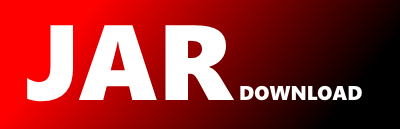
io.ultreia.java4all.jaxx.widgets.combobox.BeanEnumEditor Maven / Gradle / Ivy
package io.ultreia.java4all.jaxx.widgets.combobox;
/*-
* #%L
* JAXX :: Widgets
* %%
* Copyright (C) 2008 - 2024 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.collect.ImmutableMap;
import io.ultreia.java4all.bean.JavaBean;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import org.nuiton.jaxx.runtime.bean.BeanScopeAware;
import org.nuiton.jaxx.runtime.swing.editor.EnumEditor;
import org.nuiton.jaxx.runtime.swing.renderer.EnumEditorRenderer;
import java.util.EnumSet;
import java.util.function.Function;
/**
* Created on 01/30/19.
*
* @author Tony Chemit - [email protected]
* @since 8.0
*/
public class BeanEnumEditor> extends EnumEditor implements BeanScopeAware {
private static final Logger log = LogManager.getLogger(BeanEnumEditor.class);
final boolean useIndex;
private final BeanEnumEditorHandler handler;
private String property;
private JavaBean bean;
public BeanEnumEditor(Class type) {
this(type, false);
}
public BeanEnumEditor(Class type, boolean useIndex) {
super(type);
this.useIndex = useIndex;
this.handler = new BeanEnumEditorHandler<>();
}
public BeanEnumEditor(Class type, int maxOrdinal) {
this(type, maxOrdinal, false);
}
public BeanEnumEditor(Class type, int maxOrdinal, boolean useIndex) {
super(type, maxOrdinal);
this.useIndex = useIndex;
this.handler = new BeanEnumEditorHandler<>();
}
public void init(Function labelFunction) {
handler.init(this);
ImmutableMap.Builder labelsBuilder = ImmutableMap.builder();
for (B e : EnumSet.allOf(getType())) {
String label = labelFunction.apply(e);
labelsBuilder.put(e, label);
}
setRenderer(new EnumEditorRenderer<>(labelsBuilder.build()));
}
public String getProperty() {
return property;
}
public void setProperty(String property) {
handler.checkNotInit();
log.debug(String.format("%s - set property: %s", getName(), property));
this.property = property;
}
@Override
public Object getBean() {
return bean;
}
@Override
public void setBean(Object bean) {
handler.checkNotInit();
log.debug(String.format("%s - set bean: %s", getName(), property));
this.bean = (JavaBean) bean;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy