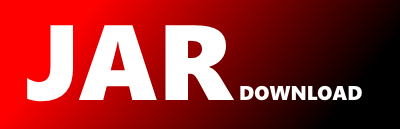
io.vertx.grpc.stub.ClientCalls Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2013 The original author or authors
* ------------------------------------------------------
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.grpc.stub;
import io.grpc.Status;
import io.grpc.stub.StreamObserver;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.Promise;
import io.vertx.core.internal.ContextInternal;
import io.vertx.core.streams.ReadStream;
import io.vertx.core.streams.WriteStream;
import java.util.function.BiConsumer;
import java.util.function.Function;
/**
* @author Rogelio Orts
* @author Eduard Català
*/
public final class ClientCalls {
private ClientCalls() {
}
public static Future oneToOne(ContextInternal ctx, I request, BiConsumer> delegate) {
Promise promise = ctx != null ? ctx.promise() : Promise.promise();
delegate.accept(request, toStreamObserver(promise));
return promise.future();
}
public static ReadStream oneToMany(ContextInternal ctx, I request, BiConsumer> delegate) {
return oneToMany(ctx, request, delegate, null, null, null);
}
public static ReadStream oneToMany(ContextInternal ctx, I request, BiConsumer> delegate, Handler handler, Handler endHandler, Handler exceptionHandler) {
StreamObserverReadStream response = new StreamObserverReadStream<>();
response.handler(handler).endHandler(endHandler).exceptionHandler(exceptionHandler);
delegate.accept(request, response);
return response;
}
public static Future manyToOne(ContextInternal ctx, Handler> requestHandler, Function, StreamObserver> delegate) {
Promise promise = ctx != null ? ctx.promise() : Promise.promise();
StreamObserver request = delegate.apply(toStreamObserver(promise));
requestHandler.handle(new GrpcWriteStream<>(request));
return promise.future();
}
public static ReadStream manyToMany(ContextInternal ctx, Handler> requestHandler, Function, StreamObserver> delegate) {
return manyToMany(ctx, requestHandler, delegate, null);
}
public static ReadStream manyToMany(ContextInternal ctx, Handler> requestHandler, Function, StreamObserver> delegate, Handler exceptionHandler) {
return manyToMany(ctx, requestHandler, delegate, null, null, null);
}
public static ReadStream manyToMany(ContextInternal ctx, Handler> requestHandler, Function, StreamObserver> delegate, Handler handler, Handler endHandler, Handler exceptionHandler) {
StreamObserverReadStream response = new StreamObserverReadStream<>();
response.handler(handler).endHandler(endHandler).exceptionHandler(exceptionHandler);
StreamObserver request = delegate.apply(response);
requestHandler.handle(new GrpcWriteStream<>(request));
return response;
}
private static StreamObserver toStreamObserver(Promise promise) {
return new StreamObserver() {
@Override
public void onNext(O tResponse) {
if (!promise.tryComplete(tResponse)) {
throw Status.INTERNAL
.withDescription("More than one responses received for unary or client-streaming call")
.asRuntimeException();
}
}
@Override
public void onError(Throwable throwable) {
promise.tryFail(throwable);
}
@Override
public void onCompleted() {
// Do nothing
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy