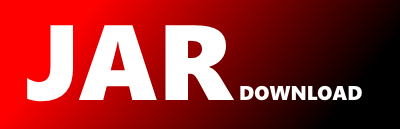
io.vertx.ext.consul.ConsulClient Maven / Gradle / Ivy
/*
* Copyright (c) 2016 The original author or authors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.ext.consul;
import io.vertx.codegen.annotations.VertxGen;
import io.vertx.core.Future;
import io.vertx.core.Vertx;
import io.vertx.core.json.JsonObject;
import io.vertx.ext.consul.impl.ConsulClientImpl;
import io.vertx.ext.consul.policy.AclPolicy;
import io.vertx.ext.consul.token.CloneAclTokenOptions;
import java.util.List;
/**
* A Vert.x service used to interact with Consul.
*
* @author Ruslan Sennov
*/
@VertxGen
public interface ConsulClient {
/**
* Create a Consul client with default options.
*
* @param vertx the Vert.x instance
* @return the client
*/
static ConsulClient create(Vertx vertx) {
return new ConsulClientImpl(vertx, new ConsulClientOptions());
}
/**
* Create a Consul client.
*
* @param vertx the Vert.x instance
* @param options the options
* @return the client
*/
static ConsulClient create(Vertx vertx, ConsulClientOptions options) {
return new ConsulClientImpl(vertx, options);
}
/**
* Returns the configuration and member information of the local agent
*
* @return a future provided with the configuration and member information of the local agent
* @see /v1/agent/self endpoint
*/
Future agentInfo();
/**
* Returns the LAN network coordinates for all nodes in a given DC
*
* @return a future provided with network coordinates of nodes in datacenter
* @see /v1/coordinate/nodes endpoint
*/
Future coordinateNodes();
/**
* Returns the LAN network coordinates for all nodes in a given DC
* This is blocking query unlike {@link ConsulClient#coordinateNodes()}
*
* @param options the blocking options
* @return a future provided with network coordinates of nodes in datacenter
* @see /v1/coordinate/nodes endpoint
*/
Future coordinateNodesWithOptions(BlockingQueryOptions options);
/**
* Returns the WAN network coordinates for all Consul servers, organized by DCs
*
* @return a future provided with network coordinates for all Consul servers
* @see /v1/coordinate/datacenters endpoint
*/
Future> coordinateDatacenters();
/**
* Returns the list of keys that corresponding to the specified key prefix.
*
* @param keyPrefix the prefix
* @return a future provided with keys list
* @see /v1/kv/:key endpoint
*/
Future> getKeys(String keyPrefix);
/**
* Returns the list of keys that corresponding to the specified key prefix.
*
* @param keyPrefix the prefix
* @param options the blocking options
* @return a future provided with keys list
* @see /v1/kv/:key endpoint
*/
Future> getKeysWithOptions(String keyPrefix, BlockingQueryOptions options);
/**
* Returns key/value pair that corresponding to the specified key.
* An empty {@link KeyValue} object will be returned if no such key is found.
*
* @param key the key
* @return a future provided with key/value pair
* @see /v1/kv/:key endpoint
*/
Future getValue(String key);
/**
* Returns key/value pair that corresponding to the specified key.
* An empty {@link KeyValue} object will be returned if no such key is found.
* This is blocking query unlike {@link ConsulClient#getValue(String)}
*
* @param key the key
* @param options the blocking options
* @return a future provided with key/value pair
* @see /v1/kv/:key endpoint
*/
Future getValueWithOptions(String key, BlockingQueryOptions options);
/**
* Remove the key/value pair that corresponding to the specified key
*
* @param key the key
* @return a future notified on complete
* @see /v1/kv/:key endpoint
*/
Future deleteValue(String key);
/**
* Returns the list of key/value pairs that corresponding to the specified key prefix.
* An empty {@link KeyValueList} object will be returned if no such key prefix is found.
*
* @param keyPrefix the prefix
* @return a future provided with list of key/value pairs
* @see /v1/kv/:key endpoint
*/
Future getValues(String keyPrefix);
/**
* Returns the list of key/value pairs that corresponding to the specified key prefix.
* An empty {@link KeyValueList} object will be returned if no such key prefix is found.
* This is blocking query unlike {@link ConsulClient#getValues(String)}
*
* @param keyPrefix the prefix
* @param options the blocking options
* @return a future provided with list of key/value pairs
* @see /v1/kv/:key endpoint
*/
Future getValuesWithOptions(String keyPrefix, BlockingQueryOptions options);
/**
* Removes all the key/value pair that corresponding to the specified key prefix
*
* @param keyPrefix the prefix
* @return a future notified on complete
* @see /v1/kv/:key endpoint
*/
Future deleteValues(String keyPrefix);
/**
* Adds specified key/value pair
*
* @param key the key
* @param value the value
* @return a future provided with success of operation
* @see /v1/kv/:key endpoint
*/
Future putValue(String key, String value);
/**
* @param key the key
* @param value the value
* @param options options used to push pair
* @return a future provided with success of operation
* @see /v1/kv/:key endpoint
*/
Future putValueWithOptions(String key, String value, KeyValueOptions options);
/**
* Manages multiple operations inside a single, atomic transaction.
*
* @param request transaction request
* @return a future provided with result of transaction
* @see /v1/txn endpoint
*/
Future transaction(TxnRequest request);
/**
* Creates a new ACL policy
*
* @param policy properties of policy
* @return a future provided with ID of created policy
* @see /acl/policy
*/
Future createAclPolicy(AclPolicy policy);
/**
* This endpoint reads an ACL policy with the given ID
*
* @param id uuid policy
* @return a future of AclPolicy
* @see GET /v1/acl/policy/:id
*/
Future readPolicy(String id);
/**
* This endpoint reads an ACL policy with the given name
*
* @param name unique name of created policy
* @return a future of AclPolicy like in {@link #readPolicy(String)}
* @see GET /v1/acl/policy/name/:name
*/
Future readPolicyByName(String name);
/**
* This endpoint updates an existing ACL policy
*
* @param id uuid of existing policy
* @param policy options that will be applied to the existing policy
* @return a future of AclPolicy
* @see PUT /acl/policy/:id
*/
Future updatePolicy(String id, AclPolicy policy);
/**
* This endpoint deletes an ACL policy
*
* @param id uuid of existing policy
* @return a future boolean value: true or false, indicating whether the deletion was successful
* @see DELETE /acl/policy/:id
*/
Future deletePolicy(String id);
/**
* This endpoint lists all the ACL policies.
* Note - The policies rules are not included in the listing and must be retrieved by the policy reading endpoint
*
* @return a future of all acl policies
* @see GET /acl/policies
*/
Future> getAclPolicies();
/**
* Create an Acl token
*
* @param token properties of the token
* @return a future NewAclToken in which two fields accessorId and secretId.
* {@link AclToken} accessorId - required in the URL path or JSON body for getting, updating and cloning token.
* {@link AclToken} secretId - using in {@link ConsulClientOptions#setAclToken(String)}.
* @see /v1/acl/create endpoint
*/
Future createAclToken(io.vertx.ext.consul.token.AclToken token);
/**
* Update an existing Acl token
*
* @param accessorId uuid of the token
* @param token properties of the token
* @return a future NewAclToken like in {@link #createAclToken(io.vertx.ext.consul.token.AclToken)}
* @see /acl/token/:accessorId endpoint
*/
Future updateAclToken(String accessorId, io.vertx.ext.consul.token.AclToken token);
/**
* Clones an existing ACL token
*
* @param accessorId uuid of the token
* @param cloneAclTokenOptions properties of cloned token
* @return a future NewAclToken like in {@link #createAclToken(io.vertx.ext.consul.token.AclToken)}
* @see /acl/token/:accessorId/clone endpoint
*/
Future cloneAclToken(String accessorId, CloneAclTokenOptions cloneAclTokenOptions);
/**
* Get list of Acl token
*
* @return a future provided with list of tokens
* @see /v1/acl/tokens endpoint
*/
Future> getAclTokens();
/**
* Reads an ACL token with the given Accessor ID
*
* @param accessorId uuid of token
* @return a future provided with token
* @see /v1/acl/token/:AccessorID endpoint
*/
Future readAclToken(String accessorId);
/**
* Deletes an ACL token
* @param accessorId uuid of token
* @return a future boolean value: true or false, indicating whether the deletion was successful.
*/
Future deleteAclToken(String accessorId);
/**
* Legacy create new Acl token
*
* @param token properties of the token
* @return a future provided with ID of created token
* @see /v1/acl/create endpoint
* @deprecated Use {@link #createAclToken(io.vertx.ext.consul.token.AclToken)} instead
*/
@Deprecated
Future createAclToken(AclToken token);
/**
* Update Acl token
*
* @param token properties of the token to be updated
* @return a future provided with ID of updated
* @see /v1/acl/update endpoint
* @deprecated Use {@link #updateAclToken(String, io.vertx.ext.consul.token.AclToken)} instead
*/
@Deprecated
Future updateAclToken(AclToken token);
/**
* Clone Acl token
*
* @param id the ID of token to be cloned
* @return a future provided with ID of cloned token
* @see /v1/acl/clone/:uuid endpoint
* @deprecated Use {@link #cloneAclToken(String, CloneAclTokenOptions)} instead
*/
@Deprecated
Future cloneAclToken(String id);
/**
* Get list of Acl token
*
* @return a future provided with list of tokens
* @see /v1/acl/list endpoint
* @deprecated Use {@link #getAclTokens()} instead
*/
@Deprecated
Future> listAclTokens();
/**
* Get info of Acl token
*
* @param id the ID of token
* @return a future provided with token
* @see /v1/acl/info/:uuid endpoint
* @deprecated Use {@link #readAclToken(String)} instead
*/
@Deprecated
Future infoAclToken(String id);
/**
* Destroy Acl token
*
* @param id the ID of token
* @return a future notified on complete
* @see /v1/acl/destroy/:uuid endpoint
* @deprecated Use {@link #deleteAclToken(String)} instead
*/
@Deprecated
Future destroyAclToken(String id);
/**
* Fires a new user event
*
* @param name name of event
* @return a future provided with properties of event
* @see /v1/event/fire/:name endpoint
*/
Future fireEvent(String name);
/**
* Fires a new user event
*
* @param name name of event
* @param options options used to create event
* @return a future provided with properties of event
* @see /v1/event/fire/:name endpoint
*/
Future fireEventWithOptions(String name, EventOptions options);
/**
* Returns the most recent events known by the agent
*
* @return a future provided with list of events
* @see /v1/event/list endpoint
*/
Future listEvents();
/**
* Returns the most recent events known by the agent.
* This is blocking query unlike {@link ConsulClient#listEvents()}. However, the semantics of this endpoint
* are slightly different. Most blocking queries provide a monotonic index and block until a newer index is available.
* This can be supported as a consequence of the total ordering of the consensus protocol. With gossip,
* there is no ordering, and instead {@code X-Consul-Index} maps to the newest event that matches the query.
*
* In practice, this means the index is only useful when used against a single agent and has no meaning globally.
* Because Consul defines the index as being opaque, clients should not be expecting a natural ordering either.
*
* @param options the blocking options
* @return a future provided with list of events
* @see /v1/event/list endpoint
*/
Future listEventsWithOptions(EventListOptions options);
/**
* Adds a new service, with an optional health check, to the local agent.
*
* @param serviceOptions the options of new service
* @return a future notified when complete
* @see /v1/agent/service/register endpoint
* @see ServiceOptions
*/
Future registerService(ServiceOptions serviceOptions);
/**
* Places a given service into "maintenance mode"
*
* @param maintenanceOptions the maintenance options
* @return a future notified when complete
* @see /v1/agent/service/maintenance/:service_id endpoint
* @see MaintenanceOptions
*/
Future maintenanceService(MaintenanceOptions maintenanceOptions);
/**
* Remove a service from the local agent. The agent will take care of deregistering the service with the Catalog.
* If there is an associated check, that is also deregistered.
*
* @param id the ID of service
* @return a future notified when complete
* @see /v1/agent/service/deregister/:service_id endpoint
*/
Future deregisterService(String id);
/**
* Returns the nodes providing a service
*
* @param service name of service
* @return a future provided with list of nodes providing given service
* @see /v1/catalog/service/:service endpoint
*/
Future catalogServiceNodes(String service);
/**
* Returns the nodes providing a service
*
* @param service name of service
* @param options options used to request services
* @return a future provided with list of nodes providing given service
* @see /v1/catalog/service/:service endpoint
*/
Future catalogServiceNodesWithOptions(String service, ServiceQueryOptions options);
/**
* Return all the datacenters that are known by the Consul server
*
* @return a future provided with list of datacenters
* @see /v1/catalog/datacenters endpoint
*/
Future> catalogDatacenters();
/**
* Returns the nodes registered in a datacenter
*
* @return a future provided with list of nodes
* @see /v1/catalog/nodes endpoint
*/
Future catalogNodes();
/**
* Returns the nodes registered in a datacenter
*
* @param options options used to request nodes
* @return a future provided with list of nodes
* @see /v1/catalog/nodes endpoint
*/
Future catalogNodesWithOptions(NodeQueryOptions options);
/**
* Returns the checks associated with the service
*
* @param service the service name
* @return a future provided with list of checks
* @see /v1/health/checks/:service endpoint
*/
Future healthChecks(String service);
/**
* Returns the checks associated with the service
*
* @param service the service name
* @param options options used to request checks
* @return a future provided with list of checks
* @see /v1/health/checks/:service endpoint
*/
Future healthChecksWithOptions(String service, CheckQueryOptions options);
/**
* Returns the checks in the specified status
*
* @param healthState the health state
* @return a future provided with list of checks
* @see /v1/health/state/:state endpoint
*/
Future healthState(HealthState healthState);
/**
* Returns the checks in the specified status
*
* @param healthState the health state
* @param options options used to request checks
* @return a future provided with list of checks
* @see /v1/health/state/:state endpoint
*/
Future healthStateWithOptions(HealthState healthState, CheckQueryOptions options);
/**
* Returns the nodes providing the service. This endpoint is very similar to the {@link ConsulClient#catalogServiceNodes} endpoint;
* however, this endpoint automatically returns the status of the associated health check as well as any system level health checks.
*
* @param service the service name
* @param passing if true, filter results to only nodes with all checks in the passing state
* @return a future provided with list of services
* @see /v1/health/service/:service endpoint
*/
Future healthServiceNodes(String service, boolean passing);
/**
* Returns the nodes providing the service. This endpoint is very similar to the {@link ConsulClient#catalogServiceNodesWithOptions} endpoint;
* however, this endpoint automatically returns the status of the associated health check as well as any system level health checks.
*
* @param service the service name
* @param passing if true, filter results to only nodes with all checks in the passing state
* @param options options used to request services
* @return a future provided with list of services
* @see /v1/health/service/:service endpoint
*/
Future healthServiceNodesWithOptions(String service, boolean passing, ServiceQueryOptions options);
/**
* Returns the checks specific to the node provided on the path.
*
* @param node the node name or ID
* @param options options used to request node health checks
* @return a future provided with list of services
* @see /v1/health/node/:node endpoint
*/
Future healthNodesWithOptions(String node, CheckQueryOptions options);
/**
* Returns the services registered in a datacenter
*
* @return a future provided with list of services
* @see /v1/catalog/services endpoint
*/
Future catalogServices();
/**
* Returns the services registered in a datacenter
* This is blocking query unlike {@link ConsulClient#catalogServices()}
*
* @param options the blocking options
* @return a future provided with list of services
* @see /v1/catalog/services endpoint
*/
Future catalogServicesWithOptions(BlockingQueryOptions options);
/**
* Returns the node's registered services
*
* @param node node name
* @return a future provided with list of services
* @see /v1/catalog/node/:node endpoint
*/
Future catalogNodeServices(String node);
/**
* Returns the node's registered services
* This is blocking query unlike {@link ConsulClient#catalogNodeServices(String)}
*
* @param node node name
* @param options the blocking options
* @return a future provided with list of services
* @see /v1/catalog/node/:node endpoint
*/
Future catalogNodeServicesWithOptions(String node, BlockingQueryOptions options);
/**
* Returns list of services registered with the local agent.
