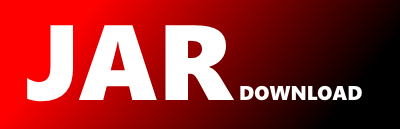
io.ultreia.java4all.jaxx.widgets.combobox.JaxxComboBoxCellEditor Maven / Gradle / Ivy
package io.ultreia.java4all.jaxx.widgets.combobox;
/*-
* #%L
* JAXX :: Widgets
* %%
* Copyright (C) 2008 - 2024 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.java4all.bean.JavaBean;
import javax.swing.DefaultCellEditor;
import javax.swing.JComboBox;
import javax.swing.JComponent;
import javax.swing.SwingUtilities;
import javax.swing.event.AncestorEvent;
import javax.swing.event.AncestorListener;
import javax.swing.table.TableCellEditor;
/**
* Created by tchemit on 20/11/2018.
*
* @author Tony Chemit - [email protected]
*/
public class JaxxComboBoxCellEditor extends DefaultCellEditor implements TableCellEditor, AncestorListener {
@SuppressWarnings("rawtypes")
public JaxxComboBoxCellEditor(JaxxComboBox editor) {
super(editor.getCombobox());
this.editorComponent = editor;
JComboBox comboBox = editor.getCombobox();
editorComponent.setOpaque(false);
comboBox.removeActionListener(this.delegate);
this.delegate = new EditorDelegate() {
@SuppressWarnings("unchecked")
@Override
public void setValue(Object value) {
if (value != null && !(value instanceof JavaBean)) {
value = null;
}
editor.setSelectedItem((O) value);
}
@Override
public Object getCellEditorValue() {
return editor.getModel().getSelectedItem();
}
@Override
public boolean stopCellEditing() {
if (comboBox.isEditable()) {
Object cellEditorValue = getCellEditorValue();
Object item = comboBox.getEditor().getItem();
if (item != null && !editor.getConfig().getBeanType().isAssignableFrom(item.getClass())) {
if (cellEditorValue == null && !"".equals(item)) {
// reset editor selected value, otherwise after there is a action performed (BasicComboBoxUI#1901)
// this will perform in JComboBox#1320 fires a selectedItem on this value
// and in JaxxComboBoxEditor#267 use this a the new value
editor.setSelectedItem(null);
}
}
}
return super.stopCellEditing();
}
};
((JComponent) comboBox.getEditor().getEditorComponent()).addAncestorListener(this);
comboBox.addActionListener(this.delegate);
}
@Override
public void ancestorAdded(AncestorEvent event) {
SwingUtilities.invokeLater(() -> {
editorComponent.requestFocusInWindow();
((JaxxComboBox>) editorComponent).getCombobox().getEditor().selectAll();
});
}
@Override
public void ancestorRemoved(AncestorEvent event) {
}
@Override
public void ancestorMoved(AncestorEvent event) {
}
@Override
public JaxxComboBox> getComponent() {
return (JaxxComboBox>) super.getComponent();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy