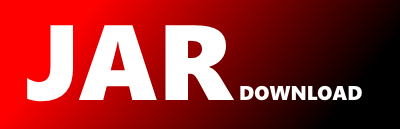
io.ultreia.java4all.bean.AbstractJavaBeanPredicateBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-bean Show documentation
Show all versions of java-bean Show documentation
Java Bean api extends by Ultreia.io
package io.ultreia.java4all.bean;
/*-
* #%L
* Java Beans extends by Ultreia.io
* %%
* Copyright (C) 2018 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import java.util.Objects;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* Created by tchemit on 11/01/2018.
*
* @author Tony Chemit - [email protected]
*/
public class AbstractJavaBeanPredicateBuilder> implements JavaBeanPredicateBuilder {
private final List> predicates;
private final JavaBeanDefinition javaBeanDefinition;
public AbstractJavaBeanPredicateBuilder(JavaBeanDefinition javaBeanDefinition) {
this.javaBeanDefinition = javaBeanDefinition;
this.predicates = new LinkedList<>();
}
protected AbstractJavaBeanPredicateBuilder(Class extends JavaBeanDefinition> javaBeanDefinitionType) {
this(JavaBeanDefinitionStore.definition(javaBeanDefinitionType));
}
@Override
public void addPredicate(Predicate predicate) {
predicates.add(predicate);
}
@Override
public Predicate build() {
Iterator> iterator = predicates.iterator();
if (!iterator.hasNext()) {
return d -> true;
}
Predicate result = iterator.next();
while (iterator.hasNext()) {
result = result.and(iterator.next());
}
return result;
}
protected ObjectQuery where(String propertyName) {
return new ObjectQuery<>(p(), this.getter(propertyName));
}
protected > ComparableQuery whereComparable(String propertyName) {
return new SimpleComparableQuery<>(p(), this.getter(propertyName));
}
protected > PrimitiveComparableQuery wherePrimitiveComparable(String propertyName) {
return new PrimitiveComparableQuery<>(p(), this.getter(propertyName));
}
protected BooleanQuery whereBoolean(String propertyName) {
return new BooleanQuery<>(p(), getter(propertyName));
}
protected PrimitiveBooleanQuery wherePrimitiveBoolean(String propertyName) {
return new PrimitiveBooleanQuery<>(p(), getter(propertyName));
}
protected StringQuery whereString(String propertyName) {
return new StringQuery<>(p(), getter(propertyName));
}
protected Function getter(String propertyName) {
return Objects.requireNonNull(javaBeanDefinition.getter(propertyName));
}
@SuppressWarnings("unchecked")
protected P p() {
return (P) this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy