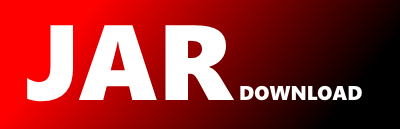
io.ultreia.java4all.i18n.runtime.I18nLanguage Maven / Gradle / Ivy
package io.ultreia.java4all.i18n.runtime;
/*-
* #%L
* I18n :: Runtime
* %%
* Copyright (C) 2018 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.java4all.i18n.spi.I18nResourceNotFoundException;
import io.ultreia.java4all.i18n.spi.I18nTemplateDefinition;
import io.ultreia.java4all.i18n.spi.I18nTranslationSetDefinition;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
import java.util.Properties;
/**
* Created by tchemit on 05/11/2018.
*
* @author Tony Chemit - [email protected]
*/
public class I18nLanguage {
private static final Logger log = LogManager.getLogger(I18nLanguage.class);
private final Locale locale;
private final I18nTranslationSetDefinition translationSetDefinition;
private final List dependenciesTranslationSetDefinition;
private final List templateDefinitions;
private final Properties translations;
private final Map templates;
/**
* Used to know null should be returned when a key is not found.
*/
private final boolean missingKeyReturnNull;
public I18nLanguage(Locale locale,
boolean missingKeyReturnNull,
I18nTranslationSetDefinition translationSetDefinition,
List dependenciesTranslationSetDefinition,
List templateDefinitions,
Properties translations,
Map templates) {
this.locale = Objects.requireNonNull(locale);
this.translationSetDefinition = translationSetDefinition;
this.dependenciesTranslationSetDefinition = dependenciesTranslationSetDefinition;
this.templateDefinitions = templateDefinitions;
this.translations = Objects.requireNonNull(translations);
this.templates = Objects.requireNonNull(templates);
this.missingKeyReturnNull = missingKeyReturnNull;
}
public Locale getLocale() {
return locale;
}
public Properties getTranslations() {
return translations;
}
public Map getTemplates() {
return templates;
}
public String getTemplate(String name) {
return templates.get(name);
}
/**
* Translate takes a sentence and returns its translation if found, the very
* same string otherwise.
*
* @param sentence sentence to translate
* @return translated sentence
*/
public String translate(String sentence) {
try {
String result = translations.getProperty(sentence);
// Empty String is also considered as missing
if (result == null || "".equals(result)) {
if (missingKeyReturnNull) {
result = null;
} else {
result = sentence;
}
}
return result;
} catch (Exception eee) {
if (log.isErrorEnabled()) {
log.error("Unexpected error while translating : ", eee);
}
return sentence;
}
}
public boolean hasRecord(String sentence) {
boolean result = false;
if (sentence != null) {
result = translations.containsKey(sentence);
// Empty String is considered as missing
String value = translations.getProperty(sentence);
result &= !"".equals(value);
}
return result;
}
public I18nTranslationSetDefinition getTranslationSetDefinition() {
return translationSetDefinition;
}
public List getTemplateDefinitions() {
return templateDefinitions;
}
public List getDependenciesTranslationSetDefinition() {
return dependenciesTranslationSetDefinition;
}
@Override
public String toString() {
return "I18nLanguage ';
}
public I18nTemplateDefinition getTemplateDefinition(String name) {
return templateDefinitions.stream().filter(t->name.equals(t.getName())).findFirst().orElseThrow(()-> new I18nResourceNotFoundException("Could not find template: "+name));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy