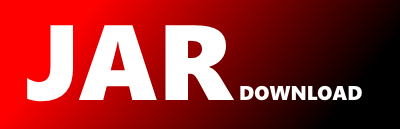
io.vertx.groovy.core.eventbus.Message.groovy Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.groovy.core.eventbus;
import groovy.transform.CompileStatic
import io.vertx.lang.groovy.InternalHelper
import io.vertx.core.json.JsonObject
import io.vertx.core.eventbus.DeliveryOptions
import io.vertx.groovy.core.MultiMap
import io.vertx.core.AsyncResult
import io.vertx.core.Handler
/**
* Represents a message that is received from the event bus in a handler.
*
* Messages have a {@link io.vertx.groovy.core.eventbus.Message#body}, which can be null, and also {@link io.vertx.groovy.core.eventbus.Message#headers}, which can be empty.
*
* If the message was sent specifying a reply handler it will also have a {@link io.vertx.groovy.core.eventbus.Message#replyAddress}. In that case the message
* can be replied to using that reply address, or, more simply by just using {@link io.vertx.groovy.core.eventbus.Message#reply}.
*
* If you want to notify the sender that processing failed, then {@link io.vertx.groovy.core.eventbus.Message#fail} can be called.
*/
@CompileStatic
public class Message {
private final def io.vertx.core.eventbus.Message delegate;
public Message(Object delegate) {
this.delegate = (io.vertx.core.eventbus.Message) delegate;
}
public Object getDelegate() {
return delegate;
}
/**
* The address the message was sent to
* @return
*/
public String address() {
def ret = this.delegate.address();
return ret;
}
/**
* Multi-map of message headers. Can be empty
* @return the headers
*/
public MultiMap headers() {
def ret= InternalHelper.safeCreate(this.delegate.headers(), io.vertx.groovy.core.MultiMap.class);
return ret;
}
/**
* The body of the message. Can be null.
* @return the body, or null.
*/
public T body() {
if (cached_0 != null) {
return cached_0;
}
// This cast is cleary flawed
def ret = (T) InternalHelper.wrapObject(this.delegate.body());
cached_0 = ret;
return ret;
}
/**
* The reply address. Can be null.
* @return the reply address, or null, if message was sent without a reply handler.
*/
public String replyAddress() {
def ret = this.delegate.replyAddress();
return ret;
}
/**
* Reply to this message.
*
* If the message was sent specifying a reply handler, that handler will be
* called when it has received a reply. If the message wasn't sent specifying a receipt handler
* this method does nothing.
* @param message the message to reply with.
*/
public void reply(Object message) {
this.delegate.reply(InternalHelper.unwrapObject(message));
}
/**
* The same as reply(R message)
but you can specify handler for the reply - i.e.
* to receive the reply to the reply.
* @param message the message to reply with.
* @param replyHandler the reply handler for the reply.
*/
public void reply(Object message, Handler>> replyHandler) {
this.delegate.reply(InternalHelper.unwrapObject(message), new Handler>>() {
public void handle(AsyncResult> event) {
AsyncResult> f
if (event.succeeded()) {
f = InternalHelper.>result(new Message