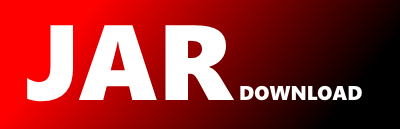
io.vertx.core.impl.ShadowContext Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2024 Contributors to the Eclipse Foundation
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the Apache License, Version 2.0
* which is available at https://www.apache.org/licenses/LICENSE-2.0.
*
* SPDX-License-Identifier: EPL-2.0 OR Apache-2.0
*/
package io.vertx.core.impl;
import io.netty.channel.EventLoop;
import io.vertx.codegen.annotations.Nullable;
import io.vertx.core.*;
import io.vertx.core.impl.deployment.DeploymentContext;
import io.vertx.core.internal.CloseFuture;
import io.vertx.core.internal.ContextInternal;
import io.vertx.core.internal.EventExecutor;
import io.vertx.core.internal.VertxInternal;
import io.vertx.core.json.JsonObject;
import io.vertx.core.spi.tracing.VertxTracer;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentMap;
/**
* A shadow context represents a context from a different Vert.x instance than the {@link #owner} instance.
*
* The primary use case for shadow context, is the integration of a Vert.x client within a Vert.x server with
* two distinct instances of Vert.x.
*
* When {@link Vertx#getOrCreateContext()} is invoked on an instance and the current thread is associated with a context
* of another instance, a shadow context is returned with an event-loop thread of the called vertx instance.
*
* When a thread is associated with a shadow context, {@link Vertx#getOrCreateContext()} returns the context tha
* was created by the corresponding instance.
*
* The following can be expected of a shadow context
*
* - {@link #threadingModel()} returns the {@link ThreadingModel#OTHER}
* - {@link #nettyEventLoop()} returns an event-loop of the {@link #owner()}
* - {@link #owner()} returns the Vertx instance that created it
* - {@link #executor()} returns the event executor of the shadowed context
* - {@link #workerPool()} returns the {@link #owner()} worker pool
*
*
*
* When a task is scheduled on a shadow context, that task is scheduled on the actual context event executor, therefore
* middleware running on the actual context interacts with this context resources, middleware running on the shadow context
* interacts with the shadow context resources.
*/
final class ShadowContext extends ContextBase {
final VertxInternal owner;
final ContextBase delegate;
private final EventLoopExecutor eventLoop;
private final TaskQueue orderedTasks;
public ShadowContext(VertxInternal owner, EventLoopExecutor eventLoop, ContextInternal delegate) {
super(((ContextBase)delegate).locals);
this.owner = owner;
this.eventLoop = eventLoop;
this.delegate = (ContextBase) delegate;
this.orderedTasks = new TaskQueue();
}
@Override
public EventExecutor eventLoop() {
return eventLoop;
}
@Override
public EventExecutor executor() {
return delegate.executor();
}
@Override
public EventLoop nettyEventLoop() {
return eventLoop.eventLoop;
}
@Override
public DeploymentContext deployment() {
return null;
}
@Override
public VertxInternal owner() {
return owner;
}
@Override
public void reportException(Throwable t) {
// Not sure of that
delegate.reportException(t);
}
@Override
public ConcurrentMap