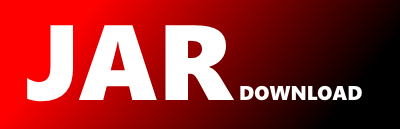
io.vertigo.dynamo.plugins.environment.loaders.kpr.rules.DsllnnerDefinitionRule Maven / Gradle / Ivy
/**
* vertigo - simple java starter
*
* Copyright (C) 2013-2016, KleeGroup, [email protected] (http://www.kleegroup.com)
* KleeGroup, Centre d'affaire la Boursidiere - BP 159 - 92357 Le Plessis Robinson Cedex - France
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.vertigo.dynamo.plugins.environment.loaders.kpr.rules;
import java.util.List;
import io.vertigo.commons.peg.AbstractRule;
import io.vertigo.commons.peg.PegRule;
import io.vertigo.commons.peg.PegRules;
import io.vertigo.core.definition.dsl.dynamic.DynamicDefinitionBuilder;
import io.vertigo.core.definition.dsl.dynamic.DynamicDefinitionRepository;
import io.vertigo.core.definition.dsl.entity.DslEntity;
import io.vertigo.dynamo.plugins.environment.loaders.kpr.definition.DslDefinitionBody;
import io.vertigo.dynamo.plugins.environment.loaders.kpr.definition.DslDefinitionEntry;
import io.vertigo.dynamo.plugins.environment.loaders.kpr.definition.DslPropertyEntry;
import io.vertigo.lang.Assertion;
final class DslInnerDefinitionRule extends AbstractRule> {
private final String entityName;
private final DslEntity entity;
DslInnerDefinitionRule(final String entityName, final DslEntity entity) {
super(createMainRule(entityName, entity), entityName);
this.entityName = entityName;
this.entity = entity;
}
private static PegRule> createMainRule(final String entityName, final DslEntity entity) {
Assertion.checkArgNotEmpty(entityName);
Assertion.checkNotNull(entity);
//-----
final DslDefinitionBodyRule definitionBodyRule = new DslDefinitionBodyRule(entity);
return PegRules.sequence(//"InnerDefinition"
PegRules.term(entityName),
DslSyntaxRules.SPACES,
DslSyntaxRules.WORD, //2
DslSyntaxRules.SPACES,
definitionBodyRule, //4
DslSyntaxRules.SPACES,
PegRules.optional(DslSyntaxRules.OBJECT_SEPARATOR));
}
@Override
protected DslDefinitionEntry handle(final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy