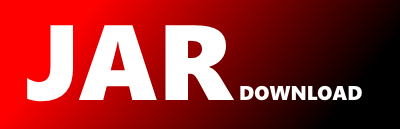
io.vertx.groovy.core.CompositeFuture.groovy Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.groovy.core;
import groovy.transform.CompileStatic
import io.vertx.lang.groovy.InternalHelper
import io.vertx.core.json.JsonObject
import java.util.List
import io.vertx.core.AsyncResult
import io.vertx.core.Handler
/**
* The composite future wraps a list of {@link io.vertx.groovy.core.Future futures}, it is useful when several futures
* needs to be coordinated.
*/
@CompileStatic
public class CompositeFuture extends Future {
private final def io.vertx.core.CompositeFuture delegate;
public CompositeFuture(Object delegate) {
super((io.vertx.core.CompositeFuture) delegate);
this.delegate = (io.vertx.core.CompositeFuture) delegate;
}
public Object getDelegate() {
return delegate;
}
/**
* Return a composite future, succeeded when all futures are succeeded, failed when any future is failed.
* @param f1 future
* @param f2 future
* @return the composite future
*/
public static CompositeFuture all(Future f1, Future f2) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.all((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#all} but with 3 futures.
* @param f1
* @param f2
* @param f3
* @return
*/
public static CompositeFuture all(Future f1, Future f2, Future f3) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.all((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#all} but with 4 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @return
*/
public static CompositeFuture all(Future f1, Future f2, Future f3, Future f4) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.all((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate(), (io.vertx.core.Future)f4.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#all} but with 5 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @return
*/
public static CompositeFuture all(Future f1, Future f2, Future f3, Future f4, Future f5) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.all((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate(), (io.vertx.core.Future)f4.getDelegate(), (io.vertx.core.Future)f5.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#all} but with 6 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @param f6
* @return
*/
public static CompositeFuture all(Future f1, Future f2, Future f3, Future f4, Future f5, Future f6) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.all((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate(), (io.vertx.core.Future)f4.getDelegate(), (io.vertx.core.Future)f5.getDelegate(), (io.vertx.core.Future)f6.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#all} but with a list of futures.
* @param futures
* @return
*/
public static CompositeFuture all(List futures) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.all((List)(futures.collect({underpants -> underpants.getDelegate()}))), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Return a composite future, succeeded when any futures is succeeded, failed when all futures are failed.
* @param f1 future
* @param f2 future
* @return the composite future
*/
public static CompositeFuture any(Future f1, Future f2) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.any((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#any} but with 3 futures.
* @param f1
* @param f2
* @param f3
* @return
*/
public static CompositeFuture any(Future f1, Future f2, Future f3) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.any((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#any} but with 4 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @return
*/
public static CompositeFuture any(Future f1, Future f2, Future f3, Future f4) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.any((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate(), (io.vertx.core.Future)f4.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#any} but with 5 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @return
*/
public static CompositeFuture any(Future f1, Future f2, Future f3, Future f4, Future f5) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.any((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate(), (io.vertx.core.Future)f4.getDelegate(), (io.vertx.core.Future)f5.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#any} but with 6 futures.
* @param f1
* @param f2
* @param f3
* @param f4
* @param f5
* @param f6
* @return
*/
public static CompositeFuture any(Future f1, Future f2, Future f3, Future f4, Future f5, Future f6) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.any((io.vertx.core.Future)f1.getDelegate(), (io.vertx.core.Future)f2.getDelegate(), (io.vertx.core.Future)f3.getDelegate(), (io.vertx.core.Future)f4.getDelegate(), (io.vertx.core.Future)f5.getDelegate(), (io.vertx.core.Future)f6.getDelegate()), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
/**
* Like {@link io.vertx.groovy.core.CompositeFuture#any} but with a list of futures.
* @param futures
* @return
*/
public static CompositeFuture any(List futures) {
def ret= InternalHelper.safeCreate(io.vertx.core.CompositeFuture.any((List)(futures.collect({underpants -> underpants.getDelegate()}))), io.vertx.groovy.core.CompositeFuture.class);
return ret;
}
public CompositeFuture setHandler(Handler> handler) {
( /* Work around for https://jira.codehaus.org/browse/GROOVY-6970 */ (io.vertx.core.CompositeFuture) this.delegate).setHandler(new Handler>() {
public void handle(AsyncResult event) {
AsyncResult f
if (event.succeeded()) {
f = InternalHelper.result(new CompositeFuture(event.result()))
} else {
f = InternalHelper.failure(event.cause())
}
handler.handle(f)
}
});
return this;
}
/**
* Returns a cause of a wrapped future
* @param index the wrapped future index
* @return
*/
public Throwable cause(int index) {
def ret = this.delegate.cause(index);
return ret;
}
/**
* Returns true if a wrapped future is succeeded
* @param index the wrapped future index
* @return
*/
public boolean succeeded(int index) {
def ret = this.delegate.succeeded(index);
return ret;
}
/**
* Returns true if a wrapped future is failed
* @param index the wrapped future index
* @return
*/
public boolean failed(int index) {
def ret = this.delegate.failed(index);
return ret;
}
/**
* Returns true if a wrapped future is completed
* @param index the wrapped future index
* @return
*/
public boolean isComplete(int index) {
def ret = this.delegate.isComplete(index);
return ret;
}
/**
* Returns the result of a wrapped future
* @param index the wrapped future index
* @return
*/
public T result(int index) {
// This cast is cleary flawed
def ret = (T) InternalHelper.wrapObject(this.delegate.result(index));
return ret;
}
/**
* @return the number of wrapped future
* @return
*/
public int size() {
def ret = this.delegate.size();
return ret;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy