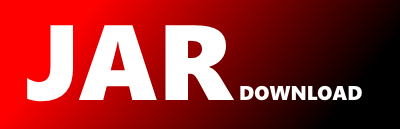
io.vertx.core.streams.ReadStream Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2013 The original author or authors
* ------------------------------------------------------
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.core.streams;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.codegen.annotations.Nullable;
import io.vertx.codegen.annotations.VertxGen;
import io.vertx.core.Handler;
/**
* Represents a stream of items that can be read from.
*
* Any class that implements this interface can be used by a {@link Pump} to pump data from it
* to a {@link WriteStream}.
*
* @author Tim Fox
*/
@VertxGen(concrete = false)
public interface ReadStream extends StreamBase {
/**
* Set an exception handler on the read stream.
*
* @param handler the exception handler
* @return a reference to this, so the API can be used fluently
*/
ReadStream exceptionHandler(Handler handler);
/**
* Set a data handler. As data is read, the handler will be called with the data.
*
* @return a reference to this, so the API can be used fluently
*/
@Fluent
ReadStream handler(@Nullable Handler handler);
/**
* Pause the {@code ReadSupport}. While it's paused, no data will be sent to the {@code dataHandler}
*
* @return a reference to this, so the API can be used fluently
*/
@Fluent
ReadStream pause();
/**
* Resume reading. If the {@code ReadSupport} has been paused, reading will recommence on it.
*
* @return a reference to this, so the API can be used fluently
*/
@Fluent
ReadStream resume();
/**
* Set an end handler. Once the stream has ended, and there is no more data to be read, this handler will be called.
*
* @return a reference to this, so the API can be used fluently
*/
@Fluent
ReadStream endHandler(@Nullable Handler endHandler);
}