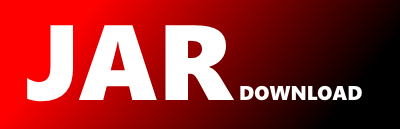
io.vertx.ext.consul.Watch Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2016 The original author or authors
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.ext.consul;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.codegen.annotations.VertxGen;
import io.vertx.core.Handler;
import io.vertx.core.Vertx;
import io.vertx.ext.consul.impl.WatchImpl;
/**
* Watches are a way of specifying a view of data (e.g. list of nodes, KV pairs, health checks)
* which is monitored for updates. When an update is detected, an {@code Handler} with {@code WatchResult} is invoked.
* All errors, except {@code java.util.concurrent.TimeoutException}, will be handled, with resubscribing with a progressive delay.
* All timeout errors will be ignored, with resubscribing without any delay.
* As an example, you could watch the status of health checks and notify when a check is critical.
*
* @author Ruslan Sennov
*/
@VertxGen
public interface Watch {
/**
* Creates {@code Watch} to monitoring a specific key in the KV store.
* The underlying Consul client will be created with default options.
* This maps to the /v1/kv/ API internally.
*
* @param key the key
* @param vertx the {@code Vertx} instance
* @return the {@code Watch} instance
*/
static Watch key(String key, Vertx vertx) {
return key(key, vertx, new ConsulClientOptions());
}
/**
* Creates {@code Watch} to monitoring a specific key in the KV store.
* This maps to the /v1/kv/ API internally.
*
* @param key the key
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch key(String key, Vertx vertx, ConsulClientOptions options) {
return new WatchImpl.Key(key, vertx, options);
}
/**
* Creates {@code Watch} to monitoring a prefix of keys in the KV store.
* The underlying Consul client will be created with default options.
* This maps to the /v1/kv/ API internally.
*
* @param keyPrefix the key
* @param vertx the {@code Vertx} instance
* @return the {@code Watch} instance
*/
static Watch keyPrefix(String keyPrefix, Vertx vertx) {
return keyPrefix(keyPrefix, vertx, new ConsulClientOptions());
}
/**
* Creates {@code Watch} to monitoring a prefix of keys in the KV store.
* This maps to the /v1/kv/ API internally.
*
* @param keyPrefix the key
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch keyPrefix(String keyPrefix, Vertx vertx, ConsulClientOptions options) {
return new WatchImpl.KeyPrefix(keyPrefix, vertx, options);
}
/**
* Creates {@code Watch} to monitoring the list of available services.
* The underlying Consul client will be created with default options.
* This maps to the /v1/catalog/services API internally.
*
* @param vertx the {@code Vertx} instance
* @return the {@code Watch} instance
*/
static Watch services(Vertx vertx) {
return services(vertx, new ConsulClientOptions());
}
/**
* Creates {@code Watch} to monitoring the list of available services.
* This maps to the /v1/catalog/services API internally.
*
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch services(Vertx vertx, ConsulClientOptions options) {
return new WatchImpl.Services(vertx, options);
}
/**
* Creates {@code Watch} to monitoring the nodes providing the service.
* Nodes will be sorted by distance from the consul agent.
* The underlying Consul client will be created with default options.
* This maps to the /v1/health/service/<service> API internally.
*
* @param service the service name
* @param vertx the {@code Vertx} instance
* @return the {@code Watch} instance
*/
static Watch service(String service, Vertx vertx) {
return service(service, vertx, new ConsulClientOptions());
}
/**
* Creates {@code Watch} to monitoring the nodes providing the service.
* Nodes will be sorted by distance from the consul agent.
* This maps to the /v1/health/service/<service> API internally.
*
* @param service the service name
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch service(String service, Vertx vertx, ConsulClientOptions options) {
return new WatchImpl.Service(service, vertx, options);
}
/**
* Creates {@code Watch} to monitoring the custom user events.
* The underlying Consul client will be created with default options.
* This maps to the /v1/event/list API internally.
*
* @param event the event name
* @param vertx the {@code Vertx} instance
* @return the {@code Watch} instance
*/
static Watch events(String event, Vertx vertx) {
return events(event, vertx, new ConsulClientOptions());
}
/**
* Creates {@code Watch} to monitoring the custom user events.
* This maps to the /v1/event/list API internally.
*
* @param event the event name
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch events(String event, Vertx vertx, ConsulClientOptions options) {
return new WatchImpl.Events(event, vertx, options);
}
/**
* Creates {@code Watch} to monitoring the list of available nodes.
* The underlying Consul client will be created with default options.
* This maps to the /v1/catalog/nodes API internally.
*
* @param vertx the {@code Vertx} instance
* @return the {@code Watch} instance
*/
static Watch nodes(Vertx vertx) {
return new WatchImpl.Nodes(vertx, new ConsulClientOptions());
}
/**
* Creates {@code Watch} to monitoring the list of available nodes.
* This maps to the /v1/catalog/nodes API internally.
*
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch nodes(Vertx vertx, ConsulClientOptions options) {
return new WatchImpl.Nodes(vertx, options);
}
/**
* Creates {@code Watch} to monitoring the health checks of the nodes.
* @param node node name or ID
* @param opt options like namespace, datacenter and filter
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch nodeHealthChecks(String node, CheckQueryOptions opt, Vertx vertx, ConsulClientOptions options){
return new WatchImpl.NodeHealthCheck(node, opt, vertx, options);
}
/**
* Creates {@code Watch} to monitoring the health checks of the nodes.
*
* @param service the service name
* @param checkQueryOptions options used to request checks
* @param vertx the {@code Vertx} instance
* @param options the options to create underlying Consul client
* @return the {@code Watch} instance
*/
static Watch serviceHealthChecks(String service, CheckQueryOptions checkQueryOptions, Vertx vertx, ConsulClientOptions options){
return new WatchImpl.ServiceHealthCheck(service, checkQueryOptions, vertx, options);
}
/**
* Set the result handler. As data is changed, the handler will be called with the result.
*
* @param handler the result handler
* @return reference to this, for fluency
*/
@Fluent
Watch setHandler(Handler> handler);
/**
* Start this {@code Watch}
*
* @return reference to this, for fluency
*/
@Fluent
Watch start();
/**
* Stop the watch and release its resources
*/
void stop();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy