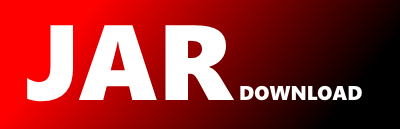
io.vanillabp.cockpit.adapter.common.wiring.TemplatingHandlerBase Maven / Gradle / Ivy
The newest version!
package io.vanillabp.cockpit.adapter.common.wiring;
import freemarker.template.Configuration;
import freemarker.template.TemplateNotFoundException;
import io.vanillabp.cockpit.adapter.common.properties.VanillaBpCockpitProperties;
import io.vanillabp.springboot.adapter.TaskHandlerBase;
import io.vanillabp.springboot.parameters.MethodParameter;
import java.io.File;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import org.slf4j.Logger;
import org.springframework.data.repository.CrudRepository;
import org.springframework.ui.freemarker.FreeMarkerTemplateUtils;
public abstract class TemplatingHandlerBase extends TaskHandlerBase {
protected final VanillaBpCockpitProperties properties;
protected final Optional templating;
public TemplatingHandlerBase(
final VanillaBpCockpitProperties properties,
final Optional templating,
final CrudRepository
© 2015 - 2025 Weber Informatics LLC | Privacy Policy