*
* @return a future provided with list of services
* @see /v1/agent/services endpoint
*/
Future> localServices();
/**
* Return all the checks that are registered with the local agent.
*
* @return a future provided with list of checks
* @see /v1/agent/checks endpoint
*/
Future> localChecks();
/**
* Add a new check to the local agent. The agent is responsible for managing the status of the check
* and keeping the Catalog in sync.
*
* @param checkOptions options used to register new check
* @return a future notified when complete
* @see /v1/agent/check/register endpoint
*/
Future registerCheck(CheckOptions checkOptions);
/**
* Remove a check from the local agent. The agent will take care of deregistering the check from the Catalog.
*
* @param checkId the ID of check
* @return a future notified when complete
* @see /v1/agent/check/deregister/:check_id endpoint
*/
Future deregisterCheck(String checkId);
/**
* Set status of the check to "passing". Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @return a future notified when complete
* @see /v1/agent/check/pass/:check_id endpoint
* @see CheckStatus
*/
Future passCheck(String checkId);
/**
* Set status of the check to "passing". Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's {@code Output} field.
* @return a future notified when complete
* @see /v1/agent/check/pass/:check_id endpoint
* @see CheckStatus
*/
Future passCheckWithNote(String checkId, String note);
/**
* Set status of the check to "warning". Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @return a future notified when complete
* @see /v1/agent/check/warn/:check_id endpoint
* @see CheckStatus
*/
Future warnCheck(String checkId);
/**
* Set status of the check to "warning". Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's {@code Output} field.
* @return a future notified when complete
* @see /v1/agent/check/warn/:check_id endpoint
* @see CheckStatus
*/
Future warnCheckWithNote(String checkId, String note);
/**
* Set status of the check to "critical". Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @return a future notified when complete
* @see /v1/agent/check/fail/:check_id endpoint
* @see CheckStatus
*/
Future failCheck(String checkId);
/**
* Set status of the check to "critical". Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @param note specifies a human-readable message. This will be passed through to the check's {@code Output} field.
* @return a future notified when complete
* @see /v1/agent/check/fail/:check_id endpoint
* @see CheckStatus
*/
Future failCheckWithNote(String checkId, String note);
/**
* Set status of the check to given status. Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @param status new status of check
* @return a future notified when complete
* @see /v1/agent/check/update/:check_id endpoint
*/
Future updateCheck(String checkId, CheckStatus status);
/**
* Set status of the check to given status. Used with a check that is of the TTL type. The TTL clock will be reset.
*
* @param checkId the ID of check
* @param status new status of check
* @param note specifies a human-readable message. This will be passed through to the check's {@code Output} field.
* @return a future notified when complete
* @see /v1/agent/check/update/:check_id endpoint
*/
Future updateCheckWithNote(String checkId, CheckStatus status, String note);
/**
* Get the Raft leader for the datacenter in which the agent is running.
* It returns an address in format "10.1.10.12:8300
"
*
* @return a future provided with address of cluster leader
* @see /v1/status/leader endpoint
*/
Future leaderStatus();
/**
* Retrieves the Raft peers for the datacenter in which the the agent is running.
* It returns a list of addresses "10.1.10.12:8300
", "10.1.10.13:8300
"
*
* @return a future provided with list of peers
* @see /v1/status/peers endpoint
*/
Future> peersStatus();
/**
* Initialize a new session
*
* @return a future provided with ID of new session
* @see /v1/session/create endpoint
*/
Future createSession();
/**
* Initialize a new session
*
* @param options options used to create session
* @return a future provided with ID of new session
* @see /v1/session/create endpoint
*/
Future createSessionWithOptions(SessionOptions options);
/**
* Returns the requested session information
*
* @param id the ID of requested session
* @return a future provided with info of requested session
* @see /v1/session/info/:uuid endpoint
*/
Future infoSession(String id);
/**
* Returns the requested session information
* This is blocking query unlike {@link ConsulClient#infoSession(String)}
*
* @param id the ID of requested session
* @param options the blocking options
* @return a future provided with info of requested session
* @see /v1/session/info/:uuid endpoint
*/
Future infoSessionWithOptions(String id, BlockingQueryOptions options);
/**
* Renews the given session. This is used with sessions that have a TTL, and it extends the expiration by the TTL
*
* @param id the ID of session that should be renewed
* @return a future provided with info of renewed session
* @see /v1/session/renew/:uuid endpoint
*/
Future renewSession(String id);
/**
* Returns the active sessions
*
* @return a future provided with list of sessions
* @see /v1/session/list endpoint
*/
Future listSessions();
/**
* Returns the active sessions
* This is blocking query unlike {@link ConsulClient#listSessions()}
*
* @param options the blocking options
* @return a future provided with list of sessions
* @see /v1/session/list endpoint
*/
Future listSessionsWithOptions(BlockingQueryOptions options);
/**
* Returns the active sessions for a given node
*
* @param nodeId the ID of node
* @return a future provided with list of sessions
* @see /v1/session/node/:node endpoint
*/
Future listNodeSessions(String nodeId);
/**
* Returns the active sessions for a given node
* This is blocking query unlike {@link ConsulClient#listNodeSessions(String)}
*
* @param nodeId the ID of node
* @param options the blocking options
* @return a future provided with list of sessions
* @see /v1/session/node/:node endpoint
*/
Future listNodeSessionsWithOptions(String nodeId, BlockingQueryOptions options);
/**
* Destroys the given session
*
* @param id the ID of session
* @return a future notified when complete
* @see /v1/session/destroy/:uuid endpoint
*/
Future destroySession(String id);
/**
* @param definition definition of the prepare query
* @return a future provided with id of created prepare query
* @see /v1/query endpoint
*/
Future createPreparedQuery(PreparedQueryDefinition definition);
/**
* Returns an existing prepared query
*
* @param id the id of the query to read
* @return a future provided with definition of the prepare query
* @see /v1/query/:uuid endpoint
*/
Future getPreparedQuery(String id);
/**
* Returns a list of all prepared queries.
*
* @return a future provided with list of definitions of the all prepare queries
* @see /v1/query endpoint
*/
Future> getAllPreparedQueries();
/**
* @param definition definition of the prepare query
* @return a future notified when complete
* @see /v1/query/:uuid endpoint
*/
Future updatePreparedQuery(PreparedQueryDefinition definition);
/**
* Deletes an existing prepared query
*
* @param id the id of the query to delete
* @return a future notified when complete
* @see /v1/query/:uuid endpoint
*/
Future deletePreparedQuery(String id);
/**
* Executes an existing prepared query.
*
* @param query the ID of the query to execute. This can also be the name of an existing prepared query,
* or a name that matches a prefix name for a prepared query template.
* @return a future provided with response
* @see /v1/query/:uuid/execute endpoint
*/
Future executePreparedQuery(String query);
/**
* Executes an existing prepared query.
*
* @param query the ID of the query to execute. This can also be the name of an existing prepared query,
* or a name that matches a prefix name for a prepared query template.
* @param options the options used to execute prepared query
* @return a future provided with response
* @see /v1/query/:uuid/execute endpoint
*/
Future executePreparedQueryWithOptions(
String query,
PreparedQueryExecuteOptions options
);
/**
* Register node with external service
*
* @param nodeOptions the options of new node
* @param serviceOptions the options of new service
* @return a future provided with response
* @see /v1/catalog/register endpoint
*/
Future registerCatalogService(Node nodeOptions, ServiceOptions serviceOptions);
/**
* Deregister entities from the node or deregister the node itself.
*
* @param nodeId the ID of node
* @param serviceId the ID of the service to de-registered; if it is null, the node itself will be de-registered (as well as the entities that belongs to that node)
* @return a future notified when complete
* @see /v1/catalog/deregister endpoint
*/
Future deregisterCatalogService(String nodeId, String serviceId);
/**
* Close the client and release its resources
*/
void close();
}