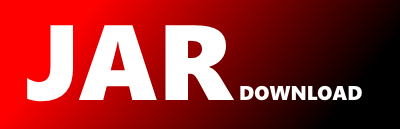
io.vertx.docgen.processor.impl.DocWriter Maven / Gradle / Ivy
The newest version!
package io.vertx.docgen.processor.impl;
import java.io.IOException;
import java.io.Writer;
import java.lang.reflect.UndeclaredThrowableException;
import java.util.ArrayList;
import java.util.List;
import java.util.function.Supplier;
/**
* @author Julien Viet
*/
public class DocWriter extends Writer {
private final StringBuilder delegate;
private final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy