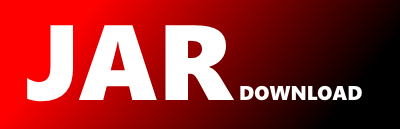
io.vavr.Tuple Maven / Gradle / Ivy
Show all versions of vavr Show documentation
/* ____ ______________ ________________________ __________
* \ \/ / \ \/ / __/ / \ \/ / \
* \______/___/\___\______/___/_____/___/\___\______/___/\___\
*
* Copyright 2019 Vavr, http://vavr.io
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vavr;
/*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*\
G E N E R A T O R C R A F T E D
\*-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-*/
import io.vavr.collection.Seq;
import io.vavr.collection.Stream;
import java.lang.Iterable;
import java.util.Map;
import java.util.Objects;
/**
* The base interface of all tuples.
*/
public interface Tuple {
/**
* The maximum arity of an Tuple.
*
* Note: This value might be changed in a future version of Vavr.
* So it is recommended to use this constant instead of hardcoding the current maximum arity.
*/
int MAX_ARITY = 8;
/**
* Returns the number of elements of this tuple.
*
* @return the number of elements.
*/
int arity();
/**
* Converts this tuple to a sequence.
*
* @return A new {@code Seq}.
*/
Seq> toSeq();
// -- factory methods
/**
* Creates the empty tuple.
*
* @return the empty tuple.
*/
static Tuple0 empty() {
return Tuple0.instance();
}
/**
* Creates a {@code Tuple2} from a {@link Map.Entry}.
*
* @param Type of first component (entry key)
* @param Type of second component (entry value)
* @param entry A {@link java.util.Map.Entry}
* @return a new {@code Tuple2} containing key and value of the given {@code entry}
*/
static Tuple2 fromEntry(Map.Entry extends T1, ? extends T2> entry) {
Objects.requireNonNull(entry, "entry is null");
return new Tuple2<>(entry.getKey(), entry.getValue());
}
/**
* Creates a tuple of one element.
*
* @param type of the 1st element
* @param t1 the 1st element
* @return a tuple of one element.
*/
static Tuple1 of(T1 t1) {
return new Tuple1<>(t1);
}
/**
* Creates a tuple of two elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param t1 the 1st element
* @param t2 the 2nd element
* @return a tuple of two elements.
*/
static Tuple2 of(T1 t1, T2 t2) {
return new Tuple2<>(t1, t2);
}
/**
* Creates a tuple of three elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param type of the 3rd element
* @param t1 the 1st element
* @param t2 the 2nd element
* @param t3 the 3rd element
* @return a tuple of three elements.
*/
static Tuple3 of(T1 t1, T2 t2, T3 t3) {
return new Tuple3<>(t1, t2, t3);
}
/**
* Creates a tuple of 4 elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param type of the 3rd element
* @param type of the 4th element
* @param t1 the 1st element
* @param t2 the 2nd element
* @param t3 the 3rd element
* @param t4 the 4th element
* @return a tuple of 4 elements.
*/
static Tuple4 of(T1 t1, T2 t2, T3 t3, T4 t4) {
return new Tuple4<>(t1, t2, t3, t4);
}
/**
* Creates a tuple of 5 elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param type of the 3rd element
* @param type of the 4th element
* @param type of the 5th element
* @param t1 the 1st element
* @param t2 the 2nd element
* @param t3 the 3rd element
* @param t4 the 4th element
* @param t5 the 5th element
* @return a tuple of 5 elements.
*/
static Tuple5 of(T1 t1, T2 t2, T3 t3, T4 t4, T5 t5) {
return new Tuple5<>(t1, t2, t3, t4, t5);
}
/**
* Creates a tuple of 6 elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param type of the 3rd element
* @param type of the 4th element
* @param type of the 5th element
* @param type of the 6th element
* @param t1 the 1st element
* @param t2 the 2nd element
* @param t3 the 3rd element
* @param t4 the 4th element
* @param t5 the 5th element
* @param t6 the 6th element
* @return a tuple of 6 elements.
*/
static Tuple6 of(T1 t1, T2 t2, T3 t3, T4 t4, T5 t5, T6 t6) {
return new Tuple6<>(t1, t2, t3, t4, t5, t6);
}
/**
* Creates a tuple of 7 elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param type of the 3rd element
* @param type of the 4th element
* @param type of the 5th element
* @param type of the 6th element
* @param type of the 7th element
* @param t1 the 1st element
* @param t2 the 2nd element
* @param t3 the 3rd element
* @param t4 the 4th element
* @param t5 the 5th element
* @param t6 the 6th element
* @param t7 the 7th element
* @return a tuple of 7 elements.
*/
static Tuple7 of(T1 t1, T2 t2, T3 t3, T4 t4, T5 t5, T6 t6, T7 t7) {
return new Tuple7<>(t1, t2, t3, t4, t5, t6, t7);
}
/**
* Creates a tuple of 8 elements.
*
* @param type of the 1st element
* @param type of the 2nd element
* @param type of the 3rd element
* @param type of the 4th element
* @param type of the 5th element
* @param type of the 6th element
* @param type of the 7th element
* @param type of the 8th element
* @param t1 the 1st element
* @param t2 the 2nd element
* @param t3 the 3rd element
* @param t4 the 4th element
* @param t5 the 5th element
* @param t6 the 6th element
* @param t7 the 7th element
* @param t8 the 8th element
* @return a tuple of 8 elements.
*/
static Tuple8 of(T1 t1, T2 t2, T3 t3, T4 t4, T5 t5, T6 t6, T7 t7, T8 t8) {
return new Tuple8<>(t1, t2, t3, t4, t5, t6, t7, t8);
}
/**
* Return the order-dependent hash of the one given value.
*
* @param o1 the 1st value to hash
* @return the same result as {@link Objects#hashCode(Object)}
*/
static int hash(Object o1) {
return Objects.hashCode(o1);
}
/**
* Return the order-dependent hash of the two given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
return result;
}
/**
* Return the order-dependent hash of the three given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @param o3 the 3rd value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2, Object o3) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
result = 31 * result + hash(o3);
return result;
}
/**
* Return the order-dependent hash of the 4 given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @param o3 the 3rd value to hash
* @param o4 the 4th value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2, Object o3, Object o4) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
result = 31 * result + hash(o3);
result = 31 * result + hash(o4);
return result;
}
/**
* Return the order-dependent hash of the 5 given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @param o3 the 3rd value to hash
* @param o4 the 4th value to hash
* @param o5 the 5th value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2, Object o3, Object o4, Object o5) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
result = 31 * result + hash(o3);
result = 31 * result + hash(o4);
result = 31 * result + hash(o5);
return result;
}
/**
* Return the order-dependent hash of the 6 given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @param o3 the 3rd value to hash
* @param o4 the 4th value to hash
* @param o5 the 5th value to hash
* @param o6 the 6th value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2, Object o3, Object o4, Object o5, Object o6) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
result = 31 * result + hash(o3);
result = 31 * result + hash(o4);
result = 31 * result + hash(o5);
result = 31 * result + hash(o6);
return result;
}
/**
* Return the order-dependent hash of the 7 given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @param o3 the 3rd value to hash
* @param o4 the 4th value to hash
* @param o5 the 5th value to hash
* @param o6 the 6th value to hash
* @param o7 the 7th value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2, Object o3, Object o4, Object o5, Object o6, Object o7) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
result = 31 * result + hash(o3);
result = 31 * result + hash(o4);
result = 31 * result + hash(o5);
result = 31 * result + hash(o6);
result = 31 * result + hash(o7);
return result;
}
/**
* Return the order-dependent hash of the 8 given values.
*
* @param o1 the 1st value to hash
* @param o2 the 2nd value to hash
* @param o3 the 3rd value to hash
* @param o4 the 4th value to hash
* @param o5 the 5th value to hash
* @param o6 the 6th value to hash
* @param o7 the 7th value to hash
* @param o8 the 8th value to hash
* @return the same result as {@link Objects#hash(Object...)}
*/
static int hash(Object o1, Object o2, Object o3, Object o4, Object o5, Object o6, Object o7, Object o8) {
int result = 1;
result = 31 * result + hash(o1);
result = 31 * result + hash(o2);
result = 31 * result + hash(o3);
result = 31 * result + hash(o4);
result = 31 * result + hash(o5);
result = 31 * result + hash(o6);
result = 31 * result + hash(o7);
result = 31 * result + hash(o8);
return result;
}
/**
* Narrows a widened {@code Tuple1 extends T1>} to {@code Tuple1}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple1}.
* @param the 1st component type
* @return the given {@code t} instance as narrowed type {@code Tuple1}.
*/
@SuppressWarnings("unchecked")
static Tuple1 narrow(Tuple1 extends T1> t) {
return (Tuple1) t;
}
/**
* Narrows a widened {@code Tuple2 extends T1, ? extends T2>} to {@code Tuple2}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple2}.
* @param the 1st component type
* @param the 2nd component type
* @return the given {@code t} instance as narrowed type {@code Tuple2}.
*/
@SuppressWarnings("unchecked")
static Tuple2 narrow(Tuple2 extends T1, ? extends T2> t) {
return (Tuple2) t;
}
/**
* Narrows a widened {@code Tuple3 extends T1, ? extends T2, ? extends T3>} to {@code Tuple3}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple3}.
* @param the 1st component type
* @param the 2nd component type
* @param the 3rd component type
* @return the given {@code t} instance as narrowed type {@code Tuple3}.
*/
@SuppressWarnings("unchecked")
static Tuple3 narrow(Tuple3 extends T1, ? extends T2, ? extends T3> t) {
return (Tuple3) t;
}
/**
* Narrows a widened {@code Tuple4 extends T1, ? extends T2, ? extends T3, ? extends T4>} to {@code Tuple4}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple4}.
* @param the 1st component type
* @param the 2nd component type
* @param the 3rd component type
* @param the 4th component type
* @return the given {@code t} instance as narrowed type {@code Tuple4}.
*/
@SuppressWarnings("unchecked")
static Tuple4 narrow(Tuple4 extends T1, ? extends T2, ? extends T3, ? extends T4> t) {
return (Tuple4) t;
}
/**
* Narrows a widened {@code Tuple5 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5>} to {@code Tuple5}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple5}.
* @param the 1st component type
* @param the 2nd component type
* @param the 3rd component type
* @param the 4th component type
* @param the 5th component type
* @return the given {@code t} instance as narrowed type {@code Tuple5}.
*/
@SuppressWarnings("unchecked")
static Tuple5 narrow(Tuple5 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5> t) {
return (Tuple5) t;
}
/**
* Narrows a widened {@code Tuple6 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6>} to {@code Tuple6}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple6}.
* @param the 1st component type
* @param the 2nd component type
* @param the 3rd component type
* @param the 4th component type
* @param the 5th component type
* @param the 6th component type
* @return the given {@code t} instance as narrowed type {@code Tuple6}.
*/
@SuppressWarnings("unchecked")
static Tuple6 narrow(Tuple6 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6> t) {
return (Tuple6) t;
}
/**
* Narrows a widened {@code Tuple7 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6, ? extends T7>} to {@code Tuple7}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple7}.
* @param the 1st component type
* @param the 2nd component type
* @param the 3rd component type
* @param the 4th component type
* @param the 5th component type
* @param the 6th component type
* @param the 7th component type
* @return the given {@code t} instance as narrowed type {@code Tuple7}.
*/
@SuppressWarnings("unchecked")
static Tuple7 narrow(Tuple7 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6, ? extends T7> t) {
return (Tuple7) t;
}
/**
* Narrows a widened {@code Tuple8 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6, ? extends T7, ? extends T8>} to {@code Tuple8}.
* This is eligible because immutable/read-only tuples are covariant.
* @param t A {@code Tuple8}.
* @param the 1st component type
* @param the 2nd component type
* @param the 3rd component type
* @param the 4th component type
* @param the 5th component type
* @param the 6th component type
* @param the 7th component type
* @param the 8th component type
* @return the given {@code t} instance as narrowed type {@code Tuple8}.
*/
@SuppressWarnings("unchecked")
static Tuple8 narrow(Tuple8 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6, ? extends T7, ? extends T8> t) {
return (Tuple8) t;
}
/**
* Turns a sequence of {@code Tuple1} into a Tuple1 of {@code Seq}.
*
* @param 1st component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of one {@link Seq}.
*/
static Tuple1> sequence1(Iterable extends Tuple1 extends T1>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple1<>(s.map(Tuple1::_1));
}
/**
* Turns a sequence of {@code Tuple2} into a Tuple2 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of two {@link Seq}s.
*/
static Tuple2, Seq> sequence2(Iterable extends Tuple2 extends T1, ? extends T2>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple2<>(s.map(Tuple2::_1), s.map(Tuple2::_2));
}
/**
* Turns a sequence of {@code Tuple3} into a Tuple3 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param 3rd component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of three {@link Seq}s.
*/
static Tuple3, Seq, Seq> sequence3(Iterable extends Tuple3 extends T1, ? extends T2, ? extends T3>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple3<>(s.map(Tuple3::_1), s.map(Tuple3::_2), s.map(Tuple3::_3));
}
/**
* Turns a sequence of {@code Tuple4} into a Tuple4 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param 3rd component type
* @param 4th component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of 4 {@link Seq}s.
*/
static Tuple4, Seq, Seq, Seq> sequence4(Iterable extends Tuple4 extends T1, ? extends T2, ? extends T3, ? extends T4>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple4<>(s.map(Tuple4::_1), s.map(Tuple4::_2), s.map(Tuple4::_3), s.map(Tuple4::_4));
}
/**
* Turns a sequence of {@code Tuple5} into a Tuple5 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param 3rd component type
* @param 4th component type
* @param 5th component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of 5 {@link Seq}s.
*/
static Tuple5, Seq, Seq, Seq, Seq> sequence5(Iterable extends Tuple5 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple5<>(s.map(Tuple5::_1), s.map(Tuple5::_2), s.map(Tuple5::_3), s.map(Tuple5::_4), s.map(Tuple5::_5));
}
/**
* Turns a sequence of {@code Tuple6} into a Tuple6 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param 3rd component type
* @param 4th component type
* @param 5th component type
* @param 6th component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of 6 {@link Seq}s.
*/
static Tuple6, Seq, Seq, Seq, Seq, Seq> sequence6(Iterable extends Tuple6 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple6<>(s.map(Tuple6::_1), s.map(Tuple6::_2), s.map(Tuple6::_3), s.map(Tuple6::_4), s.map(Tuple6::_5), s.map(Tuple6::_6));
}
/**
* Turns a sequence of {@code Tuple7} into a Tuple7 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param 3rd component type
* @param 4th component type
* @param 5th component type
* @param 6th component type
* @param 7th component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of 7 {@link Seq}s.
*/
static Tuple7, Seq, Seq, Seq, Seq, Seq, Seq> sequence7(Iterable extends Tuple7 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6, ? extends T7>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple7<>(s.map(Tuple7::_1), s.map(Tuple7::_2), s.map(Tuple7::_3), s.map(Tuple7::_4), s.map(Tuple7::_5), s.map(Tuple7::_6), s.map(Tuple7::_7));
}
/**
* Turns a sequence of {@code Tuple8} into a Tuple8 of {@code Seq}s.
*
* @param 1st component type
* @param 2nd component type
* @param 3rd component type
* @param 4th component type
* @param 5th component type
* @param 6th component type
* @param 7th component type
* @param 8th component type
* @param tuples a {@link Iterable} of tuples
* @return a tuple of 8 {@link Seq}s.
*/
static Tuple8, Seq, Seq, Seq, Seq, Seq, Seq, Seq> sequence8(Iterable extends Tuple8 extends T1, ? extends T2, ? extends T3, ? extends T4, ? extends T5, ? extends T6, ? extends T7, ? extends T8>> tuples) {
Objects.requireNonNull(tuples, "tuples is null");
final Stream> s = Stream.ofAll(tuples);
return new Tuple8<>(s.map(Tuple8::_1), s.map(Tuple8::_2), s.map(Tuple8::_3), s.map(Tuple8::_4), s.map(Tuple8::_5), s.map(Tuple8::_6), s.map(Tuple8::_7), s.map(Tuple8::_8));
}
}