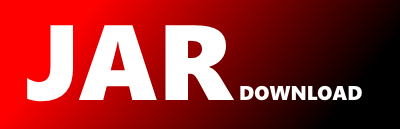
io.vavr.kotlin.Control.kt Maven / Gradle / Ivy
/* __ __ __ __ __ ___
* \ \ / / \ \ / / __/
* \ \/ / /\ \ \/ / /
* \____/__/ \__\____/__/
*
* Copyright 2014-2017 Vavr, http://vavr.io
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vavr.kotlin
import io.vavr.CheckedFunction0
import io.vavr.collection.Seq
import io.vavr.control.Either
import io.vavr.control.Option
import io.vavr.control.Option.none
import io.vavr.control.Option.some
import io.vavr.control.Try
import io.vavr.control.Validation
/**
* Constructors and Kotlin collection extensions for Option, Try, Validation and Either
*
* @author Alex Zuzin (github.com/zvozin)
*/
/**
* Creates an Option of a nullable.
* @return None() for null, Some(this) for a present value
*/
fun A?.option():
Option = if (this == null) none() else some(this)
/**
* @see Option.some
*/
fun some(a: A):
Option = Option.some(a)
/**
* @see Option.none
*/
fun none():
Option = Option.none()
/**
* Creates an Option predicated on a Boolean, using the supplied value.
* @return Some(a) if this is true, None() otherwise
*/
fun Boolean.option(a: A):
Option = if (this) some(a) else none()
/**
* Creates an Option predicated on a Boolean, using the supplier of value.
* @return Some(a.invoke()) if this is true, None() otherwise
*/
fun Boolean.option(a: () -> A):
Option = if (this) some(a.invoke()) else none()
/**
* @see Option.sequence
*/
fun Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy