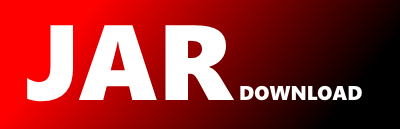
io.vertigo.dynamo.plugins.environment.loaders.kpr.rules.DslDefinitionEntryRule Maven / Gradle / Ivy
/**
* vertigo - simple java starter
*
* Copyright (C) 2013-2016, KleeGroup, [email protected] (http://www.kleegroup.com)
* KleeGroup, Centre d'affaire la Boursidiere - BP 159 - 92357 Le Plessis Robinson Cedex - France
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vertigo.dynamo.plugins.environment.loaders.kpr.rules;
import static io.vertigo.dynamo.plugins.environment.loaders.kpr.rules.DslSyntaxRules.PAIR_SEPARATOR;
import static io.vertigo.dynamo.plugins.environment.loaders.kpr.rules.DslSyntaxRules.SPACES;
import static io.vertigo.dynamo.plugins.environment.loaders.kpr.rules.DslSyntaxRules.WORD;
import static io.vertigo.dynamo.plugins.environment.loaders.kpr.rules.DslWordsRule.WORDS;
import java.util.ArrayList;
import java.util.List;
import io.vertigo.commons.peg.AbstractRule;
import io.vertigo.commons.peg.PegChoice;
import io.vertigo.commons.peg.PegRule;
import io.vertigo.commons.peg.PegRules;
import io.vertigo.dynamo.plugins.environment.loaders.kpr.definition.DslDefinitionEntry;
import io.vertigo.lang.Assertion;
/**
* règle de déclaration d'une champ référenéant une listes de clés.
* @author pchretien
*/
public final class DslDefinitionEntryRule extends AbstractRule> {
/**
* Constructeur.
*/
public DslDefinitionEntryRule(final List fieldNames) {
super(createMainRule(fieldNames));
}
private static PegRule> createMainRule(final List fieldNames) {
Assertion.checkNotNull(fieldNames);
//-----
final List> fieldNamesRules = new ArrayList<>();
for (final String fieldName : fieldNames) {
fieldNamesRules.add(PegRules.term(fieldName));
}
//-----
return PegRules.sequence(//"DefinitionKey"
PegRules.choice(fieldNamesRules), //0
SPACES,
PAIR_SEPARATOR,
SPACES,
PegRules.choice(WORD, WORDS), //4
SPACES,
PegRules.optional(DslSyntaxRules.OBJECT_SEPARATOR));
}
@Override
protected DslDefinitionEntry handle(final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy