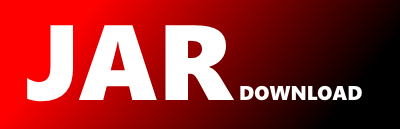
io.ultreia.java4all.i18n.spi.builder.I18nModuleConfiguration Maven / Gradle / Ivy
package io.ultreia.java4all.i18n.spi.builder;
/*-
* #%L
* I18n :: Spi Builder
* %%
* Copyright (C) 2018 - 2024 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.java4all.i18n.spi.I18nCoordinate;
import io.ultreia.java4all.i18n.spi.I18nLocaleHelper;
import java.nio.charset.Charset;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Locale;
import java.util.Objects;
import java.util.Optional;
import java.util.Properties;
import java.util.Set;
import java.util.stream.Collectors;
/**
* Place this contract on any mojo that use getter files.
*
* This is the new way to unify the mojo configuration.
*
* Created by tchemit on 23/10/2018.
*
* @author Tony Chemit - [email protected]
*/
public class I18nModuleConfiguration extends I18nCoordinate {
static final String I18N_MODULE_CONFIGURATION = "I18nModuleConfiguration";
/**
* Directory where to generate getters files.
*/
private final Path generatedGettersDirectory;
/**
* Directory where to load and store persisted getters files.
*/
private final Path persistedGettersDirectory;
/**
* Directory where to load and store translations files.
*/
private final Path translationsDirectory;
/**
* Directory where to load and store templates files.
*/
private final Path templatesDirectory;
/**
* Locales used by translations.
*/
private final Set locales;
/**
* Encoding used to load and write properties files.
*/
private final Charset encoding;
/**
* Is module getters are persisted?
*
* In that case the {@link #getPersistedGettersDirectory()} will be used instead of {@link #getGeneratedGettersDirectory()}}.
*/
private final boolean persistGetters;
/**
* Should we load getters keys at init?
*/
private final boolean loadAtInit;
private final String templateExtension;
public I18nModuleConfiguration(String packageName,
String name,
Path generatedGettersDirectory,
Path persistedGettersDirectory,
Path translationsDirectory,
Path templatesDirectory,
Set locales,
Charset encoding,
boolean persistGetters,
boolean loadAtInit, String templateExtension) {
super(packageName, name);
this.generatedGettersDirectory = generatedGettersDirectory;
this.persistedGettersDirectory = persistedGettersDirectory;
this.translationsDirectory = translationsDirectory;
this.templatesDirectory = templatesDirectory;
this.locales = locales;
this.encoding = encoding;
this.persistGetters = persistGetters;
this.loadAtInit = loadAtInit;
this.templateExtension = templateExtension;
}
public static void store(Properties properties, I18nModuleConfiguration configuration) {
Objects.requireNonNull(properties).put(I18N_MODULE_CONFIGURATION, Objects.requireNonNull(configuration).serialize());
}
public static void store(Properties properties, String configuration) {
Objects.requireNonNull(properties).put(I18N_MODULE_CONFIGURATION, Objects.requireNonNull(configuration));
}
public static I18nModuleConfiguration of(Properties properties) throws I18nModuleNotInitializedException {
String str = Objects.requireNonNull(properties).getProperty(I18N_MODULE_CONFIGURATION);
return Optional.ofNullable(str).map(I18nModuleConfiguration::deserialize).orElseThrow(() -> new I18nModuleNotInitializedException("Did not find i18n configuration, please add i18n:init goal in your maven build."));
}
private static I18nModuleConfiguration deserialize(String str) {
String[] split = str.split("\\$\\$");
return new I18nModuleConfiguration(
split[0],
split[1],
Paths.get(split[2]),
Paths.get(split[3]),
Paths.get(split[4]),
Paths.get(split[5]),
I18nLocaleHelper.parseLocalesAsSet(split[6]),
Charset.forName(split[7]),
Boolean.parseBoolean(split[8]),
Boolean.parseBoolean(split[9]),
split[10]);
}
public Path getGeneratedGettersDirectory() {
return generatedGettersDirectory;
}
public Path getPersistedGettersDirectory() {
return persistedGettersDirectory;
}
public Path getTranslationsDirectory() {
return translationsDirectory;
}
public Path getTemplatesDirectory() {
return templatesDirectory;
}
public boolean isPersistGetters() {
return persistGetters;
}
public boolean isLoadAtInit() {
return loadAtInit;
}
public Path getGettersDirectory() {
return isPersistGetters() ? getPersistedGettersDirectory() : getGeneratedGettersDirectory();
}
public Charset getEncoding() {
return encoding;
}
public Set getLocales() {
return locales;
}
public String getTemplateExtension() {
return templateExtension;
}
@Override
public String toString() {
return "I18nModuleConfiguration{" +
"id=" + getId() +
", gettersDirectory=" + getGettersDirectory() +
", translationsDirectory=" + translationsDirectory +
", templatesDirectory=" + templatesDirectory +
", templateExtension=" + templateExtension+
", locales=" + locales +
", encoding=" + encoding +
", persistGetters=" + persistGetters +
", loadAtInit=" + loadAtInit +
'}';
}
public String serialize() {
return getPackageName()
+ "$$" + getName()
+ "$$" + getGeneratedGettersDirectory().toString()
+ "$$" + getPersistedGettersDirectory().toString()
+ "$$" + getTranslationsDirectory().toString()
+ "$$" + getTemplatesDirectory().toString()
+ "$$" + getLocales().stream().map(l -> l.getLanguage() + "_" + l.getCountry()).collect(Collectors.joining(","))
+ "$$" + getEncoding().name()
+ "$$" + isPersistGetters()
+ "$$" + isLoadAtInit()
+ "$$" + getTemplateExtension();
}
}