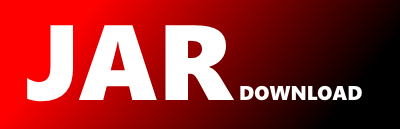
io.ultreia.java4all.i18n.runtime.boot.UserI18nBootLoader Maven / Gradle / Ivy
package io.ultreia.java4all.i18n.runtime.boot;
/*-
* #%L
* I18n :: Runtime
* %%
* Copyright (C) 2018 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.java4all.i18n.runtime.I18nConfiguration;
import io.ultreia.java4all.i18n.runtime.I18nLanguage;
import io.ultreia.java4all.i18n.runtime.I18nLanguageProvider;
import io.ultreia.java4all.i18n.spi.I18nResourceInitializationException;
import io.ultreia.java4all.i18n.spi.I18nTemplateDefinition;
import io.ultreia.java4all.i18n.spi.I18nTranslationSetDefinition;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Locale;
import java.util.Objects;
import java.util.Properties;
/**
* Created by tchemit on 06/11/2018.
*
* @author Tony Chemit - [email protected]
*/
public class UserI18nBootLoader implements I18nBootLoader {
/** Logger. */
private static final Logger log = LogManager.getLogger(UserI18nBootLoader.class);
/**
* User directory where to store the i18n resources.
*/
private final Path userDirectory;
/**
* Delegate boot loader to obtain default i18n resources to put in user directory.
*/
private final I18nBootLoader delegate;
public UserI18nBootLoader(Path userDirectory, I18nBootLoader delegate) {
this.userDirectory = userDirectory;
this.delegate = delegate;
}
@Override
public I18nConfiguration getConfiguration() {
return delegate.getConfiguration();
}
@Override
public I18nLanguageProvider init(Locale locale) {
boolean isNew = !Files.exists(userDirectory);
I18nLanguageProvider delegateLanguageProvider = Objects.requireNonNull(delegate).init(locale);
// creates the user directory and fill it with i18n resources
// coming from delegate language provider
try {
createUserI18nLayout(userDirectory, delegateLanguageProvider);
} catch (Exception e) {
throw new I18nInitializationException("Could not create user i18n directory", e);
}
I18nLanguageProvider provider;
try {
provider = I18nLanguageProvider.forDirectory(locale,
getConfiguration(),
userDirectory,
delegateLanguageProvider.getApplicationDefinition());
} catch (I18nResourceInitializationException e) {
throw new I18nInitializationException("Could not boot i18n", e);
}
if (!isNew) {
// will update directory if required
updateUserI18nLayout(userDirectory, provider);
}
return provider;
}
/**
* Creates the user i18n structure or fill it if required.
*
* will use the default initializer to obtain i18n resources from default
* system, then copy them to the user directory.
*
* @param directory the directory where to export i18n resources
* @param delegateLanguageProvider delegate language provider (from classpath)
* @throws Exception if any pb
*/
protected void createUserI18nLayout(Path directory, I18nLanguageProvider delegateLanguageProvider) throws Exception {
Path translationsPath = directory.resolve(I18nTranslationSetDefinition.PATH);
Path templatesPath = directory.resolve(I18nTemplateDefinition.PATH);
if (!Files.exists(translationsPath)) {
Files.createDirectories(translationsPath);
}
Charset encoding = delegateLanguageProvider.getConfiguration().getEncoding();
for (Locale locale : delegateLanguageProvider.getLocales()) {
I18nLanguage language = delegateLanguageProvider.getLanguage(locale);
I18nTranslationSetDefinition translationSetDefinition = language.getTranslationSetDefinition();
Properties translations = language.getTranslations();
I18nTranslationSetDefinition.write(translationSetDefinition, encoding, false, translationsPath, translations, false);
for (I18nTemplateDefinition templateDefinition : language.getTemplateDefinitions()) {
String template = language.getTemplate(templateDefinition.getName());
I18nTemplateDefinition.write(templateDefinition, templatesPath, encoding, false, template, false);
}
}
}
/**
* Hook to update the user i18n structure.
*
* If you wants to do something specific, overrides this method.
*
* @param directory the user directory where are i18n resources
* @param languageProvider user language provider (from directory)
*/
public void updateUserI18nLayout(Path directory, I18nLanguageProvider languageProvider) {
// by default nothing to do, change this if you wants something
}
}