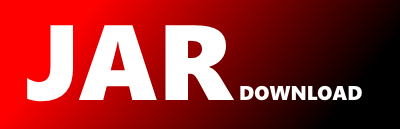
org.nuiton.i18n.init.ClassPathI18nInitializer Maven / Gradle / Ivy
/*
* #%L
* I18n :: Api
* %%
* Copyright (C) 2004 - 2017 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.i18n.init;
import java.net.URL;
import java.net.URLClassLoader;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Iterator;
import java.util.List;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import org.nuiton.i18n.I18nUtil;
import org.nuiton.i18n.bundle.I18nBundle;
import org.nuiton.i18n.bundle.I18nBundleUtil;
/**
* Implementation of a {@link I18nInitializer} using all i18n resources (from
* artifacts) discovered in classpath.
*
* Will scan all classpath.
*
* Note: No order can be predicted with this implementation on bundles.
*
* @author Tony Chemit - [email protected]
* @since 1.1
*/
public class ClassPathI18nInitializer extends I18nInitializer {
/** Logger. */
private static final Logger log =
LogManager.getLogger(ClassPathI18nInitializer.class);
/** class loader to use (optional) */
protected ClassLoader loader;
protected URL[] extraURLs;
public ClassPathI18nInitializer() {
this(null, null);
}
public ClassPathI18nInitializer(ClassLoader loader) {
this(loader, null);
}
public ClassPathI18nInitializer(ClassLoader loader, URL[] extraURLs) {
this.loader = loader == null ? getClass().getClassLoader() : loader;
this.extraURLs = extraURLs;
}
public URL[] resolvURLs() throws Exception {
// on calcule toutes les urls utilisable dans le classloader donnee
List urlToSeek = new ArrayList<>();
URLClassLoader loader = (URLClassLoader) getLoader();
urlToSeek.addAll(Arrays.asList(I18nUtil.getDeepURLs(loader)));
// on va maintenant supprimer toutes les urls qui ne respectent pas
// le pattern i18n : il faut que la resource contienne un repertoire i18n
// ce simple test permet de restreindre la recherche des resources
// i18n qui est tres couteuse
int size = urlToSeek.size();
for (Iterator it = urlToSeek.iterator(); it.hasNext(); ) {
URL url = it.next();
if (!I18nUtil.containsDirectDirectory(
url, I18nBundleUtil.DIRECTORY_SEARCH_BUNDLE_PATTERN)) {
if (log.isDebugEnabled()) {
log.debug(String.format("skip url with no %s directory : %s", I18nBundleUtil.DIRECTORY_SEARCH_BUNDLE_PATTERN, url));
}
it.remove();
}
}
if (log.isDebugEnabled()) {
log.debug(String.format("detect %d i18n capable url (out of %d)", urlToSeek.size(), size));
}
// on effectue la recherche des urls des resources i18n (tous les
// fichiers de traductions) sur toutes les urls precedemment calculees)
URL[] url1 = urlToSeek.toArray(new URL[urlToSeek.size()]);
URL[] result = I18nBundleUtil.getURLs(url1);
if (log.isDebugEnabled()) {
for (URL url : result) {
log.debug(url.toString());
}
}
return result;
}
@Override
public I18nBundle[] resolvBundles() throws Exception {
// detect bundles urls
URL[] urls = resolvURLs();
// detect bundles
return resolvBundles(urls);
}
public URL[] getExtraURLs() {
return extraURLs;
}
public ClassLoader getLoader() {
return loader;
}
}