeaderStatusLabel = new JLabel();
JPanel fieldNotesTableHeaderStatus = new JPanel();
fieldNotesTableHeaderStatus.setLayout(new BorderLayout());
fieldNotesTableHeaderStatus.add(fieldNotesTableHeaderStatusLabel, BorderLayout.WEST);
leftPane.add(fieldNotesTableHeader, BorderLayout.NORTH);
leftPane.add(new JScrollPane(fieldNotesTable), BorderLayout.CENTER);
leftPane.add(fieldNotesTableHeaderStatus, BorderLayout.SOUTH);
JScrollPane scrollPane = new JScrollPane();
splitPane.add(scrollPane);
logText = new JTextArea(this.model.getLogText());
scrollPane.setViewportView(logText);
LogTextHeader logTextHeader = new LogTextHeader(logText);
scrollPane.setColumnHeaderView(logTextHeader);
logText.addKeyListener(new KeyAdapter() {
@Override
public void keyReleased(KeyEvent e) {
LogFieldNotesContentPanelUI.this.model.setLogText(logText.getText());
updateApply();
}
});
JPanel bottomPanel = new JPanel();
bottomPanel.setLayout(new BorderLayout());
add(bottomPanel, BorderLayout.SOUTH);
JPanel actions = new JPanel();
actions.setLayout(new GridLayout(1, 0));
bottomPanel.add(actions, BorderLayout.SOUTH);
sendLogs = new JButton();
reloadFieldNotes = new JButton();
logOut = new JButton();
actions.add(logOut);
actions.add(reloadFieldNotes);
actions.add(sendLogs);
}
@Override
public void installActions(LogFieldNotesContext applicationContext, LogFieldNotesUIModel model) {
fieldNotesTable.getInputMap(WHEN_ANCESTOR_OF_FOCUSED_COMPONENT).getParent().put(SelectFavoriteAction.KEY_STROKE, "none");
fieldNotesTableHeader.add(new SelectAllFieldNotesAction(ui));
fieldNotesTableHeader.add(new SelectAllFavoritesAction(ui));
new SelectFieldNoteAction(ui);
new ResetFieldNoteAction(ui);
new SelectFavoriteAction(ui);
new ResetFavoriteAction(ui);
new QuitAction<>(ui);
SendLogsAction sendLogsAction = new SendLogsAction(ui, model, applicationContext.newFieldNotesService());
sendLogs.setAction(sendLogsAction);
sendLogs.setText("Send");
ReloadFieldNotesAction reloadFieldNotesAction = new ReloadFieldNotesAction(ui);
reloadFieldNotes.setAction(reloadFieldNotesAction);
reloadFieldNotes.setText("Reload");
LogOutAction logOutAction = new LogOutAction<>(ui);
logOut.setAction(logOutAction);
logOut.setText("Log in");
}
public JTable getFieldNotesTable() {
return fieldNotesTable;
}
@Override
public void updateApply() {
fieldNotesTableHeaderStatusLabel.setText(String.format("Selected %d out of %d (favorites: %d)", model.getNbSelected(), model.getNbFieldNotes(), model.getNbFavorites()));
sendLogs.setEnabled(model.isValid());
}
@Override
public void setBusy(boolean value) {
boolean b = !value;
logOut.setEnabled(b);
logText.setEnabled(b);
fieldNotesTable.setEnabled(b);
boolean canSend = b;
if (b) {
LogFieldNotesContext applicationContext = ui.getApplicationContext();
b = applicationContext.getAuthId().isPresent();
canSend = b && model.isValid();
}
sendLogs.setEnabled(canSend);
reloadFieldNotes.setEnabled(b);
}
@Override
public JButton getLogOut() {
return logOut;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy