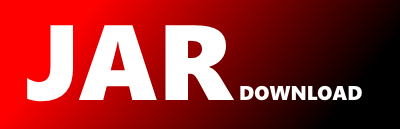
org.nuiton.jaxx.widgets.datetime.UnlimitedTimeEditorModel Maven / Gradle / Ivy
package org.nuiton.jaxx.widgets.datetime;
/*
* #%L
* JAXX :: Widgets
* %%
* Copyright (C) 2008 - 2024 Code Lutin, Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.base.Preconditions;
import io.ultreia.java4all.bean.AbstractJavaBean;
import org.nuiton.jaxx.widgets.ModelToBean;
import java.util.function.Predicate;
/**
* Created on 11/30/14.
*
* @author Tony Chemit - [email protected]
* @since 2.18
*/
public class UnlimitedTimeEditorModel extends AbstractJavaBean implements ModelToBean {
public static final String PROPERTY_TIME = "time";
public static final String PROPERTY_HOURS = "hours";
public static final String PROPERTY_MINUTES = "minutes";
public static final String PROPERTY_VALUE_IS_ADJUSTING = "valueIsAdjusting";
/**
* State to be able to custom the model. will be pass to {@code false} by the {@code DateTimeEditorHandler#init(DateTimeEditor)}.
*/
protected final boolean fillState = true;
/**
* Optional bean where to push back dates.
*/
protected Object bean;
/**
* Optional bean property where to push back the time date.
*/
protected String propertyTime;
/**
* Time in minutes.
*/
protected Integer time = null;
/**
* To stop propagate events when we are doing some modifications on the model.
*/
protected boolean valueIsAdjusting;
public String getPropertyTime() {
return propertyTime;
}
public void setPropertyTime(String propertyTime) {
Preconditions.checkState(fillState, "cant change *propertyTimeDate* property once the fillState is off.");
this.propertyTime = propertyTime;
}
@Override
public Object getBean() {
return bean;
}
public void setBean(Object bean) {
Preconditions.checkState(fillState, "cant change *bean* property once the fillState is off.");
this.bean = bean;
}
public Integer getTime() {
return time;
}
public void setTime(Integer time) {
if (!isValueIsAdjusting()) {
Integer oldHours = getHours();
Integer oldMinutes = getMinutes();
setTime(getHours(time), getMinutes(time));
firePropertyChange(PROPERTY_HOURS, oldHours, getHours());
firePropertyChange(PROPERTY_MINUTES, oldMinutes, getMinutes());
}
}
public int getHours() {
return getHours(time);
}
public void setHours(Integer hour) {
if (!isValueIsAdjusting()) {
Integer oldValue = getHours();
setTime(hour, getMinutes());
firePropertyChange(PROPERTY_HOURS, oldValue, hour);
}
}
public int getMinutes() {
return getMinutes(time);
}
public void setMinutes(Integer minutes) {
if (!isValueIsAdjusting()) {
Integer oldValue = getMinutes();
setTime(getHours(), minutes);
firePropertyChange(PROPERTY_MINUTES, oldValue, minutes);
}
}
public boolean isValueIsAdjusting() {
return valueIsAdjusting;
}
public void setValueIsAdjusting(boolean valueIsAdjusting) {
boolean oldValue = isValueIsAdjusting();
this.valueIsAdjusting = valueIsAdjusting;
fireValueIsAdjusting(oldValue);
}
public int getHours(Integer time) {
return time == null ? 0 : time / 60;
}
public int getMinutes(Integer time) {
return time == null ? 0 : time % 60;
}
protected void setTime(Integer hour, Integer minute) {
Integer oldTime = getTime();
setValueIsAdjusting(true);
try {
if (hour == null || minute == null) {
this.time = null;
} else {
this.time = hour * 60 + minute;
}
} finally {
setValueIsAdjusting(false);
fireTime(oldTime);
}
}
protected void fireTime(Integer oldTime) {
firePropertyChange(PROPERTY_TIME, oldTime, getTime());
}
protected void fireValueIsAdjusting(boolean oldValue) {
firePropertyChange(PROPERTY_VALUE_IS_ADJUSTING, oldValue, isValueIsAdjusting());
}
protected Predicate canUpdateBeanValuePredicate() {
return input -> !isValueIsAdjusting();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy