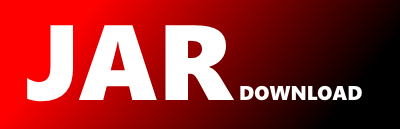
org.nuiton.i18n.init.DefaultI18nInitializer Maven / Gradle / Ivy
/*
* #%L
* I18n :: Api
* %%
* Copyright (C) 2004 - 2017 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
package org.nuiton.i18n.init;
import java.io.InputStream;
import java.net.URL;
import java.nio.charset.Charset;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Locale;
import java.util.Properties;
import org.apache.logging.log4j.Logger;
import org.apache.logging.log4j.LogManager;
import org.nuiton.i18n.I18nUtil;
import org.nuiton.i18n.bundle.I18nBundle;
/**
* Default implementation of a {@link I18nInitializer} using the default i18n
* implementation (one unique bundle with a definition properties file).
*
* @author Tony Chemit - [email protected]
* @since 1.1
*/
public class DefaultI18nInitializer extends I18nInitializer {
/** Logger. */
private static final Logger log =
LogManager.getLogger(DefaultI18nInitializer.class);
public static String UNIQUE_BUNDLE_DEF = "%1$s-definition.properties";
public static String UNIQUE_BUNDLE_ENTRY = "%1$s_%2$s.properties";
public static String BUNDLE_DEF_LOCALES = "locales";
public static String BUNDLE_DEF_VERSION = "version";
public static String BUNDLE_DEF_ENCODING = "encoding";
public static String BUNDLES_FOR_LOCALE = "bundles.";
/** the name of the bundle. */
protected final String bundleName;
/** class loader to use (optional). */
protected ClassLoader loader;
/** i18n path where to seek for resources (optional). */
protected String i18nPath;
/** location of the definition file. */
protected URL definitionURL;
public static final String DEFAULT_I18N_PATH = "META-INF/";
public DefaultI18nInitializer(String bundleName) throws
NullPointerException {
this(bundleName, null, null);
}
public DefaultI18nInitializer(String bundleName,
ClassLoader loader) throws
NullPointerException {
this(bundleName, loader, null);
}
public DefaultI18nInitializer(String bundleName,
ClassLoader loader,
String i18nPath) throws NullPointerException {
if (bundleName == null) {
throw new NullPointerException(
"parameter 'bundleName' can not be null");
}
this.bundleName = bundleName;
this.loader = loader == null ? getClass().getClassLoader() : loader;
this.i18nPath = i18nPath == null ? DEFAULT_I18N_PATH : i18nPath;
}
public String getBundleName() {
return bundleName;
}
public ClassLoader getLoader() {
return loader;
}
public String getI18nPath() {
return i18nPath;
}
protected void setLoader(ClassLoader loader) {
this.loader = loader;
}
protected void setI18nPath(String i18nPath) {
this.i18nPath = i18nPath;
}
protected URL getResourceURL(String resource) {
if (log.isDebugEnabled()) {
log.debug(String.format("resource to seek : %s", resource));
}
return getLoader().getResource(resource);
}
public String resolvDefinition(Properties properties) throws Exception {
String filename = String.format(UNIQUE_BUNDLE_DEF, getBundleName());
URL url = getDefinitionURL();
if (log.isInfoEnabled()) {
log.info(String.format("definition file to seek : %s", url));
}
// load definition file
try (InputStream stream = url.openStream()) {
properties.load(stream);
stream.close();
}
// Load encoding from definition file and use it as Charset encoding
String encoding = properties.getProperty(BUNDLE_DEF_ENCODING);
Charset charset = Charset.forName(encoding);
if (log.isInfoEnabled()) {
log.info(String.format("Use encoding %s", charset));
}
setEncoding(charset);
String prefix = url.toString();
prefix = prefix.substring(0, prefix.length() - filename.length());
return prefix;
}
public URL getDefinitionURL() throws NullPointerException {
if (definitionURL == null) {
String filename = String.format(UNIQUE_BUNDLE_DEF, getBundleName());
String path = getI18nPath() + filename;
definitionURL = getResourceURL(path);
if (definitionURL == null) {
throw new NullPointerException(String.format("could not find bundle definition file at %s", path));
}
}
return definitionURL;
}
public URL[] resolvURLs(String prefixURL,
Properties definition) throws Exception {
// get locales from properties
String localesAsStr = definition.getProperty(BUNDLE_DEF_LOCALES);
Locale[] locales = I18nUtil.parseLocales(localesAsStr);
if (log.isDebugEnabled()) {
log.debug(String.format("Detected locales : %s", Arrays.toString(locales)));
}
List lUrls = new ArrayList<>(locales.length);
// for each locale found in definition file, add resource url
String bundleName = getBundleName();
for (Locale l : locales) {
String url = prefixURL + String.format(UNIQUE_BUNDLE_ENTRY, bundleName, l);
if (log.isInfoEnabled()) {
log.info(String.format("Detected resource for locale %s : %s", l, url));
}
URL u = new URL(url);
//FIXME on devrait tester que la resource est disponible ?
lUrls.add(u);
}
return lUrls.toArray(new URL[lUrls.size()]);
}
@Override
public I18nBundle[] resolvBundles() throws Exception {
Properties definition = new Properties();
String prefixURL = resolvDefinition(definition);
// detect bundles urls
URL[] urls = resolvURLs(prefixURL, definition);
// detect bundles
return resolvBundles(urls);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy