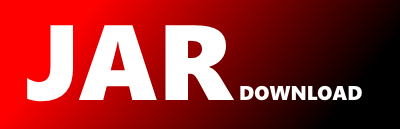
io.vertx.groovy.core.Vertx.groovy Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.groovy.core;
import groovy.transform.CompileStatic
import io.vertx.lang.groovy.InternalHelper
import io.vertx.core.json.JsonObject
import io.vertx.groovy.core.datagram.DatagramSocket
import io.vertx.groovy.core.http.HttpServer
import io.vertx.groovy.core.shareddata.SharedData
import io.vertx.groovy.core.eventbus.EventBus
import io.vertx.core.AsyncResult
import io.vertx.core.http.HttpClientOptions
import io.vertx.core.datagram.DatagramSocketOptions
import io.vertx.groovy.core.net.NetClient
import io.vertx.core.VertxOptions
import java.util.Set
import io.vertx.core.net.NetClientOptions
import io.vertx.groovy.core.dns.DnsClient
import io.vertx.core.net.NetServerOptions
import io.vertx.groovy.core.metrics.Measured
import io.vertx.groovy.core.net.NetServer
import io.vertx.core.DeploymentOptions
import io.vertx.groovy.core.file.FileSystem
import io.vertx.core.http.HttpServerOptions
import io.vertx.core.Handler
import io.vertx.groovy.core.http.HttpClient
/**
* The entry point into the Vert.x Core API.
*
* You use an instance of this class for functionality including:
*
* - Creating TCP clients and servers
* - Creating HTTP clients and servers
* - Creating DNS clients
* - Creating Datagram sockets
* - Setting and cancelling periodic and one-shot timers
* - Getting a reference to the event bus API
* - Getting a reference to the file system API
* - Getting a reference to the shared data API
* - Deploying and undeploying verticles
*
*
* Most functionality in Vert.x core is fairly low level.
*
* To create an instance of this class you can use the static factory methods: {@link io.vertx.groovy.core.Vertx#vertx},
* {@link io.vertx.groovy.core.Vertx#vertx} and {@link io.vertx.groovy.core.Vertx#clusteredVertx}.
*
* Please see the user manual for more detailed usage information.
*/
@CompileStatic
public class Vertx implements Measured {
private final def io.vertx.core.Vertx delegate;
public Vertx(Object delegate) {
this.delegate = (io.vertx.core.Vertx) delegate;
}
public Object getDelegate() {
return delegate;
}
/**
* Whether the metrics are enabled for this measured object
* @return true if the metrics are enabled
*/
public boolean isMetricsEnabled() {
def ret = ((io.vertx.core.metrics.Measured) this.delegate).isMetricsEnabled();
return ret;
}
/**
* Creates a non clustered instance using default options.
* @return the instance
*/
public static Vertx vertx() {
def ret= InternalHelper.safeCreate(io.vertx.core.Vertx.vertx(), io.vertx.groovy.core.Vertx.class);
return ret;
}
/**
* Creates a non clustered instance using the specified options
* @param options the options to use (see VertxOptions)
* @return the instance
*/
public static Vertx vertx(Map options) {
def ret= InternalHelper.safeCreate(io.vertx.core.Vertx.vertx(options != null ? new io.vertx.core.VertxOptions(new io.vertx.core.json.JsonObject(options)) : null), io.vertx.groovy.core.Vertx.class);
return ret;
}
/**
* Creates a clustered instance using the specified options.
*
* The instance is created asynchronously and the resultHandler is called with the result when it is ready.
* @param options the options to use (see VertxOptions)
* @param resultHandler the result handler that will receive the result
*/
public static void clusteredVertx(Map options = [:], Handler> resultHandler) {
io.vertx.core.Vertx.clusteredVertx(options != null ? new io.vertx.core.VertxOptions(new io.vertx.core.json.JsonObject(options)) : null, new Handler>() {
public void handle(AsyncResult event) {
AsyncResult f
if (event.succeeded()) {
f = InternalHelper.result(new Vertx(event.result()))
} else {
f = InternalHelper.failure(event.cause())
}
resultHandler.handle(f)
}
});
}
/**
* Gets the current context
* @return The current context or null if no current context
*/
public static Context currentContext() {
def ret= InternalHelper.safeCreate(io.vertx.core.Vertx.currentContext(), io.vertx.groovy.core.Context.class);
return ret;
}
/**
* Gets the current context, or creates one if there isn't one
* @return The current context (created if didn't exist)
*/
public Context getOrCreateContext() {
def ret= InternalHelper.safeCreate(this.delegate.getOrCreateContext(), io.vertx.groovy.core.Context.class);
return ret;
}
/**
* Create a TCP/SSL server using the specified options
* @param options the options to use (see NetServerOptions)
* @return the server
*/
public NetServer createNetServer(Map options) {
def ret= InternalHelper.safeCreate(this.delegate.createNetServer(options != null ? new io.vertx.core.net.NetServerOptions(new io.vertx.core.json.JsonObject(options)) : null), io.vertx.groovy.core.net.NetServer.class);
return ret;
}
/**
* Create a TCP/SSL server using default options
* @return the server
*/
public NetServer createNetServer() {
def ret= InternalHelper.safeCreate(this.delegate.createNetServer(), io.vertx.groovy.core.net.NetServer.class);
return ret;
}
/**
* Create a TCP/SSL client using the specified options
* @param options the options to use (see NetClientOptions)
* @return the client
*/
public NetClient createNetClient(Map options) {
def ret= InternalHelper.safeCreate(this.delegate.createNetClient(options != null ? new io.vertx.core.net.NetClientOptions(new io.vertx.core.json.JsonObject(options)) : null), io.vertx.groovy.core.net.NetClient.class);
return ret;
}
/**
* Create a TCP/SSL client using default options
* @return the client
*/
public NetClient createNetClient() {
def ret= InternalHelper.safeCreate(this.delegate.createNetClient(), io.vertx.groovy.core.net.NetClient.class);
return ret;
}
/**
* Create an HTTP/HTTPS server using the specified options
* @param options the options to use (see HttpServerOptions)
* @return the server
*/
public HttpServer createHttpServer(Map options) {
def ret= InternalHelper.safeCreate(this.delegate.createHttpServer(options != null ? new io.vertx.core.http.HttpServerOptions(new io.vertx.core.json.JsonObject(options)) : null), io.vertx.groovy.core.http.HttpServer.class);
return ret;
}
/**
* Create an HTTP/HTTPS server using default options
* @return the server
*/
public HttpServer createHttpServer() {
def ret= InternalHelper.safeCreate(this.delegate.createHttpServer(), io.vertx.groovy.core.http.HttpServer.class);
return ret;
}
/**
* Create a HTTP/HTTPS client using the specified options
* @param options the options to use (see HttpClientOptions)
* @return the client
*/
public HttpClient createHttpClient(Map options) {
def ret= InternalHelper.safeCreate(this.delegate.createHttpClient(options != null ? new io.vertx.core.http.HttpClientOptions(new io.vertx.core.json.JsonObject(options)) : null), io.vertx.groovy.core.http.HttpClient.class);
return ret;
}
/**
* Create a HTTP/HTTPS client using default options
* @return the client
*/
public HttpClient createHttpClient() {
def ret= InternalHelper.safeCreate(this.delegate.createHttpClient(), io.vertx.groovy.core.http.HttpClient.class);
return ret;
}
/**
* Create a datagram socket using the specified options
* @param options the options to use (see DatagramSocketOptions)
* @return the socket
*/
public DatagramSocket createDatagramSocket(Map options) {
def ret= InternalHelper.safeCreate(this.delegate.createDatagramSocket(options != null ? new io.vertx.core.datagram.DatagramSocketOptions(new io.vertx.core.json.JsonObject(options)) : null), io.vertx.groovy.core.datagram.DatagramSocket.class);
return ret;
}
/**
* Create a datagram socket using default options
* @return the socket
*/
public DatagramSocket createDatagramSocket() {
def ret= InternalHelper.safeCreate(this.delegate.createDatagramSocket(), io.vertx.groovy.core.datagram.DatagramSocket.class);
return ret;
}
/**
* Get the filesystem object. There is a single instance of FileSystem per Vertx instance.
* @return the filesystem object
*/
public FileSystem fileSystem() {
if (cached_0 != null) {
return cached_0;
}
def ret= InternalHelper.safeCreate(this.delegate.fileSystem(), io.vertx.groovy.core.file.FileSystem.class);
cached_0 = ret;
return ret;
}
/**
* Get the event bus object. There is a single instance of EventBus per Vertx instance.
* @return the event bus object
*/
public EventBus eventBus() {
if (cached_1 != null) {
return cached_1;
}
def ret= InternalHelper.safeCreate(this.delegate.eventBus(), io.vertx.groovy.core.eventbus.EventBus.class);
cached_1 = ret;
return ret;
}
/**
* Create a DNS client to connect to a DNS server at the specified host and port
* @param port the port
* @param host the host
* @return the DNS client
*/
public DnsClient createDnsClient(int port, String host) {
def ret= InternalHelper.safeCreate(this.delegate.createDnsClient(port, host), io.vertx.groovy.core.dns.DnsClient.class);
return ret;
}
/**
* Get the shared data object. There is a single instance of SharedData per Vertx instance.
* @return the shared data object
*/
public SharedData sharedData() {
if (cached_2 != null) {
return cached_2;
}
def ret= InternalHelper.safeCreate(this.delegate.sharedData(), io.vertx.groovy.core.shareddata.SharedData.class);
cached_2 = ret;
return ret;
}
/**
* Set a one-shot timer to fire after delay
milliseconds, at which point handler
will be called with
* the id of the timer.
* @param delay the delay in milliseconds, after which the timer will fire
* @param handler the handler that will be called with the timer ID when the timer fires
* @return the unique ID of the timer
*/
public long setTimer(long delay, Handler handler) {
def ret = this.delegate.setTimer(delay, handler);
return ret;
}
/**
* Returns a one-shot timer as a read stream. The timer will be fired after delay
milliseconds after
* the has been called.
* @param delay the delay in milliseconds, after which the timer will fire
* @return the timer stream
*/
public TimeoutStream timerStream(long delay) {
def ret= InternalHelper.safeCreate(this.delegate.timerStream(delay), io.vertx.groovy.core.TimeoutStream.class);
return ret;
}
/**
* Set a periodic timer to fire every delay
milliseconds, at which point handler
will be called with
* the id of the timer.
* @param delay the delay in milliseconds, after which the timer will fire
* @param handler the handler that will be called with the timer ID when the timer fires
* @return the unique ID of the timer
*/
public long setPeriodic(long delay, Handler handler) {
def ret = this.delegate.setPeriodic(delay, handler);
return ret;
}
/**
* Returns a periodic timer as a read stream. The timer will be fired every delay
milliseconds after
* the has been called.
* @param delay the delay in milliseconds, after which the timer will fire
* @return the periodic stream
*/
public TimeoutStream periodicStream(long delay) {
def ret= InternalHelper.safeCreate(this.delegate.periodicStream(delay), io.vertx.groovy.core.TimeoutStream.class);
return ret;
}
/**
* Cancels the timer with the specified id
.
* @param id The id of the timer to cancel
* @return true if the timer was successfully cancelled, or false if the timer does not exist.
*/
public boolean cancelTimer(long id) {
def ret = this.delegate.cancelTimer(id);
return ret;
}
/**
* Puts the handler on the event queue for the current context so it will be run asynchronously ASAP after all
* preceeding events have been handled.
* @param action - a handler representing the action to execute
*/
public void runOnContext(Handler action) {
this.delegate.runOnContext(action);
}
/**
* Stop the the Vertx instance and release any resources held by it.
*
* The instance cannot be used after it has been closed.
*
* The actual close is asynchronous and may not complete until after the call has returned.
*/
public void close() {
this.delegate.close();
}
/**
* Like {@link io.vertx.groovy.core.Vertx#close} but the completionHandler will be called when the close is complete
* @param completionHandler The handler will be notified when the close is complete.
*/
public void close(Handler> completionHandler) {
this.delegate.close(completionHandler);
}
/**
* Deploy a verticle instance given a name.
*
* Given the name, Vert.x selects a instance to use to instantiate the verticle.
*
* For the rules on how factories are selected please consult the user manual.
* @param name the name.
*/
public void deployVerticle(String name) {
this.delegate.deployVerticle(name);
}
/**
* Like {@link io.vertx.groovy.core.Vertx#deployVerticle} but the completionHandler will be notified when the deployment is complete.
*
* If the deployment is successful the result will contain a String representing the unique deployment ID of the
* deployment.
*
* This deployment ID can subsequently be used to undeploy the verticle.
* @param name The identifier
* @param completionHandler a handler which will be notified when the deployment is complete
*/
public void deployVerticle(String name, Handler> completionHandler) {
this.delegate.deployVerticle(name, completionHandler);
}
/**
* Like {@link io.vertx.groovy.core.Vertx#deployVerticle} but DeploymentOptions are provided to configure the
* deployment.
* @param name the name
* @param options the deployment options. (see DeploymentOptions)
*/
public void deployVerticle(String name, Map options) {
this.delegate.deployVerticle(name, options != null ? new io.vertx.core.DeploymentOptions(new io.vertx.core.json.JsonObject(options)) : null);
}
/**
* Like {@link io.vertx.groovy.core.Vertx#deployVerticle} but DeploymentOptions are provided to configure the
* deployment.
* @param name the name
* @param options the deployment options. (see DeploymentOptions)
* @param completionHandler a handler which will be notified when the deployment is complete
*/
public void deployVerticle(String name, Map options, Handler> completionHandler) {
this.delegate.deployVerticle(name, options != null ? new io.vertx.core.DeploymentOptions(new io.vertx.core.json.JsonObject(options)) : null, completionHandler);
}
/**
* Undeploy a verticle deployment.
*
* The actual undeployment happens asynchronously and may not complete until after the method has returned.
* @param deploymentID the deployment ID
*/
public void undeploy(String deploymentID) {
this.delegate.undeploy(deploymentID);
}
/**
* Like {@link io.vertx.groovy.core.Vertx #undeploy(String)} but the completionHandler will be notified when the undeployment is complete.
* @param deploymentID the deployment ID
* @param completionHandler a handler which will be notified when the undeployment is complete
*/
public void undeploy(String deploymentID, Handler> completionHandler) {
this.delegate.undeploy(deploymentID, completionHandler);
}
/**
* Return a Set of deployment IDs for the currently deployed deploymentIDs.
* @return Set of deployment IDs
*/
public Set deploymentIDs() {
def ret = this.delegate.deploymentIDs();
return ret;
}
/**
* Is this Vert.x instance clustered?
* @return true if clustered
*/
public boolean isClustered() {
def ret = this.delegate.isClustered();
return ret;
}
/**
* Safely execute some blocking code.
*
* Executes the blocking code in the handler blockingCodeHandler
using a thread from the worker pool.
*
* When the code is complete the handler resultHandler
will be called with the result on the original context
* (e.g. on the original event loop of the caller).
*
* A Future
instance is passed into blockingCodeHandler
. When the blocking code successfully completes,
* the handler should call the {@link io.vertx.groovy.core.Future#complete} or {@link io.vertx.groovy.core.Future#complete} method, or the {@link io.vertx.groovy.core.Future#fail}
* method if it failed.
*
* In the blockingCodeHandler
the current context remains the original context and therefore any task
* scheduled in the blockingCodeHandler
will be executed on the this context and not on the worker thread.
* @param blockingCodeHandler handler representing the blocking code to run
* @param ordered if true then if executeBlocking is called several times on the same context, the executions for that context will be executed serially, not in parallel. if false then they will be no ordering guarantees
* @param resultHandler handler that will be called when the blocking code is complete
*/
public void executeBlocking(Handler> blockingCodeHandler, boolean ordered, Handler> resultHandler) {
this.delegate.executeBlocking(new Handler>() {
public void handle(io.vertx.core.Future event) {
blockingCodeHandler.handle(new io.vertx.groovy.core.Future(event));
}
}, ordered, new Handler>() {
public void handle(AsyncResult