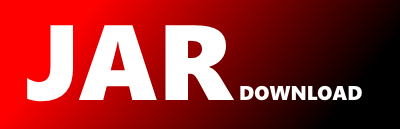
io.vavr.kotlin.Collections.kt Maven / Gradle / Ivy
/* __ __ __ __ __ ___
* \ \ / / \ \ / / __/
* \ \/ / /\ \ \/ / /
* \____/__/ \__\____/__/
*
* Copyright 2014-2017 Vavr, http://vavr.io
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vavr.kotlin
import io.vavr.Value
import io.vavr.collection.List
import io.vavr.collection.Map
import io.vavr.collection.Stream
/**
* Constructors and Kotlin collection converters for the Vavr collection values
*
* @author Alex Zuzin (github.com/zvozin)
*/
/**
* @see List.of
*/
fun list(vararg t: T):
List = List.of(*t)
/**
* Converts a Value (that is, _any_ Vavr data class) into a Kotlin MutableList
*/
fun Value.toMutableList():
MutableList = this.toJavaList().toMutableList()
/**
* Converts a Kotlin Iterable into a List
*/
fun Iterable.toVavrList():
List = List.ofAll(this)
/**
* Converts a Kotlin Array into a List
*/
fun Array.toVavrList():
List = List.ofAll(this.asIterable())
/**
* @see Stream.of
*/
fun stream(vararg t: T):
Stream = Stream.of(*t)
/**
* @see Stream.ofAll
*/
fun Iterable.toVavrStream():
Stream = Stream.ofAll(this)
/**
* Converts a Kotlin Array into a Stream
*/
fun Array.toVavrStream():
Stream = Stream.ofAll(this.asIterable())
/**
* Converts a Kotlin Sequence into a Stream
*/
fun Sequence.toVavrStream():
Stream = Stream.ofAll(this.asIterable())
/**
* Converts a Kotlin Map into a Vavr Map
*/
fun kotlin.collections.Map.toVavrMap():
io.vavr.collection.Map = io.vavr.collection.HashMap.ofAll(this)
/**
* Converts a Vavr Map to a Kotlin MutableMap
*/
fun io.vavr.collection.Map.toMutableMap():
kotlin.collections.MutableMap = this.toJavaMap().toMutableMap()
/**
* Creates a Vavr HashMap from a series of Kotlin Pairs
*/
fun hashMap(vararg p: Pair):
io.vavr.collection.HashMap =
io.vavr.collection.HashMap.ofEntries(p.asIterable().map { it.tuple() })
/**
* Creates a Vavr LinkedHashMap from a series of Kotlin Pairs
*/
fun linkedHashMap(vararg p: Pair):
io.vavr.collection.LinkedHashMap =
io.vavr.collection.LinkedHashMap.ofEntries(p.asIterable().map { it.tuple() })
/**
* Creates a Vavr TreeMap from a series of Kotlin Pairs.
* Notice that the keys of a TreeMap must be Comparable
*/
fun , V> treeMap(vararg p: Pair):
io.vavr.collection.TreeMap =
io.vavr.collection.TreeMap.ofEntries(p.asIterable().map { it.tuple() })
/**
* Returns the value associated with a key, or null if the key is not contained in the map.
*/
fun Map.getOrNull(key: K):
V? = this.getOrElse(key, null)
/**
* Converts a Kotlin Set into a Vavr Set
*/
fun kotlin.collections.Set.toVavrSet():
io.vavr.collection.Set = io.vavr.collection.HashSet.ofAll(this)
/**
* Converts a Vavr Set into a Kotlin MutableSet
*/
fun io.vavr.collection.Set.toMutableSet():
kotlin.collections.MutableSet = this.toJavaSet().toMutableSet()
/**
* Creates a Vavr HashSet
*/
fun hashSet(vararg t: T):
io.vavr.collection.HashSet = io.vavr.collection.HashSet.ofAll(t.asIterable())
/**
* Creates a Vavr LinkedHashSet
*/
fun linkedHashSet(vararg t: T):
io.vavr.collection.LinkedHashSet = io.vavr.collection.LinkedHashSet.ofAll(t.asIterable())
/**
* Creates a Vavr TreeSet.
* Notice that the elements of a TreeSet must be Comparable
*/
fun > treeSet(vararg t: T):
io.vavr.collection.TreeSet =
io.vavr.collection.TreeSet.ofAll(t.asIterable())
© 2015 - 2025 Weber Informatics LLC | Privacy Policy