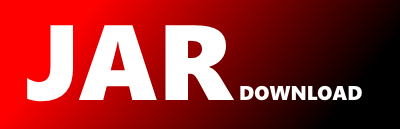
org.nuiton.jaxx.demo.component.jaxx.StatusMessagePanelDemo Maven / Gradle / Ivy
package org.nuiton.jaxx.demo.component.jaxx;
/*-
* #%L
* JAXX :: Demo
* %%
* Copyright (C) 2008 - 2020 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.awt.BorderLayout;
import java.awt.GridLayout;
import java.awt.LayoutManager;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.JButton;
import javax.swing.JPanel;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.nuiton.jaxx.demo.DemoPanel;
import org.nuiton.jaxx.runtime.JAXXContext;
import org.nuiton.jaxx.runtime.JAXXObjectDescriptor;
import org.nuiton.jaxx.runtime.JAXXUtil;
import org.nuiton.jaxx.widgets.status.StatusMessagePanel;
import static io.ultreia.java4all.i18n.I18n.t;
public class StatusMessagePanelDemo extends DemoPanel {
/*-----------------------------------------------------------------------*/
/*------------------------- Other static fields -------------------------*/
/*-----------------------------------------------------------------------*/
private static final String $jaxxObjectDescriptor = "H4sIAAAAAAAAAJ2UvW4TQRDH50zsxPmAxJGoKCxiEiTCHm5xFD4SRWA5BJEmwg1r38q56Hy37M7hS5OCgo4XoKClQXmHiAqJhjbvgJRHYPbubOfjnARc7Flz//3tf2Zn7vsfyGsFy4HqMD90MfDZHo8ipkIf3a5g9Wc7O1utPdHGdaHbypUYKEh+Vg5yTZhyBnGNwJoNItkJyTYkOyXZa0FXBr7wT4FqDZjUuO8JvSsEItwfubmttb09UNYiGar0rEzXWWf9+lQ9tsT6lxxAJMm+SfvBPwCGWY81IOc6CKXGHv/AbY/7HTKnXL9DCc2Y2JrHtX7Fu+I9HMB4AwqSK4IhPPyv8sS4GBVJhKnKuugGr7kvvEcIj8+n4NBL1u7Tktg2cgz1ptCad0S80yCkjKEFhIlKvc8rGf8R0z1KhyVRIyoOtMVK/XmIdByJ58+Ik7CRTV9UV01g7uxLi7Kpnvffc52OQM107DnDempHweKo26NuYcNuGV6c1YS8CimMsDC6UU2vvSFV0mULo7vMHBMLPx4eqG+9k+N+a42RufJVu05NEd2zVIEUCl3j7VbSVyG6nr3JZa0JRS08GsF4xJYud76dKsk9uZgzJGZI7AXXu0TLjx8f/bj97vcNyG3ApBdwZ4Mb/Uso4q6iigWeE8knT2Nz070JWmeNTYSCx/cDuku4ueJw5OWW6zt076sRVWnp8ioNTB1unfz8+vneUb9SFnmsXGfnsFr5t1Bwfc/1RTyG6YRljt2U1CJ0guH4ZA2UZZ6TMh2GO/Favpi/Cd81y0IUmkcldm/+LcbvZq4kIIyhiKh+pZXEf5kjfTVaIYrVbObstVwxs9jZhHki/AVMNoCg4wUAAA==";
private static final Logger log = LogManager.getLogger(StatusMessagePanelDemo.class);
private static final long serialVersionUID = 1L;
/*-----------------------------------------------------------------------*/
/*------------------------ Protected components ------------------------*/
/*-----------------------------------------------------------------------*/
protected StatusMessagePanel p;
/*-----------------------------------------------------------------------*/
/*------------------------- Private components -------------------------*/
/*-----------------------------------------------------------------------*/
private StatusMessagePanelDemo $DemoPanel0;
private JButton $JButton0;
private JButton $JButton1;
private JPanel $JPanel0;
/*-----------------------------------------------------------------------*/
/*---------------------------- Constructors ----------------------------*/
/*-----------------------------------------------------------------------*/
public StatusMessagePanelDemo(LayoutManager param0, boolean param1) {
super(param0 ,param1);
}
public StatusMessagePanelDemo(JAXXContext param0, LayoutManager param1, boolean param2) {
super(param0 ,param1 ,param2);
}
public StatusMessagePanelDemo(LayoutManager param0) {
super(param0);
}
public StatusMessagePanelDemo(JAXXContext param0, LayoutManager param1) {
super(param0 ,param1);
}
public StatusMessagePanelDemo() {
}
public StatusMessagePanelDemo(JAXXContext param0) {
super(param0);
}
public StatusMessagePanelDemo(boolean param0) {
super(param0);
}
public StatusMessagePanelDemo(JAXXContext param0, boolean param1) {
super(param0 ,param1);
}
/*-----------------------------------------------------------------------*/
/*--------------------------- Statics methods ---------------------------*/
/*-----------------------------------------------------------------------*/
public static JAXXObjectDescriptor $getJAXXObjectDescriptor() {
return JAXXUtil.decodeCompressedJAXXObjectDescriptor($jaxxObjectDescriptor);
}
/*-----------------------------------------------------------------------*/
/*---------------------------- Event methods ----------------------------*/
/*-----------------------------------------------------------------------*/
public void doActionPerformed__on__$JButton0(ActionEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
p.setStatus(((JButton)event.getSource()).getText() + " ? shame on you!");
}
public void doActionPerformed__on__$JButton1(ActionEvent event) {
if (log.isDebugEnabled()) {
log.debug(event);
}
p.setStatus(((JButton)event.getSource()).getText() + " ? shame on ...");
}
/*-----------------------------------------------------------------------*/
/*----------------------- Public acessor methods -----------------------*/
/*-----------------------------------------------------------------------*/
public StatusMessagePanel getP() {
return p;
}
/*-----------------------------------------------------------------------*/
/*--------------------- Protected acessors methods ---------------------*/
/*-----------------------------------------------------------------------*/
protected JButton get$JButton0() {
return $JButton0;
}
protected JButton get$JButton1() {
return $JButton1;
}
protected JPanel get$JPanel0() {
return $JPanel0;
}
/*-----------------------------------------------------------------------*/
/*--------------------- Components creation methods ---------------------*/
/*-----------------------------------------------------------------------*/
protected void createP() {
$objectMap.put("p", p = new StatusMessagePanel());
p.setName("p");
}
/*-----------------------------------------------------------------------*/
/*------------------------ Internal jaxx methods ------------------------*/
/*-----------------------------------------------------------------------*/
@Override
protected void $initialize() {
if (log.isDebugEnabled()) {
log.debug(this);
}
$DemoPanel0 = this;
super.$initialize();
}
@Override
protected void $initialize_01_createComponents() {
if (log.isDebugEnabled()) {
log.debug(this);
}
super.$initialize_01_createComponents();
$objectMap.put("$DemoPanel0", $DemoPanel0);
// inline creation of $JPanel0
$objectMap.put("$JPanel0", $JPanel0 = new JPanel());
$JPanel0.setName("$JPanel0");
$JPanel0.setLayout(new GridLayout(0,1));
// inline creation of $JButton0
$objectMap.put("$JButton0", $JButton0 = new JButton());
$JButton0.setName("$JButton0");
$JButton0.setText(t("Fool me once"));
$JButton0.addActionListener(JAXXUtil.getEventListener(ActionListener.class, "actionPerformed", this, "doActionPerformed__on__$JButton0"));
// inline creation of $JButton1
$objectMap.put("$JButton1", $JButton1 = new JButton());
$JButton1.setName("$JButton1");
$JButton1.setText(t("Fool me twice"));
$JButton1.addActionListener(JAXXUtil.getEventListener(ActionListener.class, "actionPerformed", this, "doActionPerformed__on__$JButton1"));
createP();
// inline creation of $DemoPanel0
setName("$DemoPanel0");
setLayout(new BorderLayout());
}
@Override
protected void $initialize_02_registerDataBindings() {
if (log.isDebugEnabled()) {
log.debug(this);
}
super.$initialize_02_registerDataBindings();
// register 0 data bindings
}
@Override
protected void $initialize_03_finalizeCreateComponents() {
if (log.isDebugEnabled()) {
log.debug(this);
}
super.$initialize_03_finalizeCreateComponents();
// inline complete setup of $DemoPanel0
add($JPanel0, BorderLayout.CENTER);
add(p, BorderLayout.SOUTH);
// inline complete setup of $JPanel0
$JPanel0.add($JButton0);
$JPanel0.add($JButton1);
}
@Override
protected void $initialize_04_applyDataBindings() {
if (log.isDebugEnabled()) {
log.debug(this);
}
super.$initialize_04_applyDataBindings();
}
@Override
protected void $initialize_05_setProperties() {
if (log.isDebugEnabled()) {
log.debug(this);
}
super.$initialize_05_setProperties();
}
@Override
protected void $initialize_06_finalizeInitialize() {
if (log.isDebugEnabled()) {
log.debug(this);
}
super.$initialize_06_finalizeInitialize();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy