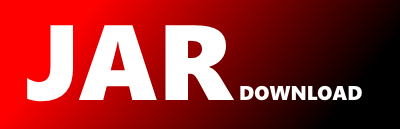
io.ultreia.java4all.config.plugin.ReportMojoSupport Maven / Gradle / Ivy
Show all versions of config-maven-plugin Show documentation
package io.ultreia.java4all.config.plugin;
/*
* #%L
* Config :: Maven plugin
* %%
* Copyright (C) 2016 - 2018 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.apache.maven.doxia.siterenderer.Renderer;
import org.apache.maven.plugins.annotations.Component;
import org.apache.maven.plugins.annotations.Parameter;
import org.apache.maven.project.MavenProject;
import org.apache.maven.reporting.AbstractMavenReport;
import org.apache.maven.reporting.MavenReportException;
import org.codehaus.plexus.i18n.I18N;
import org.codehaus.plexus.util.StringUtils;
import io.ultreia.java4all.config.ApplicationConfigHelper;
import io.ultreia.java4all.config.ApplicationConfigProvider;
import org.nuiton.i18n.I18n;
import org.nuiton.i18n.init.ClassPathI18nInitializer;
import org.nuiton.i18n.init.DefaultI18nInitializer;
import org.nuiton.i18n.init.I18nInitializer;
import java.io.File;
import java.net.MalformedURLException;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.Arrays;
import java.util.HashSet;
import java.util.Locale;
import java.util.Set;
/**
* Abstract application config report used by normal and aggregate report.
*
* @author Tony - [email protected]
* @since 3.0
*/
abstract class ReportMojoSupport extends AbstractMavenReport {
/**
* Report output directory. Note that this parameter is only relevant if the goal is run from the command line or
* from the default build lifecycle. If the goal is run indirectly as part of a site generation, the output
* directory configured in the Maven Site Plugin is used instead.
*/
@Parameter(property = "config.outputDirectory", defaultValue = "${project.reporting.outputDirectory}", required = true)
private File outputDirectory;
/**
* Report output encoding. Note that this parameter is only relevant if the goal is run from the command line or
* from the default build lifecycle. If the goal is run indirectly as part of a site generation, the output
* encoding configured in the Maven Site Plugin is used instead.
*/
@Parameter(property = "config.outputEncoding", defaultValue = "${project.reporting.outputEncoding}", required = true)
protected String outputEncoding;
/**
* Optional i18n bundle name as used by the nuiton I18n system to init his
* files.
*
* If not given, will look at all i18n files in all over class-path (which
* could be costy if many dependencies), otherwise will init I18n system
* usinga {@link DefaultI18nInitializer} with this bundle name.
*/
@Parameter(property = "config.i18nBundleName")
private String i18nBundleName;
/**
* List of application config to include in report (separated by comma).
*
* Note: If not filled then will use all config
* found in class-path.
*/
@Parameter(property = "config.include")
private String include;
/**
* List of application config to exclude from report (separated by comma).
*
* Note: If not filled no config will be exclude.
*/
@Parameter(property = "config.exclude")
private String exclude;
/**
* Flag to activate verbose mode.
*
* Note: Verbose mode is always on if you starts a debug maven instance
* (says via {@code -X}).
*/
@Parameter(property = "config.verbose", defaultValue = "${maven.verbose}")
private boolean verbose;
/**
* Skip to generate the report.
*/
@Parameter(property = "config.skip")
private boolean skip;
/**
* The Maven Project.
*/
@Parameter(defaultValue = "${project}", required = true)
private MavenProject project;
/**
* Doxia Site Renderer component.
*/
@Component
private Renderer siteRenderer;
/**
* Internationalization component.
*/
@Component
private I18N i18n;
/**
* Class loader with all compile dependencies of the module in class-path
* (used to init i18n) and obtain config provider over compile class-path.
*/
private ClassLoader newClassLoader;
/**
* Set of ApplicationConfigProvider detected from configuration.
*/
private Set configProviders;
@Override
public String getOutputName() {
return "config-report";
}
public String getDescription(Locale locale) {
return i18n.getString(getOutputName(), locale, "report.description");
}
public String getName(Locale locale) {
return i18n.getString(getOutputName(), locale, "report.title");
}
@Override
public String getCategoryName() {
return CATEGORY_PROJECT_REPORTS;
}
@Override
public boolean canGenerateReport() {
return !skip;
}
@Override
protected Renderer getSiteRenderer() {
return siteRenderer;
}
@Override
protected String getOutputDirectory() {
return outputDirectory.getAbsolutePath();
}
@Override
protected MavenProject getProject() {
return project;
}
protected abstract ClassLoader createClassLoader() throws MavenReportException;
@Override
protected void executeReport(Locale locale) throws MavenReportException {
if (newClassLoader == null) {
// not init
init(locale);
} else {
// just change init language
I18n.setDefaultLocale(locale);
}
ReportRenderer renderer =
new ReportRenderer(getSink(),
i18n,
locale,
getOutputName(),
getOutputName(),
configProviders);
renderer.render();
}
private void init(Locale locale) throws MavenReportException {
if (getLog().isDebugEnabled()) {
// debug mode set verbose flag to true
verbose = true;
}
if (newClassLoader == null) {
newClassLoader = createClassLoader();
}
// get i18n initializer
I18nInitializer initializer;
if (StringUtils.isNotEmpty(i18nBundleName)) {
initializer = new DefaultI18nInitializer(i18nBundleName,
newClassLoader);
} else {
initializer = new ClassPathI18nInitializer(newClassLoader);
}
// init i18n
I18n.init(initializer, locale);
if (configProviders == null) {
Set includes = null;
if (StringUtils.isNotEmpty(include)) {
includes = new HashSet<>(Arrays.asList(include.split("\\s*,\\s*")));
if (verbose) {
getLog().info("config - includes : " + includes);
}
}
Set excludes = null;
if (StringUtils.isNotEmpty(exclude)) {
excludes = new HashSet<>(Arrays.asList(exclude.split("\\s*,\\s*")));
if (verbose) {
getLog().info("config - excludes : " + excludes);
}
}
configProviders = ApplicationConfigHelper.getProviders(
newClassLoader,
includes,
excludes,
verbose
);
}
}
ClassLoader createClassLoader(Set paths) {
Set urls = new HashSet<>();
for (String fileName : paths) {
File pathElem = new File(fileName);
try {
URL url = pathElem.toURI().toURL();
urls.add(url);
getLog().debug("Added classpathElement URL " + url);
} catch (MalformedURLException e) {
throw new RuntimeException(
"Error in adding the classpath " + pathElem, e);
}
}
return new URLClassLoader(
urls.toArray(new URL[urls.size()]),
Thread.currentThread().getContextClassLoader());
}
}