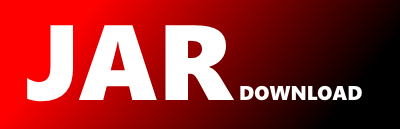
io.ultreia.java4all.bean.AbstractJavaBeanDefinition Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-bean Show documentation
Show all versions of java-bean Show documentation
Java Bean api extends by Ultreia.io
package io.ultreia.java4all.bean;
/*-
* #%L
* Java Beans extends by Ultreia.io
* %%
* Copyright (C) 2018 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import java.util.Objects;
import java.util.function.BiConsumer;
import java.util.function.Function;
import java.util.stream.Collectors;
public class AbstractJavaBeanDefinition implements JavaBeanDefinition {
private final ImmutableSet> types;
private final ImmutableMap getters;
private final ImmutableMap setters;
private final ImmutableSet copyPropertyNames;
@SuppressWarnings("unchecked")
public AbstractJavaBeanDefinition(ImmutableSet> types, ImmutableMap> getters, ImmutableMap> setters) {
this.types = types;
this.getters = (ImmutableMap) getters;
this.setters = (ImmutableMap) setters;
copyPropertyNames = ImmutableSet.copyOf(this.getters.keySet().stream().filter(this.setters::containsKey).collect(Collectors.toSet()));
}
@Override
public final ImmutableSet> types() {
return types;
}
@Override
public final ImmutableMap getters() {
return getters;
}
@Override
public final ImmutableMap setters() {
return setters;
}
@Override
public final ImmutableSet copyPropertyNames() {
return copyPropertyNames;
}
@SuppressWarnings("unchecked")
@Override
public final O get(JavaBean javaBean, String propertyName) {
Function getter = Objects.requireNonNull(getters.get(propertyName), String.format("Can't find getter for property %s on %s", propertyName, javaBean.getClass().getName()));
return (O) getter.apply(javaBean);
}
@SuppressWarnings("unchecked")
@Override
public final void set(JavaBean javaBean, String propertyName, O propertyValue) {
BiConsumer setter = Objects.requireNonNull(setters.get(propertyName), String.format("Can't find a setter for property: %s on %s", propertyName, javaBean.getClass().getName()));
setter.accept(javaBean, propertyValue);
}
@Override
public final void copy(JavaBean source, JavaBean target, String propertyName) {
Object o = get(source, propertyName);
set(target, propertyName, o);
}
@Override
public final void copy(JavaBean source, JavaBean target) {
for (String propertyName : copyPropertyNames) {
copy(source, target, propertyName);
}
}
@SuppressWarnings("unchecked")
@Override
public final void clear(JavaBean javaBean) {
for (BiConsumer o : setters.values()) {
try {
o.accept(javaBean, null);
} catch (Exception e) {
o.accept(javaBean, 0);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy