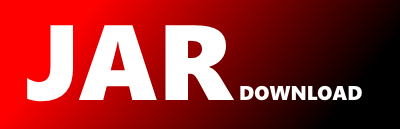
io.ultreia.java4all.config.plugin.ReportRenderer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of config-maven-plugin Show documentation
Show all versions of config-maven-plugin Show documentation
Maven plugin to use the config library
package io.ultreia.java4all.config.plugin;
/*
* #%L
* Config :: Maven plugin
* %%
* Copyright (C) 2016 - 2018 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.base.Joiner;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.maven.doxia.sink.Sink;
import org.apache.maven.doxia.sink.impl.SinkEventAttributeSet;
import org.apache.maven.reporting.AbstractMavenReportRenderer;
import org.codehaus.plexus.i18n.I18N;
import org.codehaus.plexus.util.StringUtils;
import io.ultreia.java4all.config.ApplicationConfigProvider;
import io.ultreia.java4all.config.ConfigActionDef;
import io.ultreia.java4all.config.ConfigOptionDef;
import java.util.Locale;
import java.util.Set;
import static org.nuiton.i18n.I18n.l;
/**
* Render the {@link ReportMojo} report.
*
* @author Tony - [email protected]
* @since 3.0
*/
class ReportRenderer extends AbstractMavenReportRenderer {
/**
* Internationalization component.
*/
private final I18N i18n;
/**
* The locale we are rendering for.
*/
private final Locale locale;
/**
* The report physical name (used to generated link).
*/
private final String reportName;
/**
* The name of the bundle containing our I18n resources.
*/
private final String bundleName;
/**
* Set of config providers to generate.
*/
private final Set configProviders;
ReportRenderer(Sink sink,
I18N i18n,
Locale locale,
String reportName,
String bundleName,
Set configProviders) {
super(sink);
this.i18n = i18n;
this.locale = locale;
this.reportName = reportName;
this.bundleName = bundleName;
this.sink = sink;
this.configProviders = configProviders;
}
@Override
public String getTitle() {
return getText("report.title");
}
@Override
public void renderBody() {
sink.section1();
sink.sectionTitle1();
sink.text(getText("report.overview.title"));
sink.sectionTitle1_();
sink.lineBreak();
sink.paragraph();
sink.text(getText("report.overview.text"));
sink.paragraph_();
sink.paragraph();
sink.link("http://config.nuiton.org/v/latest/index.html");
sink.text(getText("report.overview.more.information"));
sink.link_();
sink.paragraph_();
sink.lineBreak();
renderProviderSummaryTable();
sink.section1_();
if (CollectionUtils.isNotEmpty(configProviders)) {
sink.section1();
sink.sectionTitle1();
sink.text(getText("report.detail.title"));
sink.sectionTitle1_();
sink.lineBreak();
sink.paragraph();
sink.text(getText("report.detail.text"));
sink.paragraph_();
for (ApplicationConfigProvider configProvider : configProviders) {
renderConfigProviderDetail(configProvider);
}
sink.section1_();
}
}
private void renderProviderSummaryTable() {
if (CollectionUtils.isEmpty(configProviders)) {
sink.text(getText("report.detail.text.noConfig"));
} else {
sink.table();
sink.tableRow();
sinkHeaderCellText(getText("report.config.name"));
sinkHeaderCellText(getText("report.config.description"));
sinkHeaderCellText(getText("report.config.nbOptions"));
sinkHeaderCellText(getText("report.config.nbActions"));
sink.tableRow_();
for (ApplicationConfigProvider configProvider : configProviders) {
sink.tableRow();
sinkCellLink(configProvider.getName(), "detail_" + configProvider.getName());
sinkCellText(configProvider.getDescription(locale));
sinkCellText(configProvider.getOptions().length + "");
sinkCellText(configProvider.getActions().length + "");
sink.tableRow_();
}
sink.table_();
}
}
private void renderConfigProviderDetail(ApplicationConfigProvider configProvider) {
sink.section2();
sink.sectionTitle2();
sink.anchor("detail_" + configProvider.getName());
sink.text(getText("report.detail.configuration.title") + " " + configProvider.getName());
sink.anchor_();
sink.sectionTitle2_();
sink.paragraph();
sink.text(getText("report.config.name") + " : ");
sink.bold();
sink.text(configProvider.getName());
sink.bold_();
sink.paragraph_();
sink.paragraph();
sink.text(getText("report.config.description") + " : ");
sink.bold();
sink.text(configProvider.getDescription(locale));
sink.bold_();
sink.paragraph_();
sink.section3();
sink.sectionTitle3();
sink.anchor("detail_options_" + configProvider.getName());
sink.text(getText("report.detail.options.title"));
sink.anchor_();
sink.sectionTitle3_();
sink.lineBreak();
renderOptionDefsTable(configProvider.getOptions());
sink.section3_();
sink.section3();
sink.sectionTitle3();
sink.anchor("detail_actions_" + configProvider.getName());
sink.text(getText("report.detail.actions.title"));
sink.anchor_();
sink.sectionTitle3_();
sink.lineBreak();
renderActionDefsTable(configProvider.getActions());
sink.section3_();
sink.section2_();
}
private void renderOptionDefsTable(ConfigOptionDef... options) {
if (options.length == 0) {
sink.paragraph();
sink.bold();
sink.text(getText("report.detail.options.noOptions"));
sink.bold_();
sink.paragraph_();
} else {
sink.table();
sink.tableRow();
sinkHeaderCellText(getText("report.config.option.key"));
sinkHeaderCellText(getText("report.config.option.description"));
sinkHeaderCellText(getText("report.config.option.defaultValue"));
sinkHeaderCellText(getText("report.config.option.final"));
sinkHeaderCellText(getText("report.config.option.transient"));
sinkHeaderCellText(getText("report.config.option.type"));
sink.tableRow_();
for (ConfigOptionDef option : options) {
sink.tableRow();
sinkCellText(option.getKey());
sinkCellText(l(locale, option.getDescription()));
sinkCellVerbatimText(getDefaultValue(option));
sinkCellText(getText(!option.isFinal()));
sinkCellText(getText(!option.isTransient()));
sinkCellVerbatimText(option.getType().getName());
sink.tableRow_();
}
sink.table_();
}
}
private void renderActionDefsTable(ConfigActionDef... actions) {
if (actions.length == 0) {
sink.paragraph();
sink.bold();
sink.text(getText("report.detail.actions.noActions"));
sink.bold_();
sink.paragraph_();
} else {
sink.table();
sink.tableRow();
sinkHeaderCellText(getText("report.config.action.description"));
sinkHeaderCellText(getText("report.config.action.aliases"));
sinkHeaderCellText(getText("report.config.action.action"));
sink.tableRow_();
for (ConfigActionDef action : actions) {
sink.tableRow();
sinkCellText(l(locale, action.getDescription()));
sinkCellText(Joiner.on(",").join(action.getAliases()));
sinkCellText(action.getAction());
sink.tableRow_();
}
sink.table_();
}
}
private String getText(boolean key) {
return getText("report." + String.valueOf(key));
}
private String getText(String key) {
return i18n.getString(bundleName, locale, key);
}
private String getDefaultValue(ConfigOptionDef option) {
String defaultValue = option.getDefaultValue();
if (StringUtils.isBlank(defaultValue)) {
defaultValue = getText("report.noDefaultValue");
}
return defaultValue;
}
private void sinkHeaderCellText(String text) {
sink.tableHeaderCell();
sink.text(text);
sink.tableHeaderCell_();
}
private void sinkCellText(String text) {
sink.tableCell();
sink.text(text);
sink.tableCell_();
}
private void sinkCellVerbatimText(String text) {
sink.tableCell();
sink.verbatim(SinkEventAttributeSet.MONOSPACED);
sink.text(text);
sink.verbatim_();
sink.tableCell_();
}
private void sinkCellLink(String text, String url) {
sink.tableCell();
sinkLinkToAnchor(text, url);
sink.tableCell_();
}
private void sinkLinkToAnchor(String text, String anchor) {
sink.link("./" + reportName + ".html#" + anchor);
sink.text(text);
sink.link_();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy