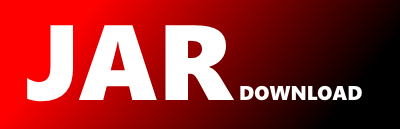
io.ultreia.java4all.jaxx.widgets.combobox.JaxxComboBoxModel Maven / Gradle / Ivy
package io.ultreia.java4all.jaxx.widgets.combobox;
/*-
* #%L
* JAXX :: Widgets
* %%
* Copyright (C) 2008 - 2024 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import io.ultreia.java4all.bean.AbstractJavaBean;
import io.ultreia.java4all.bean.JavaBean;
import io.ultreia.java4all.decoration.Decorated;
import io.ultreia.java4all.decoration.Decorator;
import io.ultreia.java4all.lang.Setters;
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
import io.ultreia.java4all.jaxx.widgets.BeanUIUtil;
import java.lang.reflect.Method;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
//@GenerateJavaBeanDefinition
public class JaxxComboBoxModel extends AbstractJavaBean {
public static final String PROPERTY_BEAN = "bean";
public static final String PROPERTY_DATA = "data";
public static final String PROPERTY_INDEX = "index";
public static final String PROPERTY_REVERSE_SORT = "reverseSort";
public static final String PROPERTY_SELECTED_ITEM = "selectedItem";
private static final Logger log = LogManager.getLogger(JaxxComboBoxModel.class);
private final JaxxComboBoxConfig config;
/**
* selectedItem property
*/
O selectedItem;
/**
* flag to reverse the sort
*/
private Boolean reverseSort = false;
/**
* bean property
*/
private Object bean;
/**
* data of the combo-box
*/
private List data;
/**
* sort index property
*/
private int index = 0;
/**
* Mutator method on the property of boxed bean in the ui
*/
private Method mutator;
public JaxxComboBoxModel(JaxxComboBoxConfig config) {
this.config = config;
}
public Boolean getReverseSort() {
return reverseSort;
}
public void setReverseSort(Boolean reverseSort) {
Boolean oldValue = getReverseSort();
this.reverseSort = Objects.equals(true, reverseSort);
firePropertyChange(PROPERTY_REVERSE_SORT, oldValue, this.reverseSort);
}
public Object getBean() {
return bean;
}
public void setBean(Object bean) {
Object oldValue = getBean();
this.bean = bean;
firePropertyChange(PROPERTY_BEAN, oldValue, bean);
}
public List getData() {
return data;
}
public void setData(List data) {
List oldValue = getData();
this.data = data;
if (data != null && config.isBeanDecoratorAware()) {
Decorator decorator = config.getDecorator();
for (O datum : data) {
((Decorated) datum).registerDecorator(decorator);
}
}
firePropertyChange(PROPERTY_DATA, oldValue, data);
}
public O getSelectedItem() {
return selectedItem;
}
public void setSelectedItem(O selectedItem) {
O oldValue = getSelectedItem();
this.selectedItem = selectedItem;
if (config.isBeanDecoratorAware() && selectedItem instanceof Decorated) {
Decorated item = (Decorated) selectedItem;
if (item.decorator().isEmpty()) {
item.registerDecorator(config.getDecorator());
}
}
firePropertyChange("selectedItem", oldValue, selectedItem);
}
public int getIndex() {
return index;
}
public void setIndex(int index) {
int oldValue = getIndex();
this.index = index;
firePropertyChange(PROPERTY_INDEX, oldValue, index);
}
public JaxxComboBoxConfig getConfig() {
return config;
}
/**
* @return {@code true} if there is no data in comboBox, {@code false} otherwise.
*/
public boolean isEmpty() {
return getData() == null || getData().isEmpty();
}
/**
* Add the given items into the comboBox.
*
* Note: The item will be inserted at his correct following the selected ordering.
*
* @param items items to add in comboBox.
*/
public void addItems(Iterable items) {
List data = getData();
boolean wasEmpty = data.isEmpty();
boolean beanDecoratorAware = config.isBeanDecoratorAware();
Decorator decorator = config.getDecorator();
for (O item : items) {
data.add(item);
if (beanDecoratorAware) {
((Decorated) item).registerDecorator(decorator);
}
}
firePropertyChange(PROPERTY_DATA, null, getData());
fireEmpty(wasEmpty);
}
/**
* Remove the given items from the comboBox model.
*
* Note: If this item was selected, then selection will be cleared.
*
* @param items items to remove from the comboBox model
*/
public void removeItems(Iterable items) {
List data = getData();
boolean needUpdate = false;
for (O item : items) {
boolean remove = data.remove(item);
if (remove) {
// item was found in data
Object selectedItem = getSelectedItem();
if (item == selectedItem) {
// item was selected item, reset selected item then
setSelectedItem(null);
}
needUpdate = true;
}
}
if (needUpdate) {
firePropertyChange(PROPERTY_DATA, null, getData());
fireEmpty(false);
}
}
/**
* Add the given item into the comboBox.
*
* Note: The item will be inserted at his correct following the selected ordering.
*
* @param item item to add in comboBox.
*/
public void addItem(O item) {
addItems(Collections.singleton(item));
}
/**
* Remove the given item from the comboBox model.
*
* Note: If this item was selected, then selection will be cleared.
*
* @param item the item to remove from the comboBox model
*/
public void removeItem(O item) {
removeItems(Collections.singleton(item));
}
void fireEmpty(boolean wasEmpty) {
firePropertyChange(JaxxComboBox.PROPERTY_EMPTY, wasEmpty, isEmpty());
}
void mutateBeanProperty(O newValue) {
Object bean = getBean();
if (mutator == null && bean != null && config.getProperty() != null) {
mutator = Setters.getMutator(bean, config.getProperty());
}
log.info(String.format("%s → set new value: %s", config.getProperty(), newValue));
BeanUIUtil.invokeMethod(mutator, bean, newValue);
}
}