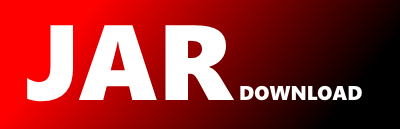
io.vertx.httpproxy.HttpProxy Maven / Gradle / Ivy
/*
* Copyright (c) 2011-2020 Contributors to the Eclipse Foundation
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License 2.0 which is available at
* http://www.eclipse.org/legal/epl-2.0, or the Apache License, Version 2.0
* which is available at https://www.apache.org/licenses/LICENSE-2.0.
*
* SPDX-License-Identifier: EPL-2.0 OR Apache-2.0
*/
package io.vertx.httpproxy;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.codegen.annotations.GenIgnore;
import io.vertx.codegen.annotations.VertxGen;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.http.HttpClient;
import io.vertx.core.http.HttpServerRequest;
import io.vertx.core.net.SocketAddress;
import io.vertx.httpproxy.impl.ReverseProxy;
import java.util.function.Function;
/**
* Handles the HTTP reverse proxy logic between the user agent and the origin.
*
* @author Julien Viet
*/
@VertxGen
public interface HttpProxy extends Handler {
/**
* Create a new {@code HttpProxy} instance.
*
* @param client the {@code HttpClient} that forwards outbound requests to the origin.
* @return a reference to this, so the API can be used fluently.
*/
static HttpProxy reverseProxy(HttpClient client) {
return new ReverseProxy(new ProxyOptions(), client);
}
/**
* Create a new {@code HttpProxy} instance.
*
* @param client the {@code HttpClient} that forwards outbound requests to the origin.
* @return a reference to this, so the API can be used fluently.
*/
static HttpProxy reverseProxy(ProxyOptions options, HttpClient client) {
return new ReverseProxy(options, client);
}
/**
* Set the {@code SocketAddress} of the origin.
*
* @param address the {@code SocketAddress} of the origin
* @return a reference to this, so the API can be used fluently
*/
@Fluent
default HttpProxy origin(SocketAddress address) {
return originSelector(req -> Future.succeededFuture(address));
}
/**
* Set the host name and port number of the origin.
*
* @param port the port number of the origin server
* @param host the host name of the origin server
* @return a reference to this, so the API can be used fluently
*/
@Fluent
default HttpProxy origin(int port, String host) {
return origin(SocketAddress.inetSocketAddress(port, host));
}
/**
* Set a selector that resolves the origin address based on the outbound request.
*
* @param selector the selector
* @return a reference to this, so the API can be used fluently
*/
@Fluent
HttpProxy originSelector(Function> selector);
/**
* Add an interceptor to the interceptor chain.
*
* @param interceptor
* @return a reference to this, so the API can be used fluently
*/
@Fluent
HttpProxy addInterceptor(ProxyInterceptor interceptor);
/**
* Handle the outbound {@code HttpServerRequest}.
*
* @param outboundRequest the outbound {@code HttpServerRequest}
*/
void handle(HttpServerRequest outboundRequest);
}