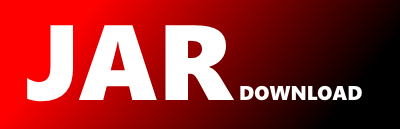
org.nuiton.i18n.bundle.I18nBundle Maven / Gradle / Ivy
/*
* #%L
* I18n :: Api
* %%
* Copyright (C) 2004 - 2017 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.i18n.bundle;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
/**
* Class to represent a i18n Bundle.
*
* A bundle is defined by a resource prefix (eg /tmp/bundle.properties), and a
* list of locale implemented entries.
*
* The property {@link #bundlePrefix} is the equals order property.
*
* The property {@link #entries} contains all entries defined for this bundle.
*
* The method {@link #getEntries(Locale)} filter entries for a given locale,
* including scope inclusive property.
*
* The method {@link #getEntries(I18nBundleScope)} filter entries for a given
* scope, with no inclusive logi.
*
* Thoses filter methods return result in the order defines in {@link
* I18nBundleEntry}, e.g
*
* XXX.properties
* XXX-fr.properties
* XXX-fr_FR.properties
*
* In that way, we can load resource in the good order : load before more
* general scope to more specialized.
*
* @author Tony Chemit - [email protected]
* @see I18nBundleScope
* @see I18nBundleEntry
*/
public class I18nBundle implements Iterable {
/** Logger. */
static final Logger log = LogManager.getLogger(I18nBundle.class);
/** les entrés du bundle */
protected List entries;
/** le nom du bundle encapsulé (correspond au prefix de l'url de chargement) */
protected final String bundlePrefix;
public I18nBundle(String bundlePrefix) {
this.bundlePrefix = bundlePrefix;
}
public String getBundlePrefix() {
return bundlePrefix;
}
/**
* Obtain the entries for a given locale, with a inclusive scope search.
*
* The order of result respect {@link I18nBundleEntry} order.
*
* @param locale the required locale
* @return the array of entries matching extacly the locale or one of the
* lesser scope one.
*/
public I18nBundleEntry[] getEntries(Locale locale) {
I18nBundleScope scope = I18nBundleScope.valueOf(locale);
List result = new ArrayList<>();
for (I18nBundleEntry entry : entries) {
I18nBundleScope i18nBundleScope = entry.getScope();
// load from general to the max scope and always if there is
// only one bundle entry found
if ((i18nBundleScope == scope ||
i18nBundleScope.ordinal() < scope.ordinal()) &&
entry.matchLocale(locale, scope)) {
result.add(entry);
}
}
return result.toArray(new I18nBundleEntry[result.size()]);
}
/**
* Obtain the entries for a given scope
with no incluvie
* logic.
*
* The order of result respect {@link I18nBundleEntry} order.
*
* @param scope the required scope
* @return the list of entries matching exactly the given scope
*/
public I18nBundleEntry[] getEntries(I18nBundleScope scope) {
List result = new ArrayList<>();
for (I18nBundleEntry entry : entries) {
I18nBundleScope i18nBundleScope = entry.getScope();
// load from general to the max scope and always if there is
// only one bundle entry found
if (i18nBundleScope == scope) {
result.add(entry);
}
}
return result.toArray(new I18nBundleEntry[result.size()]);
}
/** @return number of entries in bundle */
public int size() {
return entries == null ? 0 : entries.size();
}
@Override
public String toString() {
String s = super.toString();
return String.format("<%s, bundlePrefix:%s, size:%d>", s.substring(s.lastIndexOf(".") + 1), bundlePrefix, size());
}
protected List getEntries() {
return entries;
}
protected boolean matchLocale(Locale locale) {
I18nBundleScope scope = I18nBundleScope.valueOf(locale);
boolean result = false;
if (size() != 0) {
for (I18nBundleEntry entry : entries) {
if (entry.matchLocale(locale, scope)) {
result = true;
break;
}
}
}
return result;
}
public boolean addEntry(I18nBundleEntry entry) {
if (entries == null) {
entries = new ArrayList<>();
}
boolean b = entries.add(entry);
if (log.isInfoEnabled()) {
log.info(this + "\n\t" + entry);
}
return b;
}
@Override
public Iterator iterator() {
return entries.iterator();
}
}