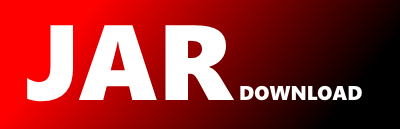
io.vertx.ext.dropwizard.impl.HttpRequestMetric Maven / Gradle / Ivy
package io.vertx.ext.dropwizard.impl;
import java.util.Iterator;
import java.util.LinkedList;
import java.util.List;
import io.vertx.core.http.HttpMethod;
/**
* @author Julien Viet
*/
class HttpRequestMetric {
final HttpMethod method;
final String uri;
long requestBegin;
// a string for a single route, a list of string for multiple
private Object routes;
// tracks length of resulting routes string
private int routesLength = 0;
HttpRequestMetric(HttpMethod method, String uri) {
this.method = method;
this.uri = uri;
requestBegin = System.nanoTime();
}
String getRoute() {
if (routes == null) {
return null;
}
if (routes instanceof String) {
return (String) routes;
}
StringBuilder concatenation = new StringBuilder(routesLength);
@SuppressWarnings("unchecked") Iterator iterator = ((List) routes).iterator();
concatenation.append(iterator.next());
while (iterator.hasNext()) {
concatenation.append('>').append(iterator.next());
}
routes = concatenation.toString();
return (String) routes;
}
// we try to minimize allocations as far as possible. see https://github.com/vert-x3/vertx-dropwizard-metrics/pull/101
void addRoute(String route) {
if (route == null) {
return;
}
routesLength += route.length();
if (routes == null) {
routes = route;
return;
}
++routesLength;
if (routes instanceof LinkedList) {
//noinspection unchecked
((LinkedList) routes).add(route);
return;
}
LinkedList multipleRoutes = new LinkedList<>();
multipleRoutes.add((String) routes);
multipleRoutes.add(route);
routes = multipleRoutes;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy