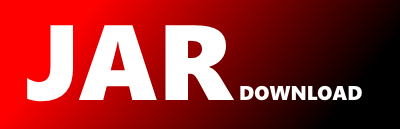
org.nuiton.jaxx.widgets.select.BeanDoubleListModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxx-widgets-select Show documentation
Show all versions of jaxx-widgets-select Show documentation
Widgets to select data (using combobox, jlist, doublelist...)
The newest version!
package org.nuiton.jaxx.widgets.select;
/*
* #%L
* JAXX :: Widgets Select
* %%
* Copyright (C) 2008 - 2020 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import org.jdesktop.beans.AbstractSerializableBean;
import org.nuiton.jaxx.runtime.swing.model.JaxxDefaultListModel;
import org.nuiton.jaxx.runtime.swing.model.JaxxFilterableListModel;
import javax.swing.ListModel;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.function.Predicate;
/**
* The model of the {@link BeanDoubleList} widget
*
* @param the type of the object in the list
* @author Kevin Morin - [email protected]
* @see BeanDoubleList
* @since 2.5.8
*/
public class BeanDoubleListModel extends AbstractSerializableBean {
public static final String PROPERTY_ADD_ENABLED = "addEnabled";
public static final String PROPERTY_REMOVE_ENABLED = "removeEnabled";
public static final String PROPERTY_SELECTED_UP_ENABLED = "selectedUpEnabled";
public static final String PROPERTY_SELECTED_DOWN_ENABLED = "selectedDownEnabled";
public static final String PROPERTY_USE_MULTI_SELECT = "useMultiSelect";
private static final long serialVersionUID = 1L;
/** List of all the available items */
protected List universe = new ArrayList<>();
/** Model containing the remaining available items */
protected final JaxxFilterableListModel universeModel = new JaxxFilterableListModel<>();
/** List of the selected items */
protected final List selected = new ArrayList<>();
/** Model containing the selected items */
protected final JaxxDefaultListModel selectedModel = new JaxxDefaultListModel<>();
protected boolean addEnabled;
protected boolean removeEnabled;
protected boolean selectedUpEnabled;
protected boolean selectedDownEnabled;
protected boolean useMultiSelect;
protected final Collection>> canRemoveItemsPredicates = new ArrayList<>();
/**
* To get the selected items.
*
* @return a list of O
*/
public List getSelected() {
return selected;
}
/**
* Sets the list of selected items.
* It fills the model of the list of the selected items with these items
* and removes them from the model of list of the universe.
*
* @param selected a list of O
*/
public void setSelected(List selected) {
//reset all the universe in the universe list
resetUniverse();
if (selected == null) {
selected = new ArrayList<>();
}
this.selected.clear();
selectedModel.clear();
addToSelected(selected);
}
/**
* To get all the available items.
*
* @return a list of O
*/
public List getUniverse() {
return universe;
}
/**
* Sets the list of the available items.
* It fills the model of the universe list with these items.
*
* @param universe a list of O
*/
public void setUniverse(List universe) {
if (universe == null) {
this.universe = new ArrayList<>();
} else {
this.universe = new ArrayList<>(universe);
}
resetUniverse();
}
protected void resetUniverse() {
universeModel.setAllElements(universe);
}
public ListModel getSelectedModel() {
return selectedModel;
}
public JaxxFilterableListModel getUniverseModel() {
return universeModel;
}
/**
* Adds an item to the selected items.
*
* @param item the item to select
*/
public void addToSelected(O item) {
selected.add(item);
selectedModel.addElement(item);
if (!useMultiSelect) {
universeModel.removeElement(item);
}
}
/**
* Adds a list of items to the selected items.
*
* @param items the list of the items to select
*/
public void addToSelected(List items) {
selected.addAll(items);
for (O item : items) {
selectedModel.setValueIsAdjusting(true);
universeModel.setValueIsAdjusting(true);
try {
selectedModel.addElement(item);
if (!useMultiSelect) {
universeModel.removeElement(item);
}
} finally {
selectedModel.setValueIsAdjusting(false);
universeModel.setValueIsAdjusting(false);
}
}
selectedModel.refresh();
universeModel.refresh();
}
/**
* Removes an item from the selected items.
*
* @param item the item to unselect
*/
public void removeFromSelected(O item) {
selected.remove(item);
selectedModel.removeElement(item);
if (!useMultiSelect) {
addToUniverseList(item);
}
}
/**
* Removes a list of items from the list of selected items.
*
* @param items the list of the items to unselect
*/
public void removeFromSelected(List items) {
selected.removeAll(items);
for (O item : items) {
selectedModel.removeElement(item);
if (!useMultiSelect) {
addToUniverseList(item);
}
}
}
/**
* Move up a selected item.
*
* @param item the selected item
* @since 2.5.26
*/
public void moveUpSelected(O item) {
int i = selected.indexOf(item);
selected.remove(item);
selectedModel.removeElement(item);
selected.add(i - 1, item);
selectedModel.insertElementAt(item, i - 1);
}
/**
* Move down a selected item.
*
* @param item the selected item
* @since 2.5.26
*/
public void moveDownSelected(O item) {
int i = selected.indexOf(item);
selected.remove(item);
selectedModel.removeElement(item);
selected.add(i + 1, item);
selectedModel.insertElementAt(item, i + 1);
}
public boolean isAddEnabled() {
return addEnabled;
}
public void setAddEnabled(boolean addEnabled) {
boolean oldValue = isAddEnabled();
this.addEnabled = addEnabled;
firePropertyChange(PROPERTY_ADD_ENABLED, oldValue, addEnabled);
}
public boolean isRemoveEnabled() {
return removeEnabled;
}
public void setRemoveEnabled(boolean removeEnabled) {
boolean oldValue = isRemoveEnabled();
this.removeEnabled = removeEnabled;
firePropertyChange(PROPERTY_REMOVE_ENABLED, oldValue, removeEnabled);
}
public boolean isSelectedUpEnabled() {
return selectedUpEnabled;
}
public void setSelectedUpEnabled(boolean selectedUpEnabled) {
boolean oldValue = isSelectedUpEnabled();
this.selectedUpEnabled = selectedUpEnabled;
firePropertyChange(PROPERTY_SELECTED_UP_ENABLED, oldValue, selectedUpEnabled);
}
public boolean isSelectedDownEnabled() {
return selectedDownEnabled;
}
public void setSelectedDownEnabled(boolean selectedDownEnabled) {
boolean oldValue = isSelectedDownEnabled();
this.selectedDownEnabled = selectedDownEnabled;
firePropertyChange(PROPERTY_SELECTED_DOWN_ENABLED, oldValue, selectedDownEnabled);
}
public boolean isUseMultiSelect() {
return useMultiSelect;
}
public void setUseMultiSelect(boolean useMultiSelect) {
boolean oldValue = isUseMultiSelect();
this.useMultiSelect = useMultiSelect;
firePropertyChange(PROPERTY_USE_MULTI_SELECT, oldValue, useMultiSelect);
}
/**
* Adds an item to the available items list at the right index
* to keep always the same order.
*
* @param item the item to add to the universe list
*/
protected void addToUniverseList(O item) {
// the maximum index where we should insert the item is its index
// in the list of all the available items
int index = universe.indexOf(item);
if (index != -1) {
// if the index is upper than the size of the list of the remaining available items,
// get the size of this list
int insertionIndex = Math.min(index, universeModel.getSize());
// we decrease the index to insert until we meet an item whose index
// in the list of all the available index is lower than the one of the item
// we want to insert
while (insertionIndex > 0) {
O o = universeModel.get(--insertionIndex);
int oIndex = universe.indexOf(o);
if (oIndex < index) {
insertionIndex++;
break;
}
}
universeModel.add(insertionIndex, item);
}
}
public int getSelectedListSize() {
return selected.size();
}
public void addCanRemoveItemsPredicate(java.util.function.Predicate> canRemoveItemsPredicate) {
canRemoveItemsPredicates.add(canRemoveItemsPredicate);
}
public void removeCanRemoveItemsPredicate(Predicate> canRemoveItemsPredicate) {
canRemoveItemsPredicates.remove(canRemoveItemsPredicate);
}
public boolean computeRemoveEnabled(List toRemoveItems) {
boolean result = true;
Iterator>> iterator = canRemoveItemsPredicates.iterator();
while (result && iterator.hasNext()) {
result = iterator.next().test(toRemoveItems);
}
setRemoveEnabled(result);
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy