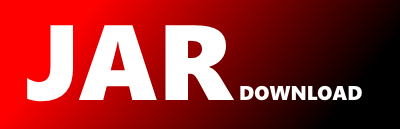
io.ultreia.java4all.i18n.spi.builder.I18nModule Maven / Gradle / Ivy
The newest version!
package io.ultreia.java4all.i18n.spi.builder;
/*-
* #%L
* I18n :: Spi Builder
* %%
* Copyright (C) 2018 - 2024 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.java4all.i18n.spi.I18nCoordinate;
import io.ultreia.java4all.i18n.spi.I18nKeySetDefinition;
import io.ultreia.java4all.i18n.spi.I18nModuleDefinition;
import io.ultreia.java4all.i18n.spi.I18nResourceInitializationException;
import io.ultreia.java4all.i18n.spi.I18nTemplateDefinition;
import io.ultreia.java4all.i18n.spi.I18nTranslationSetDefinition;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import java.io.IOException;
import java.nio.charset.Charset;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.Collections;
import java.util.LinkedList;
import java.util.List;
import java.util.Locale;
import java.util.Objects;
import java.util.Optional;
import java.util.Properties;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Created by tchemit on 29/10/2018.
*
* @author Tony Chemit - [email protected]
*/
public class I18nModule extends I18nCoordinate {
private static final Logger log = LogManager.getLogger(I18nModule.class);
/**
* Module configuration.
*/
private final I18nModuleConfiguration configuration;
/**
* Module getters.
*/
private final List moduleGetters;
/**
* Dependencies getters.
*/
private final List dependenciesGetters;
/**
* Module translations.
*/
private final List moduleTranslations;
/**
* Dependencies translations.
*/
private final List dependenciesTranslations;
/**
* Module templates.
*/
private final List moduleTemplates;
/**
* Dependencies templates.
*/
private final List dependenciesTemplates;
private I18nModule(I18nModuleConfiguration configuration,
List moduleGetters,
List dependenciesGetters,
List moduleTranslations,
List dependenciesTranslations,
List moduleTemplates,
List dependenciesTemplates) {
super(configuration);
this.configuration = configuration;
this.moduleGetters = moduleGetters == null ? null : new LinkedList<>(moduleGetters);
this.dependenciesGetters = dependenciesGetters;
this.moduleTranslations = moduleTranslations == null ? null : new LinkedList<>(moduleTranslations);
this.dependenciesTranslations = dependenciesTranslations;
this.moduleTemplates = moduleTemplates;
this.dependenciesTemplates = dependenciesTemplates;
}
public static Builder builder(Properties properties) throws I18nModuleNotInitializedException {
return new Builder(I18nModuleConfiguration.of(properties));
}
public static I18nModule forGetter(Properties properties) throws I18nResourceInitializationException, I18nModuleNotInitializedException {
return builder(properties).loadKeySets().build();
}
public I18nModuleConfiguration getConfiguration() {
return configuration;
}
public I18nKeySet getModuleKeySet(String name) throws I18nModuleNotInitializedException {
if (moduleGetters == null) {
throw new I18nModuleNotInitializedException("No getters configuration found.");
}
return getOrCreate(moduleGetters, name);
}
public boolean withModuleGetters() {
return moduleGetters != null && moduleGetters.size() > 0;
}
public boolean withDependenciesGetters() {
return dependenciesGetters != null && dependenciesGetters.size() > 0;
}
public boolean withModuleTranslations() {
return moduleTranslations != null && moduleTranslations.size() > 0;
}
public boolean withDependenciesTranslations() {
return dependenciesTranslations != null && dependenciesTranslations.size() > 0;
}
public boolean withModuleTemplates() {
return moduleTemplates != null && moduleTemplates.size() > 0;
}
public boolean withDependenciesTemplates() {
return dependenciesTemplates != null && dependenciesTemplates.size() > 0;
}
public List getModuleGetters() {
return moduleGetters;
}
public List getDependenciesGetters() {
return dependenciesGetters;
}
public List getModuleTranslations() {
return moduleTranslations;
}
public List getDependenciesTranslations() {
return dependenciesTranslations;
}
public List getModuleTemplates() {
return moduleTemplates;
}
public List getDependenciesTemplates() {
return dependenciesTemplates;
}
public I18nTranslationSet getModuleTranslation(Locale locale) {
return getOrCreate(moduleTranslations, locale);
}
public List getDependenciesTranslations(Locale locale) {
if (dependenciesTranslations == null) {
return Collections.emptyList();
}
return get(dependenciesTranslations, locale);
}
public Set getModuleKeys() {
return getKeys0(moduleGetters);
}
public Set getDependenciesKeys() {
return getKeys0(dependenciesGetters);
}
public void deleteModuleGetters() throws IOException {
if (withModuleGetters()) {
Path gettersDirectory = configuration.getGettersDirectory();
for (I18nKeySet moduleGetter : getModuleGetters()) {
String fileName = moduleGetter.getDefinition().getResourcePath(false);
Path target = gettersDirectory.resolve(fileName);
Files.deleteIfExists(target);
}
Files.deleteIfExists(gettersDirectory);
}
}
public void storeModuleKeySet(I18nKeySet i18nKeysFile) throws IOException {
I18nKeySetDefinition.write(i18nKeysFile.getDefinition(), configuration.getGettersDirectory(), configuration.getEncoding(), false, i18nKeysFile.getKeySet());
}
public void storeModuleTranslation(I18nTranslationSet i18nTranslationFile) throws IOException {
I18nTranslationSetDefinition.write(i18nTranslationFile.getDefinition(), configuration.getEncoding(), false, configuration.getTranslationsDirectory(), i18nTranslationFile.getTranslations(), true);
}
public Path exportModuleGetters(Path outputDir) throws IOException {
I18nKeySetDefinition definition = new I18nKeySetDefinition(this);
if (withModuleGetters()) {
Set moduleKeys = getModuleKeys();
return I18nKeySetDefinition.write(definition, outputDir, configuration.getEncoding(), true, moduleKeys);
}
return null;
}
public void exportModuleTranslation(I18nTranslationSetDefinition i18nTranslationFile, Path directory, Properties properties) throws IOException {
I18nTranslationSetDefinition.write(i18nTranslationFile, configuration.getEncoding(), true, directory, properties, true);
}
public void exportModuleTranslations(Path outputDir) throws IOException {
if (withModuleTranslations()) {
for (I18nTranslationSet moduleTranslation : getModuleTranslations()) {
I18nTranslationSetDefinition.write(moduleTranslation.getDefinition(), configuration.getEncoding(), true, outputDir, moduleTranslation.getTranslations(), true);
}
}
}
public void exportModuleTemplates(Path outputDir) throws IOException {
if (withModuleTemplates()) {
Charset encoding = configuration.getEncoding();
for (I18nTemplate moduleTemplate : getModuleTemplates()) {
I18nTemplateDefinition.write(moduleTemplate.getDefinition(), outputDir, encoding, true, moduleTemplate.getTemplate(), true);
}
}
}
public void exportDependenciesTemplates(Path outputDir) throws IOException {
if (withDependenciesTemplates()) {
Charset encoding = configuration.getEncoding();
for (I18nTemplate template : getDependenciesTemplates()) {
I18nTemplateDefinition.write(template.getDefinition(), outputDir, encoding, true, template.getTemplate(), true);
}
}
}
public boolean isEmpty() {
return !withModuleGetters()
&& !withModuleTranslations()
&& !withModuleTemplates()
&& !withDependenciesGetters()
&& !withDependenciesTranslations()
&& !withDependenciesTemplates();
}
private I18nKeySet getOrCreate(List files, String name) {
Optional first = files.stream().filter(e -> name.equals(e.getDefinition().getName())).findFirst();
return first.orElseGet(() -> {
I18nKeySet e1 = new I18nKeySet(new I18nKeySetDefinition(getPackageName(), name), Collections.emptySet());
files.add(e1);
return e1;
});
}
private List get(List files, Locale name) {
return files.stream().filter(e -> name.equals(e.getDefinition().getLocale())).collect(Collectors.toList());
}
private I18nTranslationSet getOrCreate(List files, Locale name) {
List first = get(files, name);
if (first.isEmpty()) {
I18nTranslationSet e1 = new I18nTranslationSet(new I18nTranslationSetDefinition(getPackageName(), getName(), name), new Properties());
files.add(e1);
return e1;
} else {
return first.get(0);
}
}
private Set getKeys0(List files) {
return files == null ? Collections.emptySet() : files.stream().flatMap(e -> e.getKeySet().stream()).collect(Collectors.toSet());
}
public static class Builder {
private final I18nModuleConfiguration configuration;
private boolean loadKeySets;
private boolean loadAtInit;
private boolean loadTranslations;
private boolean loadTemplates;
private ClassLoader classLoader;
private Builder(I18nModuleConfiguration configuration) {
this.configuration = Objects.requireNonNull(configuration);
}
Builder loadKeySets() {
return loadKeySets(configuration.isLoadAtInit());
}
public Builder loadKeySets(boolean loadAtInit) {
this.loadKeySets = true;
this.loadAtInit = loadAtInit;
return this;
}
public Builder loadTranslations() {
this.loadTranslations = true;
return this;
}
public Builder loadTemplates() {
this.loadTemplates = true;
return this;
}
public Builder loadDependencies(ClassLoader classLoader) {
this.classLoader = Objects.requireNonNull(classLoader);
return this;
}
public I18nModule build() throws I18nResourceInitializationException {
log.debug("Starts building i18n module.");
List moduleKeySets = null;
String packageName = configuration.getPackageName();
if (loadKeySets) {
moduleKeySets = I18nKeySet.detectKeySets(configuration.getGettersDirectory(), packageName, loadAtInit);
}
List moduleTranslations = null;
Charset encoding = configuration.getEncoding();
if (loadTranslations) {
moduleTranslations = I18nTranslationSet.detect(configuration.getTranslationsDirectory(), packageName, encoding);
}
List moduleTemplates = null;
if (loadTemplates) {
moduleTemplates = I18nTemplate.detect(configuration.getTemplatesDirectory(), packageName, configuration.getTemplateExtension(), encoding);
}
List dependenciesKeySets = null;
List dependenciesTranslations = null;
List dependenciesTemplates = null;
if (classLoader != null) {
List i18nModuleDefinitions = I18nModuleDefinition.detect(classLoader);
if (loadKeySets) {
dependenciesKeySets = I18nKeySet.detectKeySets(classLoader, i18nModuleDefinitions);
}
if (loadTranslations) {
dependenciesTranslations = I18nTranslationSet.detect(classLoader, encoding, i18nModuleDefinitions);
}
if (loadTemplates) {
dependenciesTemplates = I18nTemplate.detect(classLoader, encoding, i18nModuleDefinitions);
}
}
return new I18nModule(configuration, moduleKeySets, dependenciesKeySets, moduleTranslations, dependenciesTranslations, moduleTemplates, dependenciesTemplates);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy