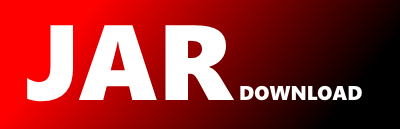
io.ultreia.java4all.lang.Numbers Maven / Gradle / Ivy
package io.ultreia.java4all.lang;
/*-
* #%L
* Java Lang extends by Ultreia.io
* %%
* Copyright (C) 2017 - 2021 Ultreia.io
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation, either version 3 of the
* License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Lesser Public License for more details.
*
* You should have received a copy of the GNU General Lesser Public
* License along with this program. If not, see
* .
* #L%
*/
import java.math.BigDecimal;
import java.math.MathContext;
import java.util.Arrays;
import java.util.function.Predicate;
/**
* Created by tchemit on 29/12/2017.
*
* @author Tony Chemit - [email protected]
*/
public class Numbers {
public static final Predicate NULL_OR_ZERO_INTEGER = input -> input == null || input == 0;
private static final MathContext mc1Digit = new MathContext(1);
public static final Predicate NULL_OR_ZERO_FLOAT_ONE_DIGIT = input -> input == null || Math.abs(roundOneDigit(input)) < 0.1;
private static final MathContext mc2Digits = new MathContext(2);
public static final Predicate NULL_OR_ZERO_FLOAT_TWO_DIGITS = input -> input == null || Math.abs(roundTwoDigits(input)) < 0.01;
private static final MathContext mc3Digits = new MathContext(3);
public static final Predicate NULL_OR_ZERO_FLOAT_THREE_DIGITS = input -> input == null || Math.abs(roundThreeDigits(input)) < 0.001;
private static final MathContext mc4Digits = new MathContext(4);
public static final Predicate NULL_OR_ZERO_FLOAT_FOUR_DIGITS = input -> input == null || Math.abs(roundFourDigits(input)) < 0.0001;
private static final MathContext mc5Digits = new MathContext(5);
public static final Predicate NULL_OR_ZERO_FLOAT_FIVE_DIGITS = input -> input == null || Math.abs(roundFiveDigits(input)) < 0.00001;
/**
* Divide the divisor by the dividend.
* Returns an array containing the quotients rounded up or down
* to ensure the sum of the quotients equals the divisor.
*
* e.g. divideAndEnsureSum(100, 3) returns {34, 33, 33}
*
* @param divisor the divisor
* @param dividend the dividend
* @return an array whose length equals dividend
*/
public static int[] divideAndEnsureSum(int divisor, int dividend) {
// dividing by 0 is not permitted
if (dividend == 0) {
return null;
}
int quotient = (int) ((double) divisor) / dividend;
int[] result = new int[dividend];
Arrays.fill(result, quotient);
int sum = quotient * dividend;
int i = 0;
while (sum != divisor && i < dividend) {
result[i]++;
sum++;
i++;
}
return result;
}
public static Float roundOneDigit(Float number) {
return round(number, mc1Digit);
}
public static Float roundTwoDigits(Float number) {
return round(number, mc2Digits);
}
public static Float roundThreeDigits(Float number) {
return round(number, mc3Digits);
}
public static Float roundFourDigits(Float number) {
return round(number, mc4Digits);
}
private static Float roundFiveDigits(Float number) {
return round(number, mc5Digits);
}
public static Float roundNDigits(Float number, int digits) {
return round(number, new MathContext(digits));
}
public static Float round(Float number, MathContext mc) {
float old = number;
float partieEntier = (int) old;
float digit = old - (int) old;
number = partieEntier + new BigDecimal(digit).round(mc).floatValue();
return number;
}
}