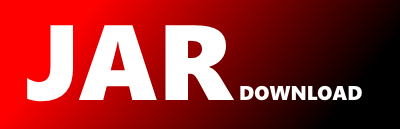
org.nuiton.i18n.spi.GetterFile Maven / Gradle / Ivy
The newest version!
package org.nuiton.i18n.spi;
/*-
* #%L
* I18n :: Api
* %%
* Copyright (C) 2004 - 2017 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.io.BufferedWriter;
import java.io.File;
import java.io.IOException;
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.Set;
import java.util.TreeSet;
import java.util.stream.Collectors;
/**
* Represents a getter file with all his detected keys.
* Created by tchemit on 29/10/17.
*
* @author Tony Chemit - [email protected]
*/
public class GetterFile {
private static final String GETTER_EXTENSION = ".getter";
private final File file;
private final String name;
private final Set keys;
public static List load(File gettersDirectory) throws IOException {
if (gettersDirectory.exists()) {
return Files
.walk(gettersDirectory.toPath(), 1)
.filter(p -> Files.isRegularFile(p) && p.toFile().getName().endsWith(GETTER_EXTENSION))
.map(Path::toFile)
.map(f -> new GetterFile(f.getParentFile(), f.getName()))
.collect(Collectors.toList());
} else {
return Collections.emptyList();
}
}
public GetterFile(File gettersDirectory, String name) {
this.file = new File(gettersDirectory, name);
this.name = name;
this.keys = new TreeSet<>();
}
public synchronized void addKey(String key) {
keys.add(key);
}
public synchronized void addKeys(Collection result) {
keys.addAll(result);
}
public void load() throws IOException {
if (file.exists()) {
List existingKeys = Files.readAllLines(file.toPath(), StandardCharsets.UTF_8);
keys.addAll(existingKeys);
}
}
public void store() throws IOException {
if (!file.getParentFile().exists()) {
Files.createDirectories(file.getParentFile().toPath());
}
try (BufferedWriter writer = Files.newBufferedWriter(file.toPath(), StandardCharsets.UTF_8)) {
for (String key : keys) {
writer.write(key);
writer.newLine();
}
}
}
public File getFile() {
return file;
}
public String getName() {
return name;
}
public Set getKeys() {
return keys;
}
public void delete() {
try {
Files.deleteIfExists(file.toPath());
} catch (IOException e) {
throw new IllegalStateException("Can't delete getter file " + file, e);
}
}
public boolean isNotEmpty() {
return !keys.isEmpty();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy