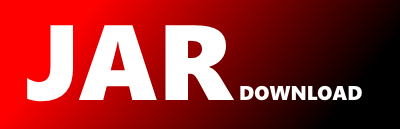
io.vertigo.account.authorization.definitions.Authorization Maven / Gradle / Ivy
The newest version!
/*
* vertigo - application development platform
*
* Copyright (C) 2013-2024, Vertigo.io, [email protected]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vertigo.account.authorization.definitions;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import io.vertigo.account.authorization.definitions.rulemodel.RuleMultiExpression;
import io.vertigo.core.lang.Assertion;
import io.vertigo.core.node.definition.AbstractDefinition;
import io.vertigo.core.node.definition.DefinitionPrefix;
import io.vertigo.datamodel.data.definitions.DataDefinition;
/**
* Une authorization est un droit sur une fonction de l'application.
* Ou sur une opération sur une entite.
* Sous condition d'un ensemble de règles.
*
* @author prahmoune, npiedeloup
*/
@DefinitionPrefix(Authorization.PREFIX)
public final class Authorization extends AbstractDefinition {
public static final String PREFIX = "Atz";
//soit authorization globale (sans règle)
//soit authorization = une opération sur une entity
private final Optional comment;
private final String label;
private final Set overrides;
private final Set grants;
private final List rules; //empty -> always true
private final Optional entityOpt;
private final Optional operationOpt;
/**
* Constructor.
*
* @param code Code de l'authorization
* @param label Label
* @param comment Comment
*/
public Authorization(final String code, final String label, final Optional comment) {
super(PREFIX + code);
//---
Assertion.check()
.isNotBlank(label)
.isNotNull(comment);
//---
this.label = label;
overrides = Collections.emptySet();
grants = Collections.emptySet();
entityOpt = Optional.empty();
operationOpt = Optional.empty();
rules = List.of();
this.comment = comment;
}
/**
* Constructor.
*
* @param operation Nom de l'opération
* @param label Label
* @param entityDefinition Entity definition
* @param overrides Liste des opérations overridé par cette opération
* @param grants Liste des opérations données par cette opération
* @param rules Règles d'évaluation
* @param comment Comment
*/
public Authorization(
final String operation,
final String label,
final Set overrides,
final Set grants,
final DataDefinition entityDefinition,
final List rules,
final Optional comment) {
super(PREFIX + entityDefinition.id().shortName() + '$' + operation);
//---
Assertion.check()
.isNotBlank(operation)
.isNotBlank(label)
.isNotNull(overrides)
.isNotNull(grants)
.isNotNull(entityDefinition)
.isNotNull(rules)
.isNotNull(comment);
//---
this.label = label;
this.overrides = new HashSet<>(overrides);
this.grants = new HashSet<>(grants);
entityOpt = Optional.of(entityDefinition);
operationOpt = Optional.of(operation);
this.rules = new ArrayList<>(rules);
this.comment = comment;
}
/**
* @return Label de la authorization
*/
public String getLabel() {
return label;
}
/**
* @return Comment de la authorization
*/
public Optional getComment() {
return comment;
}
/**
* @return Overrides for this authorization
*/
public Set getOverrides() {
return overrides;
}
/**
* @return Grants for this authorization
*/
public Set getGrants() {
return grants;
}
/**
* @return Rules used to check authorization (empty->Always true)
*/
public List getRules() {
return rules;
}
/**
* @return entity definition
*/
public Optional getEntityDefinition() {
return entityOpt;
}
/**
* @return Operation
*/
public Optional getOperation() {
return operationOpt;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy