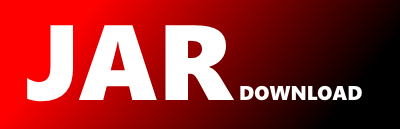
io.ultreia.java4all.bean.JavaBean Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-bean Show documentation
Show all versions of java-bean Show documentation
Java Bean api extends by Ultreia.io
package io.ultreia.java4all.bean;
/*-
* #%L
* Java Beans extends by Ultreia.io
* %%
* Copyright (C) 2018 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.beans.PropertyChangeListener;
/**
* Created by tchemit on 11/11/17.
*
* @author Tony Chemit - [email protected]
*/
public interface JavaBean extends GetterProducer, SetterProducer {
/**
* @return definition of this bean
*/
JavaBeanDefinition javaBeanDefinition();
@Override
default O get(String propertyName) {
return javaBeanDefinition().get(this, propertyName);
}
@Override
default void set(String propertyName, O propertyValue) {
javaBeanDefinition().set(this, propertyName, propertyValue);
}
default void copy(String propertyName, JavaBean target) {
javaBeanDefinition().copy(this, target, propertyName);
}
default void copy(JavaBean target) {
javaBeanDefinition().copy(this, target);
}
default void clear() {
javaBeanDefinition().clear(this);
}
default JavaBeanPredicateBuilder> predicateBuilder() {
return javaBeanDefinition().predicateBuilder();
}
default JavaBeanComparatorBuilder> comparatorBuilder() {
return javaBeanDefinition().comparatorBuilder();
}
/**
* Add a PropertyChangeListener for a specific property. The listener
* will be invoked only when a call on firePropertyChange names that
* specific property.
* The same listener object may be added more than once. For each
* property, the listener will be invoked the number of times it was added
* for that property.
* If propertyName
or listener
is null, no
* exception is thrown and no action is taken.
*
* @param propertyName The name of the property to listen on.
* @param listener The PropertyChangeListener to be added
*/
void addPropertyChangeListener(String propertyName, PropertyChangeListener listener);
/**
* Add a PropertyChangeListener to the listener list.
* The listener is registered for all properties.
* The same listener object may be added more than once, and will be called
* as many times as it is added.
* If listener
is null, no exception is thrown and no action
* is taken.
*
* @param listener The PropertyChangeListener to be added
*/
void addPropertyChangeListener(PropertyChangeListener listener);
/**
* Remove a PropertyChangeListener for a specific property.
* If listener
was added more than once to the same event
* source for the specified property, it will be notified one less time
* after being removed.
* If propertyName
is null, no exception is thrown and no
* action is taken.
* If listener
is null, or was never added for the specified
* property, no exception is thrown and no action is taken.
*
* @param propertyName The name of the property that was listened on.
* @param listener The PropertyChangeListener to be removed
*/
void removePropertyChangeListener(String propertyName, PropertyChangeListener listener);
/**
* Remove a PropertyChangeListener from the listener list.
* This removes a PropertyChangeListener that was registered
* for all properties.
* If listener
was added more than once to the same event
* source, it will be notified one less time after being removed.
* If listener
is null, or was never added, no exception is
* thrown and no action is taken.
*
* @param listener The PropertyChangeListener to be removed
*/
void removePropertyChangeListener(PropertyChangeListener listener);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy